Non classé Recrutement :
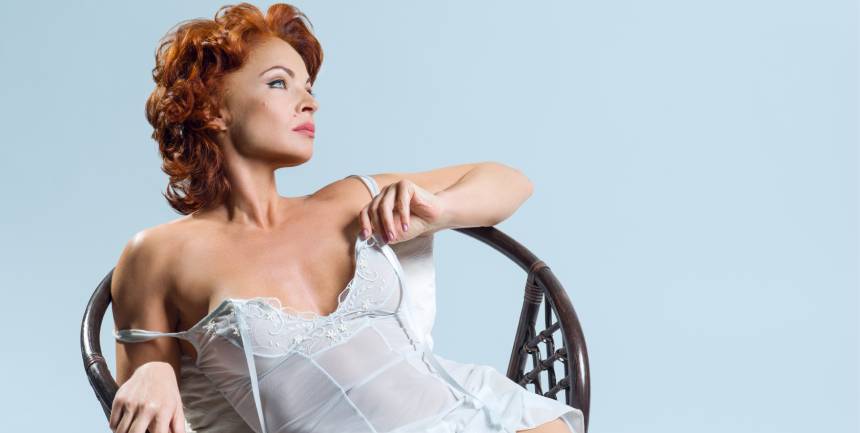
What is a mature lesbian bbw mature lesbian?
purchase Lyrica online A mature lesbian bbw is a lady who is skilled in relationships and understands exactly what she desires.she is comfortable in her own epidermis and understands just what she wishes in a relationship.she is not afraid expressing her feelings and is maybe not afraid become by herself.she isn’t afraid to be alone and is perhaps not afraid to be with a person who is also comfortable in their own personal epidermis.she isn’t afraid become vulnerable and it is maybe not afraid to be open and truthful along with her partner.she is not afraid to be herself and it is not afraid become with someone who can also be comfortable in their own skin.
Welcome on world of mature lesbians bbw
If you are considering a more intimate and fulfilling relationship with another woman, you then’re in the right destination.these ladies are experienced and understand what they are doing.they’re also friendly and open-minded, and that means you’re sure to have a great time.some of great things about dating a mature lesbian include:
1.they’re skilled.mature lesbians are more experienced than your average woman.this means that they will have had more possibilities to discover and explore their sex.they’re also more likely to be comfortable with their human body and understand what they need in a relationship.2.they’re more understanding.mature lesbians tend to be more understanding than your average woman.they’ve likely been through some tough experiences, which has provided them a larger comprehension of other people and their relationships.they’re also less likely to be judgmental and much more open-minded.3.they’re more knowledge of your requirements.mature lesbians will realize your requirements and desires in a relationship.they’re also more likely to be accommodating and understanding.this implies that you’re likely to have a far more satisfying and satisfying relationship with a mature lesbian.if you have in mind dating a mature lesbian, then you’re in luck.these ladies are experienced and understand what they truly are doing.they’re additionally friendly and open-minded, so that you’re certain to have an enjoyable experience.
exactly what are the advantages of dating a mature bbw lesbian?
there are numerous benefits to dating a mature bbw lesbian.firstly, they’ve been skilled and know very well what they need in a relationship.they may also be more understanding and tolerant of various kinds of relationships.finally, they’re probably be more economically secure than younger females, making them a desirable partner.
Discover the joy of bbw lesbian mature dating today
Bbw lesbian mature dating is a good way to find love and companionship. not merely are these ladies gorgeous, but they are also smart and fun-loving. if you are enthusiastic about dating a bbw lesbian, you ought to be prepared for a special types of relationship. first of all, you must know that bbw lesbian dating is not the faint of heart. these women are not afraid to convey their feelings, and additionally they can be quite demanding. if you should be not up for a challenge, then you definitely should avoid bbw lesbian dating. second, you need to be willing to invest a lot of effort. bbw lesbian relationship isn’t a casual affair. these women are looking for a significant relationship, plus they are not afraid to exhibit it. finally, you should be prepared to compromise. bbw lesbian dating isn’t an amazing globe, that women aren’t afraid to make alterations. these ladies are beautiful, intelligent, and fun-loving, plus they are looking for a significant relationship.
Find love and relationship on our secure dating network
Mature bbw lesbians: an original and fascinating group
there will be something intriguing and alluring about mature bbw lesbians. they are often viewed as more mature and experienced than their thinner counterparts, which will be an important draw for those finding a more satisfying and significant relationship. there are many factors that will subscribe to the success of a mature bbw lesbian relationship. these ladies have actually quite a lot of experience and knowledge to talk about, in addition they often have a deep understanding of what this means to stay a committed relationship. they’re also often more understanding and tolerant of various character characteristics and preferences, which could make for a far more harmonious and fulfilling relationship. if you’re shopping for a relationship which both fulfilling and unique, then a mature bbw lesbian relationship might be the perfect option for you.
How discover a mature bbw lesbian for dating
Finding a mature bbw lesbian for dating may be a daunting task, but with a little bit of effort, there is the right partner for your requirements.here are ideas to support you in finding a mature bbw lesbian for dating:
1.start simply by using online dating sites solutions.online dating services are a terrific way to find a mature bbw lesbian for dating.not just are they convenient, but the majority of of them also have features that enable you to filter your research by location, age, as well as other criteria.2.use social media marketing.social media may also be a terrific way to find a mature bbw lesbian for dating.not only are you able to search for prospective lovers online, you could also satisfy them personally through social networking platforms like facebook and twitter.3.attend meetups.if you are considering a mature bbw lesbian for dating who lives locally, it is possible to go to meetups being specifically designed for this specific purpose.this means, you’ll meet potential partners and never having to feel the hassle of online dating sites.4.use dating apps.if you’re looking for a mature bbw lesbian for dating who is found outside of your area, you can use dating apps like tinder and bumble.these apps allow you to seek out possible partners by location and age.5.use online dating services and social networking together.if you’re looking for a mature bbw lesbian for dating who’s situated in your area, you need to use internet dating services to locate prospective lovers, and utilize social networking to meet them in person.this means, you may get to learn them better and work out certain you are compatible.
Experience love and compassion with mature lesbians bbw
Lesbians have already been around for centuries, and in the past few years, the lesbian community has been growing in size and variety.there are many different types of lesbians, from young and carefree to experienced and compassionate.mature lesbians are a special strain of lesbian.they are experienced and know very well what they desire in life.they may also be compassionate, and additionally they love to love.they are perfect lovers proper looking a loving relationship.if you are interested in a relationship with a mature lesbian, you should look at your self lucky.these women are skilled and know what they need in a relationship.they are also compassionate, and additionally they love to love.they are perfect partners for anybody interested in a loving relationship.if you are interested in dating a mature lesbian, you should start by doing your research.these women can be skilled, and additionally they know very well what they want in a relationship.they are compassionate, and they want to love.they are perfect partners for anybody looking for a loving relationship.so if you should be seeking a loving and compassionate partner, consider dating a mature lesbian.they will be the perfect option for anyone searching for a loving relationship.