Non classé Recrutement :
Ready to begin dating a sugar momma for females momma? begin now
http://marionjensen.com/2019/05 Dating a sugar momma is a great experience if you should be prepared for the challenges that come with this type of relationship.here are five ideas to help you to get started:
1.be willing to put in a large amount of effort.a sugar momma will probably be very demanding, and you will need to be ready to put in a lot of work to keep the woman happy.if you’re not willing to devote the time and effort, then you’ll definitely be rejected by her.2.be prepared to be financially influenced by the sugar momma.a sugar momma is going to be very good with her cash, and you’ll likely need to be economically determined by the woman in order to maintain a relationship with her.this means that you will have to anticipate to provide the girl a lot of cash, and you should not be expectant of the woman to produce any economic support in exchange.3.be prepared to be emotionally influenced by the sugar momma.a sugar momma is likely to be really emotionally dependent on you, and you will probably need to be emotionally dependent on her to maintain a relationship with her.this implies that you need to anticipate to give the lady a lot of emotional help, and you should not really expect the woman to deliver any emotional support inturn.4.be prepared to tolerate a lot of drama.a sugar momma may very well be really drama-prone, and you may likely need certainly to tolerate a lot of drama to be able to maintain a relationship with her.this implies that you will have to expect you’ll handle a lot of emotional drama, and you should not be expectant of the woman to cope with any emotional drama in return.5.be prepared to tolerate a lot of criticism.a sugar momma may very well be very critical, and you’ll probably have to put up with a lot of critique in order to maintain a relationship with her.this means you will have to be prepared to cope with a lot of negative critique, and you should not be expectant of the woman to manage any negative criticism inturn.
Find your perfect sugar momma and enjoy dating again
Finding a sugar momma can be a terrific way to improve your dating life. they’re ladies who are prepared to offer financial and emotional help with their sons or daughters. they could be outstanding assist in finding somebody, as well as are a good source of advice. there are a variety of sugar momma dating sites available. you will find sites which are focused on helping individuals find sugar mommas, or perhaps you can find sites which are specialized in helping individuals find dates. whichever website you decide on, make sure you use the correct keywords. keywords for sugar momma dating sites
financial help, dating, sugar mommas, relationships
What are the great things about dating a sugar momma?
There are many advantageous assets to dating a sugar momma.sugar mommas tend to be experienced women who have discovered just how to take care of themselves.they are confident and know how to handle relationships.they tend to be successful and have big money.they tend to be good audience as they are able to provide their partners the eye they need.they are often able to provide their lovers with financial security and good life style.they tend to be capable provide their lovers with possibilities which they would not be able to find on their own.they in many cases are able to offer their partners with a feeling of security.
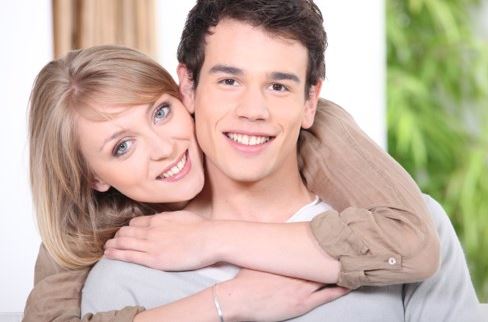
What is a lesbian sugar momma?
what’s a lesbian sugar momma dating site? a lesbian sugar momma dating site is a website designed for lesbian ladies to get other lesbian sugar mommas. sugar mommas are ladies who are financially supportive of their children or grandchildren. they are usually mothers who possess big money and therefore are capable provide their children or grandchildren economic help. sugar mommas can be an excellent source of support for their children or grandchildren. they can give them financial assistance, help these with their funds, and help them learn about money. they are able to give them emotional help and support them to build up relationships with other females. websites like this allow lesbian women discover other women who share their same passions and who can be a great way to obtain help. web sites such as this can be an effective way for lesbian ladies to get other lesbian sugar mommas who is able to help them to produce relationships and support them emotionally. web sites like this could be an effective way for lesbian ladies discover other lesbian sugar mommas who are able to give them monetary support.
Get started with sugar mommas dating now
If you’re looking for a method to begin with sugar mommas dating, you are in fortune! here are a few suggestions to allow you to get started:
1. begin by finding several sugar mommas that are thinking about dating. this can be carried out by making use of on the web dating web sites or by fulfilling up in person. 2. ensure that you be respectful and courteous to your sugar mommas. this can demonstrate to them that you are enthusiastic about dating them and can make the procedure easier. 3. expect you’ll fork out a lot of the time in the dating front. sugar mommas are busy people and additionally they want to be sure that their dates are worth their time. 4. be honest and upfront with your sugar mommas. this can help to build a very good relationship and ensure that the two of you are content with the dating arrangement.

References:
https://apptopia.com/google-play/app/com.sugarbabydatingapps.club.app/intelligence