Non classé Recrutement :
Unlock the number of choices of lesbian asian women dating today
Amod Lesbian asian women dating is an interest that is often taboo, but shouldn’t be. there are numerous possibilities for lesbian asian women to date and find love. listed here are five ideas to help unlock the possibilities of lesbian asian women dating today. 1. look for social events and gatherings especially for lesbian asian women. this will allow you to meet other lesbian asian women and kind relationships. 2. join online dating web sites especially for lesbian asian women. 3. make the most of dating apps that cater specifically to lesbian asian women. 4. venture out on dates along with other lesbian asian women. 5. likely be operational and honest about your feelings. this can permit you to form relationships along with other lesbian asian women being based on https://lesbianchat.app/asian-lesbian-dating/ mutual respect and trust.
Ready discover love? begin your lesbian asian dating adventure today
If you are considering a new dating experience, or perhaps wish to explore the world of dating, lesbian asian women dating is the perfect way to go.with numerous amazing women around, it’s difficult to choose whom to date.but don’t worry, we are right here to help.lesbian asian women dating is a great way to satisfy brand new individuals, and it can be a great and exciting experience.plus, it’s a powerful way to find love.if you are ready to start your lesbian asian dating adventure, below are a few ideas to help you to get started.first, always have a good profile.your profile must certanly be packed with information about you, plus it should be simple to find.make certain to add your name, age, and a photo.next, ensure you’re ready to date.if you aren’t ready to date yet, that’s ok.but make sure you’re prepared to date someone who is.if you aren’t prepared to date someone who is lesbian asian, that is additionally okay.but make sure you’re willing to date an individual who is available to dating a person who is lesbian asian.finally, ensure you’re willing to date somebody who is lesbian asian.if you aren’t willing to date somebody who is lesbian asian, that is also ok.but ensure you’re prepared to date someone who is open to dating an individual who is lesbian asian.ready to locate love?start your lesbian asian dating adventure today.
Get prepared to meet the girl of one’s dreams
Are you seeking a lady who shares your exact same passions and passions? if that’s the case, maybe you are thinking about dating an asian lesbian. asian lesbians are a distinctive band of ladies who share a common desire for ladies. this could easily lead to an interesting and exciting dating experience. below are a few what to bear in mind if you should be enthusiastic about dating an asian lesbian:
1. asian lesbians are generally separate and self-sufficient. this can make them tough to get acquainted with, however it can also make sure they are interesting and exciting people. 2. asian lesbians are usually open-minded and accepting of other people. this could make sure they are outstanding match for an individual who normally open-minded and accepting. 3. asian lesbians are generally passionate about their passions. 4. asian lesbians are usually dedicated and supportive friends. if you’re interested in dating an asian lesbian, be equipped for an appealing and exciting dating experience. be sure to keep these guidelines at heart whenever dating an asian lesbian.
