Non classé Recrutement :
what exactly is a milf and exactly why you ought to find one
http://sjfiremuseum.org/export.php What is a milf? a milf is a female who’s over the age of 35. she’s typically regarded as being more capable than a young girl, and is usually seen as a more desirable partner. milfs are often sought after by guys since they’re more likely to be skilled and know what they need in a relationship. also more likely to be comfortable with being sexual and are usually usually more ready to accept brand new experiences. why wouldn’t you find a milf? there are numerous factors why you should find a milf. first, they’ve been typically more capable than ladies. which means they truly are more prone to understand what they want in a relationship and are almost certainly going to be more comfortable with being sexual. also more likely to be open to brand new experiences, which is often a terrific way to add spice to your relationship. in addition, milfs tend to be more learning and patient than ladies. which means that they have been likely to be more forgiving and able to handle hard situations better. summary
finding a milf is a superb method to enhance your relationship and atart exercising . additional excitement to your life. they’ve been typically more experienced and understand what they want in a relationship, which will make for a more fulfilling experience.
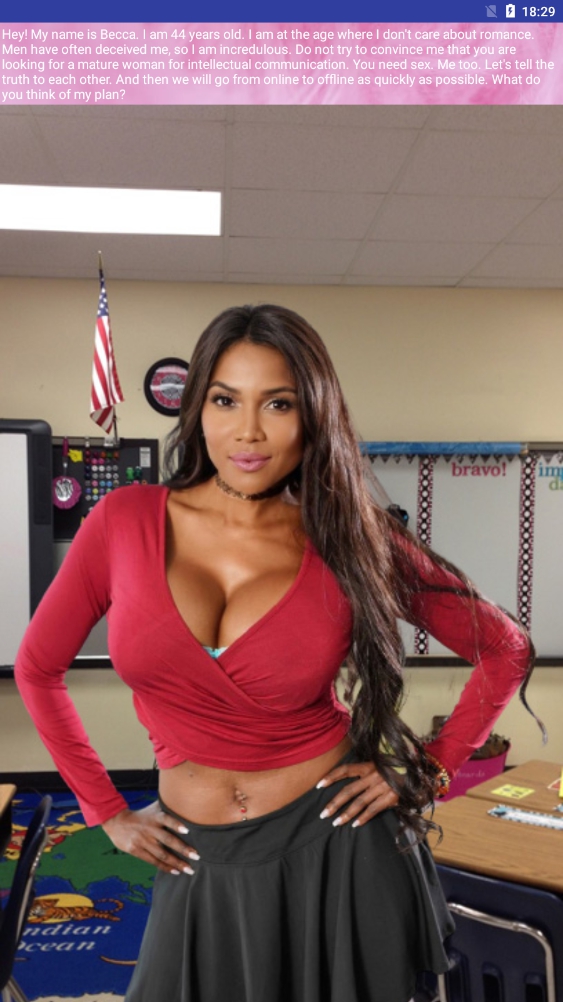
Find local hairy milfs – fulfill your ideal date now
Finding a hairy milf is a dream become a reality for all men. not only do these ladies have actually hairy pits, nevertheless they also have hairy feet, arms, and chests. plus, they’re usually quite busty too, so you’re in for an actual treat. if you are looking for a hairy milf up to now, you are in fortune. there are plenty of them available to you, and you can find them by using the tips in this article. first, you’ll want to use the internet to find local hairy milfs. this is the quickest and easiest way to find them, and you can even use engines like google. next, you’ll want to go to milf events. this is a great way to meet hairy milfs and move on to know them better. you are able to find these occasions through the use of on the web listings or by trying to find « milf events » on the web. finally, it is in addition crucial to meet hairy milfs in person. this is actually the easiest way to access know them also to find away if you have an actual connection with them. it is possible to find hairy milfs by using online dating sites solutions or by meeting them in person.
How to find milfs near you in just some steps
If you are considering ways to meet milfs near you, then you’re in luck! in this article, we’ll explain to you how to find milfs near you in only several easy steps. first, you will want to make an online search to find milfs near you. there are a number of various internet sites that offer this solution, therefore it is up to you what type you decide on. when you have found a web page that offers this solution, you will have to enter your zip code. this can allow you to find all the milfs in your area. next, you need to search for milfs who’re online. which means these are typically presently creating an online business and are also for that reason available to satisfy. once you’ve discovered a milf that is on the web, it’s also important to start messaging the lady. this is the most important the main procedure, since it will help you become familiar with the lady better. if you should be thinking about fulfilling the milf personally, then you definitelywill need to set up a gathering. this is done by utilizing a dating software or by meeting the woman in person. once you’ve met the milf, it is the right time to take what to the following level. it is possible to either have sex along with her or date the lady. in any event, it will be a great experience!
Find the perfect milf in coffs harbour today
Looking for a milf in coffs harbour? if you’re in search of a milf in coffs harbour, you’re in luck! there are many hot milfs around that ready and willing to have a blast. whether you’re looking for a one-time hookup or something like that more serious, you can actually find the right milf available in coffs harbour. keep in mind to make use of lsi keywords and long-tail key words when looking for milfs in coffs harbour, to find the very best site to find milfs match to your requirements. also remember to say « find coffs harbour milfs » within search!
Find local hairy milfs – meet hot mature ladies in your area
Looking to satisfy some hairy milfs in your town? well, you are in fortune! with the aid of some long-tail key words and lsi keywords, it is simple to find local ladies who love to get their hairy pits waxed. not just will you be in a position to find some amazing women currently, however you will additionally be able to find some hairy milfs who’re up for such a thing. if youare looking for a naughty date, or just some business while you are out checking out your town, look absolutely no further compared to the hairy milfs in your town. delighted hunting!
Tips to create outstanding first impression and acquire a date
So you’re thinking about dating once more, while’re wondering what you should do in order to make a great first impression. well, below are a few suggestions to help you out:
1. dress well
that one is obvious, but it’s always vital that you dress well when you’re out fulfilling new individuals. that you don’t wish to encounter as too formal or too casual, but you additionally never want to appear to be a slob. find one thing in the centre that fits your character and design. 2. be yourself
don’t try to be somebody you are not. if you should be timid, don’t try to be a loud and outbound person. you need to be your self and allow other person get to know you. 3. look
smiling is one of the most important things you are able to do when you’re fulfilling brand new individuals. it implies that you are happy and enthusiastic about exactly what your partner must state. 4. be courteous
no matter what the other person says or does, be polite. this applies to men and women. if some one is being rude, don’t get crazy. just politely ignore them and move on. 5. be open-minded
don’t be afraid to try brand new things. in the event that other individual invites you down for coffee, go. if they desire to get see a movie, go. you need to be open-minded and now have fun. they’re just a few suggestions to help you produce an excellent first impression. if you follow these pointers, you’ll be certain to find a night out together that you’ll love.
.jpg)