Non classé Recrutement :
Find local lesbians now
where to buy prednisone 5mg Looking for local lesbians? you’re in luck! using the right tools, you can find lesbian singles in your area right away. first, use online dating sites for connecting with lesbian singles locally. these sites provide a variety of features, including the power to create a profile, send messages, and fulfill other lesbian singles. next, utilize social media to connect with local lesbian singles. many lesbian social networking sites, such as for instance facebook and twitter, enable users in order to connect with others inside their area. you may want to utilize these sites to fairly share pictures, articles, and videos about lesbian life. finally, go to local lesbian activities. these occasions could possibly localhookupmail.com/local-lesbian.html offer you the opportunity to meet local lesbian singles and find out about their tradition.
Meet lesbians in your city easily
Are you looking someplace to meet up with local lesbians? well, you are in luck! with the right tools, it is possible to find and connect to lesbian buddies in your city. here are a few suggestions to help you to get started:
first, use social media to find lesbian friends in your area. numerous social network are especially for lesbians, and these platforms provide a terrific way to relate genuinely to other women. unless you have social networking, take to searching for lesbian meetups in your city. these activities in many cases are arranged by local lesbian groups, and they are a great way to meet other females and find out about town. 2nd, go to local events. numerous cities have events specifically for lesbians, and these activities are a terrific way to satisfy other females and learn about the city. unless you have time to attend an event, try trying to find lesbian-focused activities online. there are a number of occasions available, so that you’re certain to find something that’s perfect for you. finally, encounter local lesbians face-to-face. if you’re uncomfortable using social media marketing or occasions, meeting up with local lesbians in person may be a powerful way to connect to them. there is local lesbian meetups on the web, or you can simply ask around for suggestions. fulfilling with local lesbians is a superb solution to get to know them and find out about the city.
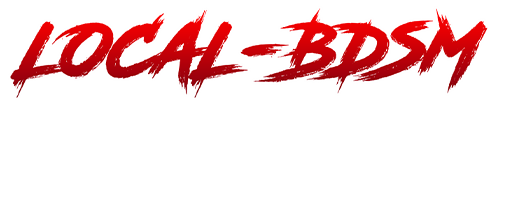
Meet lesbian singles whom live near you
Are you trying to find a lesbian dating partner whom lives in your area? in that case, you are in luck! there are many lesbian singles surviving in your neighborhood that would like to get to know you better. here are five strategies for fulfilling local lesbians:
1. join a lesbian dating internet site. one of the better approaches to meet local lesbians is join a lesbian dating internet site. these sites are made especially for lesbian relationship, plus they offer a number of features that produce them well suited for finding a partner. 2. attend a lesbian meetup. these events are usually held in pubs or restaurants, and they offer a good opportunity to network along with other lesbian singles. 3. finally, you can even meet local lesbians by attending a lesbian event. 4. join a lesbian social media marketing team. these teams are typically dedicated to networking and linking with other lesbian singles, in addition they offer a good opportunity to satisfy new individuals. 5.
Get to understand local lesbian singles and find love inside area
Site for local lesbian singles is an excellent solution to satisfy brand new individuals and discover love. with many individuals surviving in close proximity to each other, it is not surprising that finding love is a lot easier than ever. whether you are looking for a long-term relationship or simply anyone to spend time with, site for local lesbian singles will allow you to find that which youare looking for. there are a variety of great how to utilize site for local lesbian singles. you can make use of the site to find friends and speak to other lesbian singles. it is possible to use the site to find times. if you’re looking for a critical relationship, site for local lesbian singles can help you find the appropriate person. if you’re searching for ways to meet new people and find love, site for local lesbian singles is a great solution to start.
Discover a fresh realm of exciting local lesbian dating
If you are considering a fresh world of exciting local lesbian dating, you are in fortune! as a result of the internet, there are now a number of dating sites and apps available to connect to lesbian singles in your town. whether you are looking for an informal date or a more serious relationship, there’s a website or application for you personally. listed here are five of the best local lesbian internet dating sites and apps currently available:
1. the woman
the lady the most popular online dating sites for lesbian ladies. it offers a user-friendly software and is packed with features, including a talk space, a forum, and a matching system that can help you see your perfect match. 2. tinder
tinder is a dating software that has become popular within the last several years. it’s user friendly and it is ideal for people that are trying to find a quick date. 3. bumble
bumble is a dating application that’s created specifically for lesbian women. this has a unique way of matching than many other online dating sites, that makes it more likely that you will find a match you are suitable for. 4. it has an array of features, including a chat room, a forum, and a matching system. 5. there are a number of other great local lesbian internet dating sites and apps available, however these are of the most extremely popular and user-friendly options. if you should be interested in a new realm of dating opportunities, decide to try one of these simple sites or apps!
Find your perfect match: join our site for local lesbian singles
Looking for a romantic date or a relationship? discover our site for local lesbian singles! our site is designed to assist you in finding your perfect match. join our community and talk to other lesbian singles. you can find somebody who shares your passions and whom you can relate to on a personal level.