Non classé Recrutement :
Explore the options of asian man black woman dating
where can i buy Ivermectin Asian man black woman dating is an interest that is often taboo, but shouldn’t be. there are many benefits to dating an asian man, and black women can be no exclusion. here are five explanations why black females should date asian men: 1. cultural connections asian males tend to be the first to ever mention social connections. they have been well-educated while having a wide range of passions, making them great conversationalists. they are acquainted many different cultures, which can make for interesting dates. 2. household connections numerous asian guys join interracialsexualdating.com\’s referral program for rewards have strong household connections. this is often outstanding asset in terms of dating. they are generally capable provide help and guidance whenever required. 3. job aspirations asian guys frequently have strong profession goals. they are usually inspired and driven, which can make for a exciting relationship. 4. spontaneity asian men frequently have a good sense of humor. they can be fun and entertaining, which will make for a good date. 5. compatibility asian males and black women frequently have outstanding compatibility. they share many typical passions and values, which will make for a fantastic relationship.
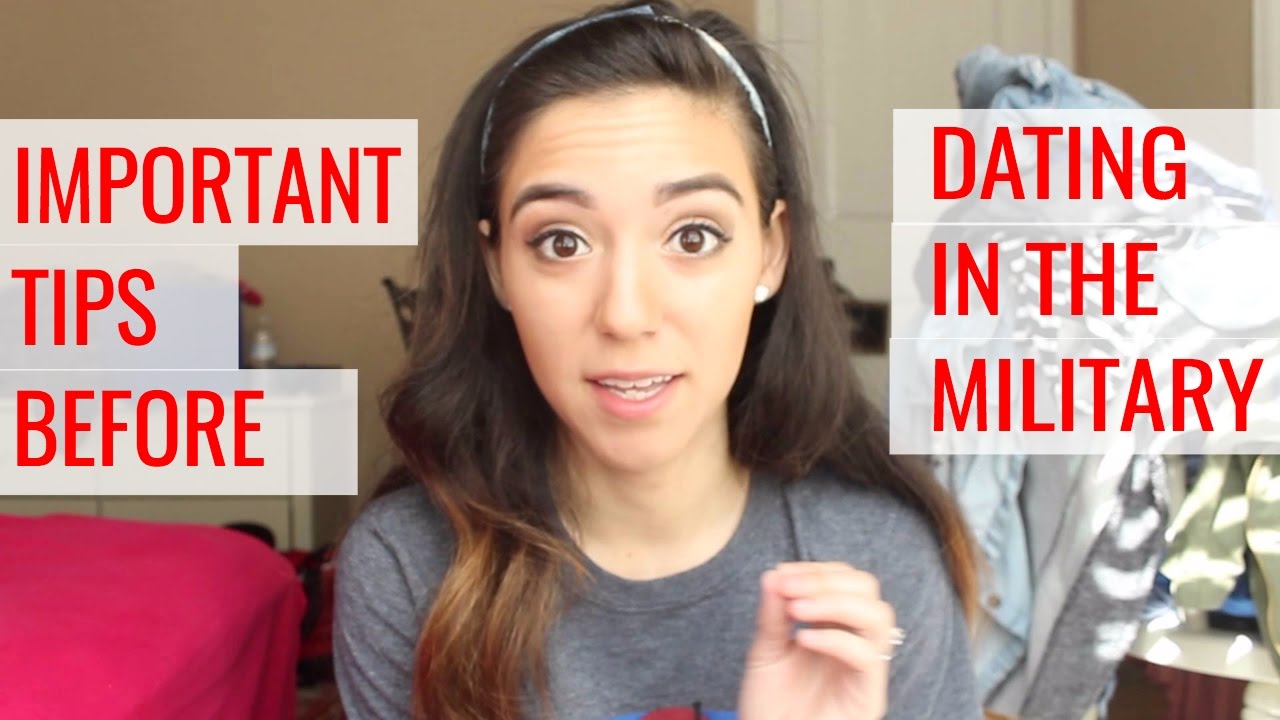
Discover the advantages of asian man black woman dating
Asian man black woman dating could be an extremely useful relationship for both parties involved. asian man black woman dating can help to broaden the horizons of both events and certainly will help to improve the partnership. asian man black woman dating can help to improve interaction and can help build a stronger relationship. asian man black woman dating can help increase the relationship by increasing the understanding and appreciation for every single other’s cultures.