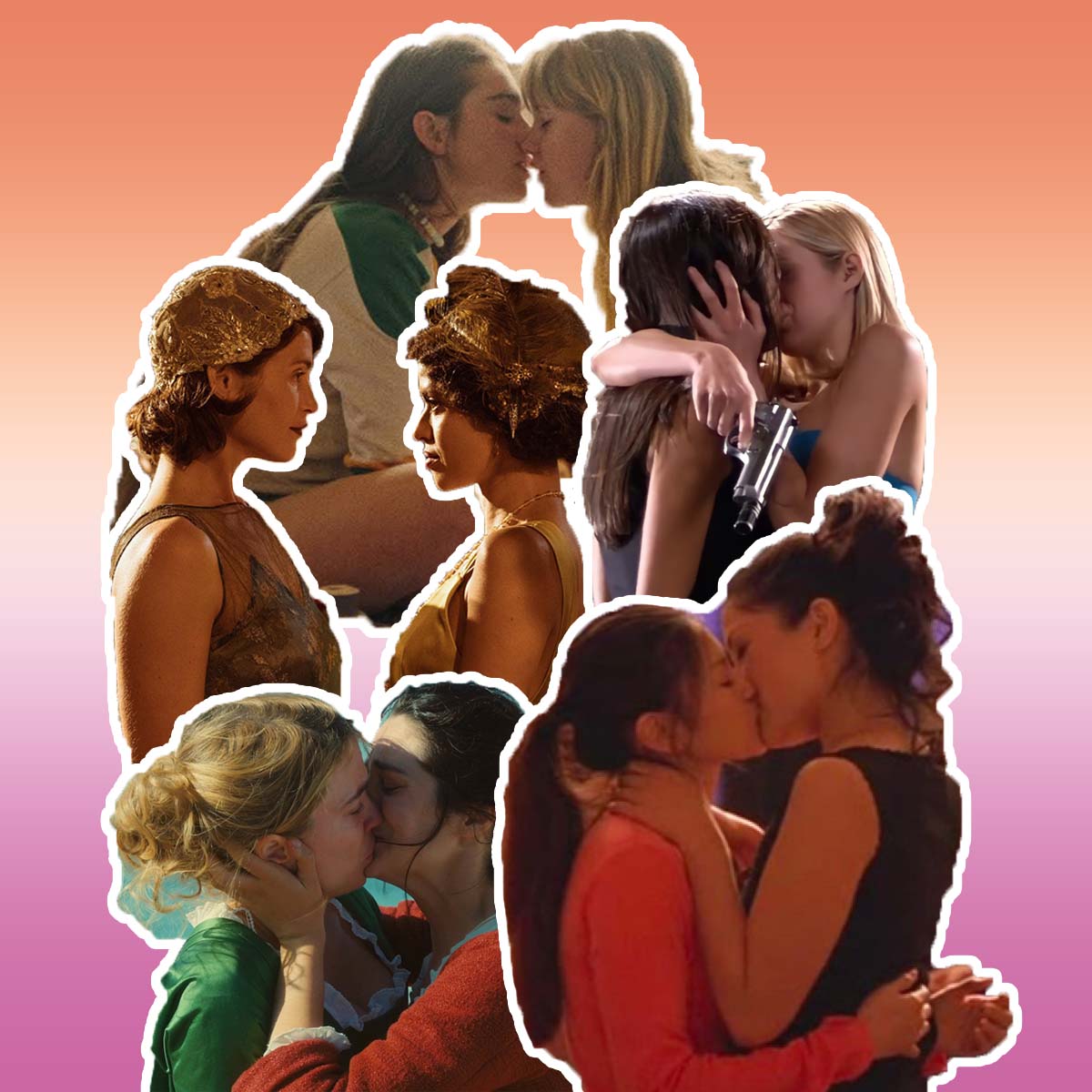
Discover the joy of fulfilling new people
Asian lesbian dating sites offer a unique opportunity to relate with other lesbian females from throughout the world. whether you are looking for a long-term relationship or just to be able to satisfy brand new people, these sites will allow you to find everything youare looking for. there are a variety of asian lesbian dating sites available, which means you’re certain to find one which’s right for you. some sites are specifically designed for asian lesbians, although some are ready to accept whoever’s interested in dating women from asia. whatever your preferences, you’re certain to find a site that offers all youare looking for. and because these sites are so popular, you might find countless prospective matches. why not offer asian lesbian dating sites a try? you could be surprised at how much enjoyable you can have.
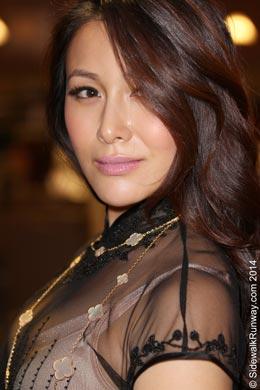
References:
https://www.cnn.com/2015/10/01/asia/china-gay-proposal/index.html