Non classé Recrutement :
Experience the excitement of meeting local singles
someway Experience the thrill of meeting local singles with a local fuckbook! whether you are considering a fresh friend or a serious relationship, a local fuckbook can help you find what you’re looking for. with a fuckbook, it is simple to find singles that are interested in similar activities and interests. plus, it is possible preview localhookupmail.com to satisfy new individuals locally without having to go out of your path. so what are you currently looking forward to? get a local fuckbook and start meeting brand new singles today!
Get started now: create your profile on a local fuckbook
When it comes to dating, there are a lot of choices available. but imagine if you wish to find someone who lives near by? or what if you intend to date an individual who is in your area? you can make use of a local fuckbook discover these people. a local fuckbook is an online site that allows individuals find both. it is possible to produce a profile on the internet site and list your interests and hobbies. you could list your location and that which you’re looking for in somebody. there are a lot of local fuckbooks available to you, so that you’ll have no problem finding the one that’s suitable for you. of course you are looking for something specific, you can look for it. when you’re looking to locate you to definitely date or just earn some new buddies, a local fuckbook may be the perfect choice.
Find your perfect local fuckbook match now
Finding your perfect local fuckbook match is currently easier than ever before! with many possibilities, it may be hard to understand where to start. fortunately, we have built a list of the most effective local fuckbook sites to assist you get the perfect partner. whether you are looking for an informal hookup or a long-term relationship, these internet sites have you covered. 1. craigslist
craigslist is probably the many well-known local fuckbook internet site. with an incredible number of users, it’s the perfect spot to find someone who’s shopping for an informal encounter. 2. backpage
backpage is a popular internet site for finding intercourse employees. with an incredible number of advertisements, it’s the perfect place to find a local fuckbook match. 3. eros
eros is a favorite dating internet site for people into the bdsm community. with millions of members, it is the perfect place to find somebody who shares your interests. 4. grindr
grindr is a popular app for homosexual and bisexual males. 5. tinder
tinder is a popular app for dating.
Make brand new connections with like-minded individuals inside area
Making new connections with like-minded individuals locally is very important for discovering the right partner, whether you’re looking for a casual hookup or a long-term relationship. if you’re perhaps not linked to individuals around you, you are at a disadvantage about finding love. there are numerous of approaches to make new connections. you’ll join a club, attend a meetup, or be involved in a social media team. you may want to join a dating site or app. whatever route you select, be sure to research the options first. you never wish to waste your time or cash on a website or software that isn’t ideal for you. also keep in mind to network with individuals you know. inquire further for recommendations or even for advice on how to make probably the most of one’s brand new connections.
Meet new individuals: relate with local singles on a fuckbook
Looking to meet up with brand new people and relate solely to local singles? look no further than a local fuckbook! these books are a great way to find anyone to have fun with making new buddies. plus, they are a terrific way to find someone who you are compatible with. there are a number of different local fuckbooks available online. you can find them in bookstores, or on the web. a very important thing about them is they’re always up-to-date. so, you may be certain the folks you find in a local fuckbook are the genuine deal. there are numerous of various ways to use a local fuckbook. you should use it to get anyone to day. a very important thing about local fuckbooks is that they are always changing. so, there is a constant understand who youare going to find using one. so, if you’re selecting something not used to do, or someone to speak to, a local fuckbook could be the option to go.
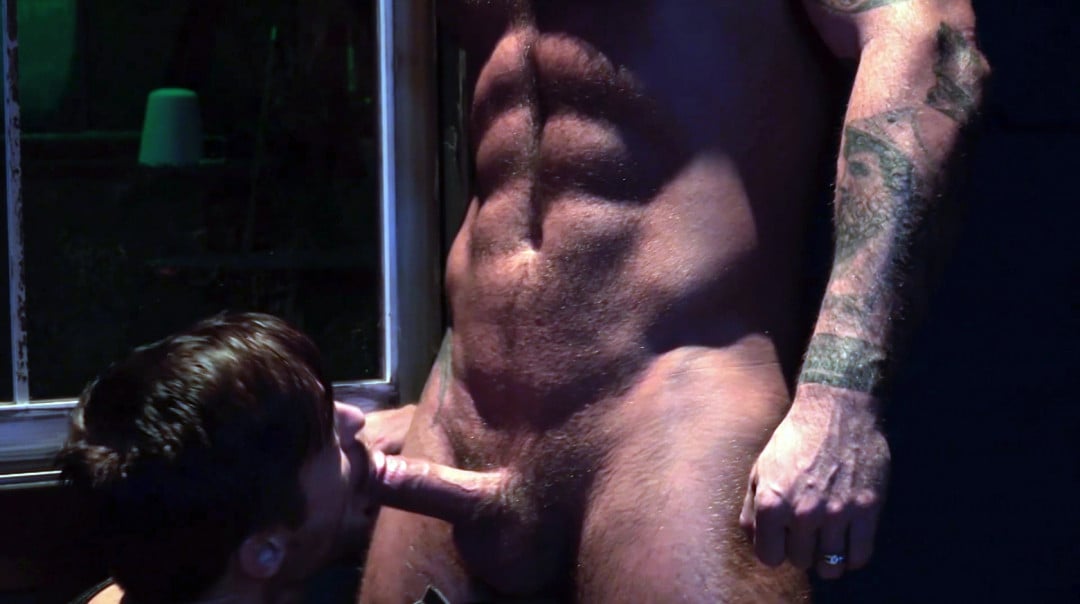