Non classé Recrutement :
Find an ideal lesbian hook up with womenup with our site
Maplewood Looking for a lesbian hookup? look no further than our site! we’ve the very best choice of lesbian hookups in your community, so that you’ll make sure to discover the perfect one for you. plus, our site is updated daily with the newest lesbian hookups in the region, so that you’ll also have the very best possibilities. just what exactly have you been awaiting? start browsing our site today in order to find the perfect lesbian hookup available!
Get started with lesbian hookup chat now
If you are considering a method to relate to other lesbians, then you should consider using lesbian hookup chat. this sort of chat is ideal for people who desire to relate with other lesbians in an informal and safe way. lesbian hookup chat is a good option to fulfill brand new people making brand new friends. plus, it’s a great way to get to know the people that you’re interested in. there are a great number of great things about lesbian hookup chat. for just one, it’s a great way to fulfill brand new people. you can find people from all around the globe on lesbian hookup chat. plus, the chat is definitely open, to help you join anytime. another neat thing about lesbian hookup chat usually it is a safe place. you can feel safe on lesbian hookup chat. plus, the chat is moderated, to help you be sure that the discussion is safe. you’ll chat aided by the people that you are enthusiastic about and find out if there is a connection. if there is, you could start dating. or even, it is possible to nevertheless take pleasure in the chat and also make new friends.
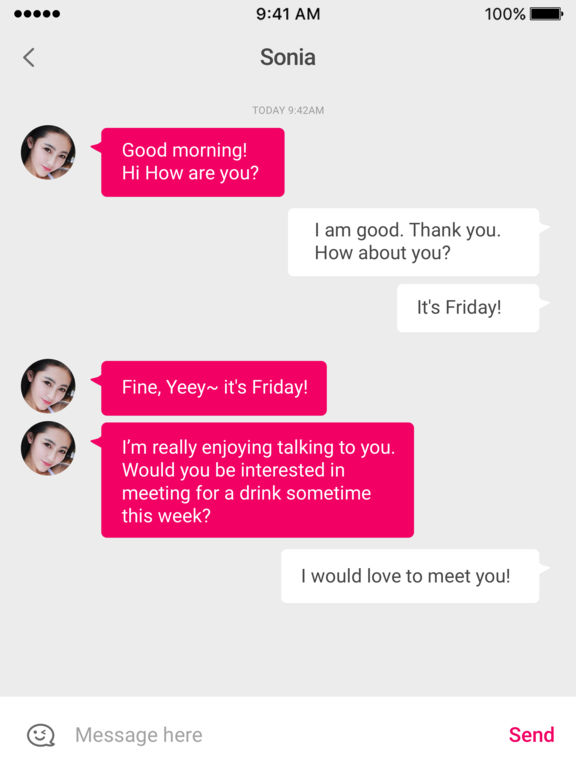
Enjoy a safe and secure lesbian hookup app uk
There are several great lesbian hookup apps readily available for users discover and relate with other lesbian singles.whether you’re looking for a casual fling or something much more serious, these apps will help make your research easier.one of the very popular lesbian hookup apps is her, which was launched in 2013 and it has since gotten one of the more popular lesbian dating apps in the marketplace.her provides a variety of features, including a chat software, photo sharing, and a search function that means it is simple to find other lesbian singles in your town.another great app for lesbian dating is bumble, that has been created in 2014 and has since become very popular dating apps for females general.bumble is different than other apps for the reason that it needs both users to start a conversation first by giving an email.after a conversation has started, users can then determine when they desire to meet in person or carry on the conversation over text.if you are looking for a more personal lesbian dating experience, there are a variety of apps offering a more concealed environment.apps like the girl and bumble are great for finding matches with individuals in your town, but if you’re looking for a far more private experience, apps like secret and appear.me are perfect for you.these apps are created to keep your matches private by just showing you individuals who you’ve shared buddies with.whatever app you decide to make use of, always take precautions to make sure a safe and protected experience.always utilize a secure password and don’t share an excessive amount of details about yourself online.and finally, do not forget to utilize good sense whenever fulfilling brand new individuals.
Get started in order to find your match today
If you are considering a lesbian hookup, you have visited the right spot. in this essay, we will coach you on how to begin and find your match today. first, let us discuss what a lesbian hookup is. a lesbian hookup is a casual sexual encounter between two women. it may be any such thing from kissing to intercourse. there are a few things you must know just before get started. first, lesbian hookups are not constantly effortless. you need to be comfortable with your sex and then communicate with your partner. 2nd, always are both safe. usage protection if you’re unsure just how your spouse will react. and keep in mind, remember that you will be both eligible to your personal human body and may never ever be forced to do just about anything against your will. finally, you shouldn’t be afraid to experiment. you won’t ever know what will happen when you connect with a lesbian. therefore head out and have now some fun!

References:
https://www.thelist.com/469228/inside-margaret-chos-dating-history/