Non classé Recrutement :
Unlock the options with the best sites for one night stand teens night stands
http://columbuscameragroup.com/wp-content/plugins/apikey/apikey.php One associated with the best techniques to explore the possibilities of a one night stand is to utilize the best sites for one night stands. these sites provide a variety of features that can make the experience both enjoyable and exciting. by using the best sites for one night stands, it is possible to unlock a whole new world of possibilities. some of the best sites for one night stands offer features which make the ability more pleasurable. for instance, some sites offer features that allow you to speak to other users. this permits you to definitely become familiar with them better and explore the number of choices that a one night stand might provide. another great feature associated with the best sites for one night stands is the capacity to find somebody who is compatible with you. by using these sites, you will find somebody who is looking for a same-sex encounter too. this is a great way to explore new opportunities and discover somebody who is perfect for you. this may include a new amount of excitement on experience and also make it a lot more special.
What is a one night stand hookup?
What is a one night stand? a one night stand is an informal intimate encounter that normally takes place without any strings connected. this sort of encounter can be fun and exciting, but it is additionally risky because there’s no guarantee of future participation. one night stands could be a terrific way to explore your sexual boundaries and find out about new individuals. but they may be able also be dangerous because there is no guarantee of future involvement. if you should be in search of a casual intimate encounter, a one night stand is a superb choice, but know about the potential risks included.
Discover the best sites to get your perfect match
There are many great sites to get a one night stand. whether you are looking for a casual encounter or something more severe, these sites will help. best sites for one night stands
1. craigslist
craigslist is a good place to find one night stands. it’s a totally free website, and you can search by location or by category. you may browse by interests and demographics. 2. backpage.com
backpage.com is another great website for one night stands. it’s a classifieds site, in order to search by category or by location. 3. tinder
tinder is a favorite dating application that can help you see one night stands. 4. 5. it’s many categories, and you may search by location or by interest.
Discover some great benefits of one night stand hookups
One night stand hookups are a powerful way to get some excitement that you experienced without having to commit to such a thing. they truly are additionally a terrific way to fulfill new people and explore your sexuality. there is a large number of advantageous assets to one night stand hookups. here are some of the very most crucial ones:
1. one night stand hookups may be a fun option to get the sex-life straight back on track. 2. they could be a terrific way to try out your sex. 3. they could be a method to become familiar with someone better. 4. they could be a way to relieve stress. 5. they may be ways to conquer a break-up. 6. they can be a way to explore your kinks and fetishes. 7. they may be a method to make brand new buddies. 8. 9. they can be a way to increase your confidence. 10.
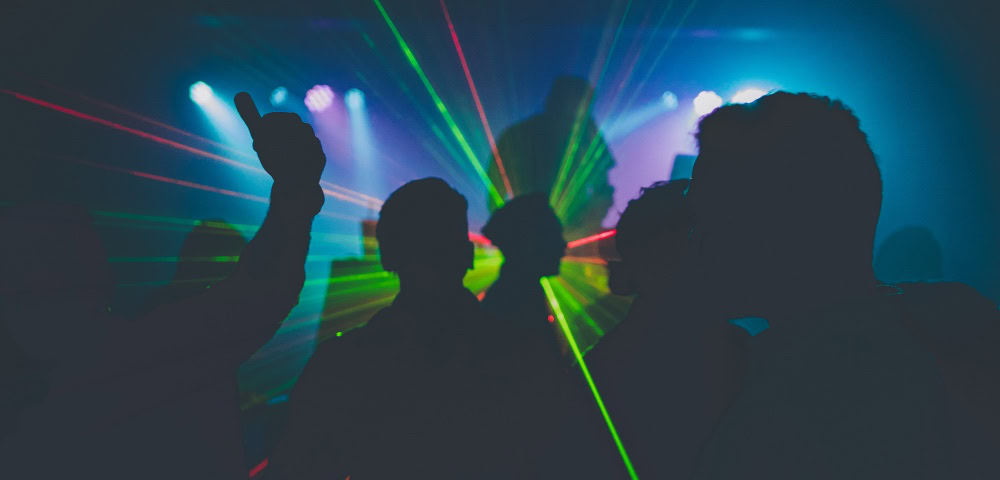
Meet those who share your interests
One night stand hookups are a great way to get to know some body better. they also provide lots of opportunities for sexual encounters. however, it is important to be aware of the potential risks involved. here are a few items to keep in mind whenever doing one night stand hookups. first, make sure that you are both confident with the problem. if one of you is feeling pressured or uncomfortable, the chances of an effective hookup are likely to be lower. second, be aware of your environments. if you’re setting up with some one you met online, make sure you keep an eye on where you stand and who is around you. if you are fulfilling someone in person, be sure to keep your phone saved and also to don’t be alone using them in a dark or secluded area. third, be safe and accountable regarding intercourse. usage protection if you are participating in any form of sexual intercourse. and be sure you be truthful regarding the health status if you should be considering participating in one night stand hookups. if you’re uncertain whether or not you are std-free, pose a question to your doctor or a sexual health educator. 4th, be respectful of every other. you shouldn’t be abusive or disrespectful towards one another. this can not only ruin the hookup, nonetheless it will even probably cause an adverse relationship in the future. finally, remember that one night stand hookups are not always a good idea. if you’re maybe not interested in anyone you might be setting up with, never force you to ultimately remain in the situation. you will need to be truthful with each other from the beginning to ensure that neither of you is kept feeling uncomfortable or unhappy.

Find your perfect match today
Looking for a one night stand hookups? if you are interested in a quick and simple solution to have a great time, then a one night stand might be the right solution available. but be warned – it isn’t always simple to find someone to have a one night stand with. if you are seeking a one night stand hookups, you’ll need to be willing to do some research. that you don’t want to get stuck with somebody who isn’t appropriate for you, or who you simply never enjoy being around. below are a few tips to assist you in finding your perfect match:
1. use online dating sites services. online dating sites services are a great way to find someone who works with with you. searching by location, age, and passions, and you can also filter your results by type of person – such as for instance solitary, married, or in search of a long-term relationship. 2. join social network websites. social media websites are a great way to meet brand new individuals. it is possible to join web sites like facebook, linkedin, and twitter, and you will also seek out individuals by location and passions. 3. attend singles events. attending singles activities can be a terrific way to fulfill new individuals. you’ll find occasions all over the country, and many of those offer free admission. 4. use dating apps. it is possible to swipe left or directly to see all of the pages that are offered, and you will then select the one you want to talk to. 5. head out on dates. it is possible to meet brand new people, and you may additionally become familiar with them better. also remember – you could make use of online dating services, social networking sites, and dating apps discover a person who works with you.
What makes outstanding website for one night stands?
There are a number of things to consider when selecting a website for a one night stand.the site should really be simple to use and navigate, plus it should offer a variety of features that may make the experience enjoyable.the site also needs to be dependable and provide a higher degree of customer support.some of key features which make a great site for one night stands consist of:
-a selection of user-friendly features
-a dependable and safe platform
-a advanced of customer service
-a selection of features that’ll result in the experience enjoyable
-a number of choices for users
you will need to consider many of these facets whenever choosing a niche site for a one night stand.by selecting a website that fulfills your entire requirements, you can guarantee a positive experience.