Non classé Recrutement :
Uncover the most effective mega hookup site spots in st paul
Şarkîkaraağaç St paul is an excellent place to find a hookup. with so many pubs and clubs, it is difficult to perhaps not find someplace for down. listed below are five of the best spots in st paul to find a hookup. 1. the landing
the landing is a good spot to find a hookup as it has some power. it’s a well known spot for individuals to satisfy and socialize, so that you’re likely to find anyone to connect with. plus, the products are cheap while the atmosphere is enjoyable. 2. the cabooze
the cabooze is another great destination to find a hookup. 3. the myth
the misconception is a good place to find a hookup since it’s a popular club with many people. it’s also a great spot to find a night out together, because it has plenty of restaurants and pubs nearby. 4. 5.
Hookup with older sluts – enjoy an unforgettable experience
There’s something about older females that makes them extremely sexy. they know what they desire plus they aren’t afraid to pursue it. they truly are additionally experienced and understand how to celebrate. if you’re looking for an unforgettable experience, then chances are you should attach with an older slut. below are a few tips about how to do it:
1. be upfront regarding the intentions. if you should be simply in search of a one-time hookup, be upfront about this from the beginning. older women can be always being sexualized and they are not going to be offended if you should be upfront about what you are considering. 2. avoid being afraid become your self. older women are used to being around confident males whom understand what they desire. if you’re shy or introverted, that is ok. just be yourself plus don’t try to be some body you are not. 3. don’t be afraid to take things sluggish. they’re not going to rush you and they’re not likely to expect anything away from you that you are maybe not prepared to give. 4. likely be operational to brand new experiences. older women can be often more available to new experiences than more youthful women. if you should be open to trying one thing new, then older women are probably be available to it besides. 5. if you’re comfortable being sexual, then you definitely’re apt to be comfortable with older ladies too. 6. be respectful. if you are respectful of them, chances are they’re likely to be respectful of you. 7. 8. do not be afraid to inquire of for what you need. if you should be confident and know what you need, then chances are you’re apt to be in a position to have it from an older woman. 9. don’t be afraid to be available to brand new relationships.
What to look out for in a new hookup site
When it comes to finding a new hookup site, there are some items to consider. above all, it is critical to find a website that’s user-friendly and easy to navigate. in addition, you need to find a site that a sizable individual base and it is well liked among the community. finally, it’s important to find a website who has a strong reputation and it is reliable.

Get ready for a wild trip: get hooked up with older sluts
If you are considering a wild and crazy night, you should positively start thinking about setting up with older sluts. not just will they be more knowledgeable in the wide world of love, nonetheless they’re additionally prone to be up for some serious enjoyable. and, contrary to popular belief, there are numerous older women who are seeking a great time, too. so, if you should be ready for a night of pure pleasure, make sure you browse the older sluts in your area. you will not be sorry!
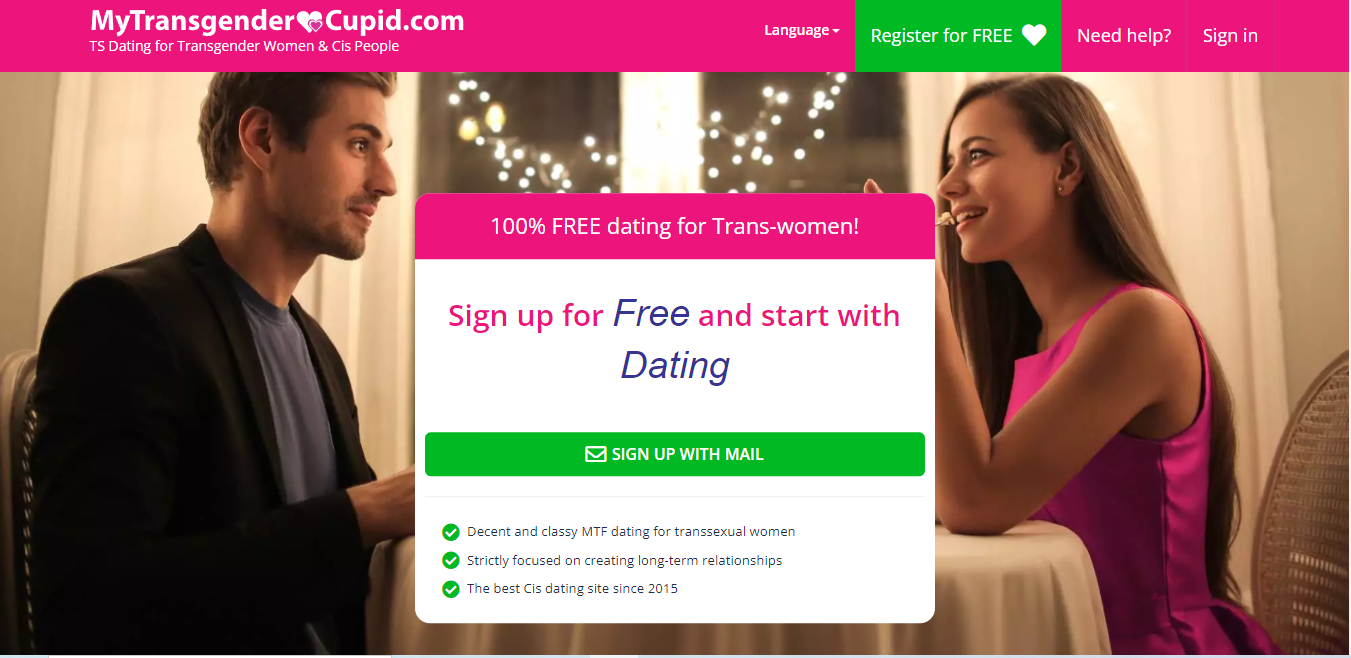
Make your fantasies be realized: hookup with older sluts today
Hookup with older sluts is a well known fantasy for many men. whether you are looking for a one-time encounter or something more serious, there’s a slut online that is simply awaiting you. if you should be worried about how old you are or whether you’re prepared for a relationship, you shouldn’t be. older sluts tend to be more than thrilled to have a casual hookup with some guy who is interested. in fact, many are searching for something more than just an instant fuck. if you’re ready to make your fantasies become a reality, here are a few ideas to begin:
1. be upfront about your motives. older sluts are well alert to what they’re getting on their own into, so be upfront in what you are considering. if you are just in search of a one-time hookup, be upfront about that. if you should be enthusiastic about something more severe, be upfront about this, too. 2. be respectful. older sluts are skilled and understand how to handle by themselves. never treat them like children or try to take advantage of them. respect their boundaries plus don’t push too hard. 3. be honest. older sluts are used to being honest with males, so be truthful with them, too. if you should be perhaps not ready for a relationship, be upfront about this. 4. have patience. older sluts are used to being choosy, so have patience and do not expect them become available on a regular basis. if you are maybe not ready to wait, that’s okay, too. there are plenty of other opportunities out there. 5. be respectful of their own time. older sluts are busy people and additionally they do not have time for games. if you are willing to make your fantasies come true, connect with older sluts today.
References:
https://www.goodreads.com/book/show/31398386-mystery-suspense