Discover love and friendship with asian man black woman dating
Asian man black woman dating is a relationship that may be very fulfilling. it can be a way to find love and friendship, and it will be ways to build a strong relationship. there are a few facts to consider when dating an asian man black woman. first, you should understand the cultural differences when considering asian men and women. asian males are typically more reserved than women, in addition they may not show their feelings as easily. it is critical to be patient and understanding whenever dating an asian man. 2nd, it is vital to be aware of different dating customs in asia. for instance, numerous asian cultures consider it rude to kiss in the first date. it’s important to know about these traditions and follow them if you’re dating an asian man. finally, you will need to be familiar with the various expectations that asian people have in terms of relationships. asian males are generally more traditional than ladies, and so they may choose to get hitched and possess young ones immediately after they begin dating.
Unlock the potential of asian man black woman dating with our expert advice
Asian man black woman dating – unlock the potential of asian man black woman dating with this professional advice
dating asian man black woman are a fantastic experience for both parties involved. however, it are hard to know very well what to accomplish to increase the possibility of success. in this article, we’ll provide solid advice on how to unlock the potential of asian man black woman dating. the first step is understand the differences between the two countries. asian men are typically more conservative than united states males, and black women can be typically more separate than white women. these differences can lead to conflicts if not understood and respected. it is also crucial that you understand the distinctions in dating behavior. the important thing to a fruitful asian man black woman dating is to comprehend and respect the distinctions between the two countries and dating behavior. by doing so, it is possible to maximize the prospect of a fruitful relationship.
Secrets to finding love with asian man black woman dating
Asian man and black woman dating could be a very worthwhile experience if you know the best secrets. listed below are five tips to assist you in finding love with an asian man black woman dating:
1. start by understanding both’s countries. one of the first things you need to do would be to realize each other’s countries. this means understanding the values and opinions that each and every of you possess. this can help you to connect on a deeper level and make certain that your relationship is founded on something more than simply shallow factors. 2. be truthful and open together. perhaps one of the most considerations you need to do is be honest and available with one another. this implies being prepared to share your thoughts and feelings, in spite of how difficult they might be. this can help to build trust and self-confidence between you and your partner. 3. do not be afraid to test brand new things. one of the better reasons for having dating an asian man black woman dating is the fact that you can always take to brand new things. this implies being ready to simply take risks and explore new territory. this will help add spice to your relationship while making it more exciting. 4. do not be afraid expressing your feelings. what this means is being ready to communicate openly and truthfully. this may help to build a good relationship which based on trust and mutual respect. 5. avoid being afraid to compromise. this means being willing to make adjustments and concessions to be able to maintain a powerful relationship. this may help to make certain that both of you are happy and satisfied.
Exploring the many benefits of dating an asian man and a black woman
Dating an asian man and a black woman are a terrific way to explore new countries and meet interesting people. those two groups tend to be misinterpreted, nevertheless they can have a lot in accordance. here are a few associated with advantages of dating an asian man and a black woman:
1. they’ve a distinctive perspective
asian guys and black ladies frequently have a unique perspective in the globe. this is often a powerful way to learn more about various countries. 2. they’re proficient at communicating
asian guys and black women are usually good at interacting. this could make relationships easier simply because theyare able to communicate efficiently. 3. they truly are devoted
asian males and black women are frequently devoted. this could easily make relationships stay longer since they’re faithful towards people they’re with. 4. this can make relationships more versatile simply because theyare able to adjust to various circumstances. 5.
Unlocking the potential of asian man black woman dating
Asian man black woman dating is a relationship that the prospective to be very effective. people don’t realize the many benefits of dating an asian man black woman. listed here are five explanations why dating an asian man black woman may be beneficial. 1. they will have an original viewpoint. asian man black woman dating offers an original viewpoint that’s not usually present other relationships. the reason being asian man black woman dating offers an opportunity to explore various cultures and philosophy. this is an invaluable chance of both parties. 2. they are smart. asian man black woman dating supplies the opportunity to date somebody who is intelligent and capable. many asian man black woman dating couples have plenty in keeping and are usually able to build a powerful relationship based on provided values and interests. 3. they truly are dedicated. asian man black woman dating couples are faithful together. it is because they realize the importance of loyalty and dedication. also able to trust both, that will be a valuable asset in a relationship. 4. they’ve been understanding. it is because they can start to see the globe from an alternate perspective. also in a position to understand the wants and wants of the other person. 5. these are typically passionate. this is because they can connect on a deep degree. they are in a position to share their emotions and emotions with every other.