Non classé Recrutement :
Get prepared for an enjoyable and exciting gay bear hookup bear dating experience
http://city-made.com/?p=1128 Are you ready for an enjoyable and exciting gay bear dating experience? in that case, then chances are you must take a look at gay bear dating app! this app is ideal for those who are looking for a dating experience that is both fun and exciting. with this particular app, you’ll be able to to connect along with other gay bear singles and have some fun. if you are wanting a dating app which both enjoyable and exciting, then chances are you should browse the gay bear dating app.

How to find your perfect gay bear date
Finding your perfect gay bear date could be a daunting task, however with a little bit of research and effort, it can be a lot of enjoyment. below are a few tips to help you get started:
1. start with searching on the web. there is a large number of great dating sites around specifically made for gay bears, as well as offer a number of features that will make finding a date easier. 2. be open-minded. it is vital to remember that not absolutely all gay bears are the same, therefore don’t be afraid to experience various dating sites and discover what realy works best for you. 3. anticipate to place in a little bit of work. dating could be lots of fun, but it is also essential to be willing to devote a little bit of effort. meaning being prepared to go out while having some fun, being ready to be yourself. 4. be patient. it can take a while to find your perfect gay bear date, therefore show patience and do not give up the search.
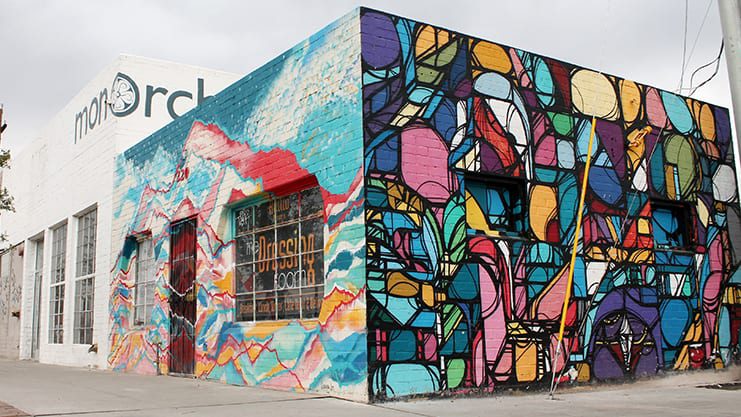
Sign up now in order to find the love in your life on gay bear dating website web site
If you are considering a dating site that caters specifically to gay bears, then you’re in luck! gay bear dating website web site could be the perfect spot to help you find love. with this site, you can relate to other gay bears who are shopping for a serious relationship. you’ll also have the ability to learn about the newest dating styles and techniques, in addition to uncover advice on how exactly to improve your dating abilities. so just why maybe not sign up now and find the love of your life on gay bear dating website web site? you won’t be disappointed!
Why gay bears dating could be the perfect place to find love
When you’re looking for love, there are a great number of places it is possible to go.you can go out with friends, go on dates, or perhaps you can even try on the web dating.but how about gay bears dating?why gay bears dating could be the perfect place to find love.there are lots of advantageous assets to dating gay bears.first of most, you are guaranteed in full discover an individual who is compatible with you.secondly, you’re likely to find a person who is just as interested in dating and achieving a relationship while.and finally, you’ll get to know one another a lot better than you’ll if perhaps you were dating somebody else.so if you are selecting someplace to find love, gay bears dating may be the perfect spot to start.
Get ready to find love aided by the most readily useful gay bear dating app
Are you selecting a fresh dating app to test? if that’s the case, you might look at the gay bear dating app. this app is made especially for gay and bisexual guys. it includes a variety of features that may allow it to be an ideal choice for everyone selecting a dating app. perhaps one of the most crucial top features of the gay bear dating app may be the graphical user interface. it really is built to be easy to use and easy to utilize. this makes it a fantastic choice if you are a new comer to dating apps. it really is built to be a residential area based app. this means that the likelihood is to own a big user base. this makes it a great choice for the people interested in a dating app which populated with people that are similar to them. one of the most crucial features of any dating app may be the quality associated with the matches that you make. the gay bear dating app is made to make quality matches no problem finding. this is done through the use of filters and the ability to search by location, interests, and more. overall, the gay bear dating app is a good choice for those finding a dating app that’s designed especially for gay and bisexual men.
References:
https://gizmodo.com/10-creatures-that-stole-our-hearts-in-2017-1821388972