Non classé Recrutement :
Make a connection with other gay bdsm enthusiasts
Amesbury In the modern world, it’s not unusual to get individuals of various different backgrounds and passions interacting online. whether you’re a fan of activities, music, or perhaps spending time with buddies, you can find a variety of online communities to become listed on. one of the more popular online communities may be the gay community. gay bdsm dating online is a good method to connect to other gay bdsm enthusiasts in order to find new friends. additionally it is a terrific way to find love. if you’re wanting a way to relate to other gay bdsm enthusiasts, then you must look into utilizing online dating services. there are a variety of online dating solutions that cater especially to your gay community. there is services that provide a variety of features, including gay bdsm dating. they provide many different features, including gay bdsm dating, and they are a powerful way to find new buddies.
Find love & romance with all the most readily useful gay dating sites
If you are looking for a spot discover love and love, you ought to consider some of the best gay dating sites available to you. these sites offer many different features that can make finding someone simple and enjoyable. 1. gaydar.com
this website is one of the most popular alternatives for gay dating. it offers many different features, including a search engine that can help you see some body in your area. you could join forums and forums to meet local gay up other folks. 2. grindr
grindr is another popular site for gay dating. 3. adam4adam
this website is designed for people who are looking a long-term relationship. it includes many different features, including an email board where you are able to publish messages and find times. 4. the gay males’s chorus of l . a .
this site is made for those who are trying to find a place to generally meet other gay guys. 5. the gay & lesbian chamber of commerce
this website is made to help you find companies that focus on the gay community. it includes a search engine that will help you find companies in your area. 6. the gay & lesbian nationwide hotline
this is a nationwide hotline that provides guidance and help services for those who are gay or lesbian. 7. gay.com
this site was created to support you in finding someone to date. 8. the gay & lesbian times
this might be a national magazine which specialized in the gay and lesbian community. 9.
Find love and companionship with gay senior dating
If you’re looking for a brand new dating site to explore, then you should truly think about gay senior dating internet sites. these websites are specifically designed for individuals over 50 years old, and additionally they offer a lot of wonderful features which can be perfect for individuals of that age bracket. among the best things about these websites is the fact that they feature some different choices for dating. you can find people that are searching for a serious relationship, or you can simply enjoy some casual dating. additionally countless different types of people on these websites, so that you’re sure to find an individual who you connect with. another neat thing about these sites is they’re extremely active. you can always find new visitors to date, and you can additionally find folks who are looking for a long-term relationship. which means that you are sure to find the right person for you, regardless of what your preferences are. general, gay senior dating web sites are a powerful way to find love and companionship. they feature some great benefits, and they’re extremely active.
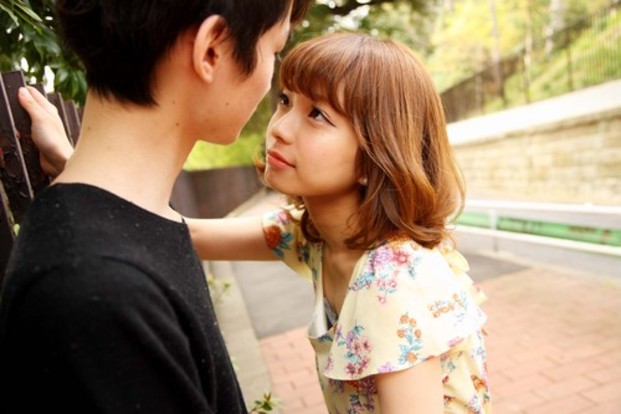
Uncover some great benefits of top 10 gay dating sites
The advantages
about dating, everyone has unique preferences. whether you are considering some one with comparable interests, a person who shares your values, or a person who just may seem like good person, there’s a dating site for you personally. but which one is the better? to find out, we viewed the top 10 gay dating sites and evaluated their features and advantages. 1. grindr
grindr may be the number 1 gay dating site on the planet, and for valid reason. it has a user-friendly interface, a great deal of features, and a wide range of users. one of the primary benefits of grindr is it is perhaps one of the most comprehensive sites. you will find users from all over the globe, therefore you’re more likely to find someone who shares your passions. another great feature of grindr is its talk function. you can keep in touch with other users directly, that makes it easy to connect and discover more about them. 2. adam4adam
adam4adam is another great option if you are interested in a website with an array of users. adam4adam comes with an excellent talk function, which makes it simple to connect with other users. 3. 4. 5. 6. one of the biggest benefits of tinder is its chat function. 7. 8. 9.
References:
https://www.vogue.co.uk/article/mona-tougaard-british-vogue-cover-interview