Non classé Recrutement :
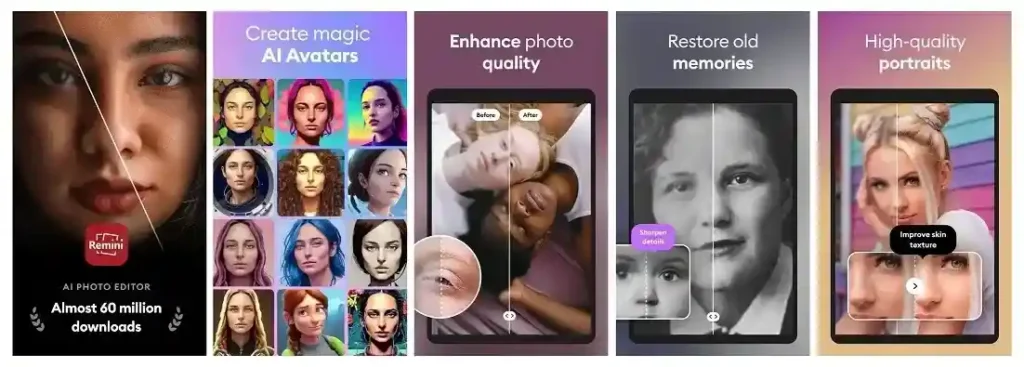
What are the advantages of bbw dating?
Sneek do you know the great things about dating a bbw? there are many benefits to dating a bbw, plus they vary with regards to the individual. many people discover that bbws tend to be more understanding and compassionate than many other forms of ladies, and they are more likely to be supportive and understanding in a relationship. also, bbws in many cases are regarded as being more physically attractive than many other women, and they are apt to have countless character. this can alllow for a fun and interesting relationship, and it can be a lot of enjoyment currently a person who is distinctive from you. another advantage to dating a bbw is they will be more intimately adventurous than other women. this is a great thing, as it can spice up your sex life and also make it more exciting. bbws are also frequently very affectionate, plus they are often more ready to express their love for you in a physical method. this can be outstanding thing, as it could make your relationship more satisfying and romantic. finally, dating a bbw could be a lot of enjoyment. they are generally really outbound and social, and they are usually really enthusiastic about trying brand new things.
exactly what is bbw dating?
Bbw dating is an evergrowing trend among singles who are finding a far more diverse dating pool.bbw dating is not merely for over weight individuals; it is proper whom is interested in dating an individual who is perhaps not the average-sized person.there are advantages to dating a bbw, such as the fact that they are usually more understanding and compassionate than many other types of individuals.bbw dating is not just for over weight people; it is for anyone whom is interested in dating an individual who is not the average-sized person.there are benefits to dating a bbw, like the undeniable fact that they are often more understanding and compassionate than many other types of people.bbw dating is not just for obese people; it is for anybody who is thinking about dating someone who is not the average-sized person.there are many benefits to dating a bbw, like the undeniable fact that they are usually more understanding and compassionate than other forms of individuals.bbw dating is not merely for obese people; it is proper who is enthusiastic about dating a person who is perhaps not the average-sized person.there are advantages to dating a bbw, such as the undeniable fact that they are often more understanding and compassionate than other forms of people.bbw dating is not just for over weight people; it is for anyone who is enthusiastic about dating someone who is maybe not the average-sized person.there are many benefits to dating a bbw, including the undeniable fact that they are generally more understanding and compassionate than many other forms of people.bbw dating is not only for overweight people; it is proper who is interested in dating someone who is not the average-sized person.there are many benefits to dating a bbw, like the fact that they are often more understanding and compassionate than other kinds of individuals.bbw dating is not just for obese people; it is proper who is interested in dating a person who is not the average-sized person.there are benefits to dating a bbw, such as the fact that they are generally more understanding and compassionate than other types of individuals.bbw dating is not only for overweight people; it is for anyone who is interested in dating somebody who is perhaps not the average-sized person.there are benefits to dating a bbw, such as the fact that they are usually more understanding and compassionate than many other kinds of individuals.bbw dating is not merely for over weight people; it is proper who is thinking about dating a person who is maybe not the plussizedatingsites.org/bbw-dating.html average-sized person.there are benefits to dating a bbw, such as the fact that they are usually more understanding and compassionate than other kinds of people.bbw dating is not just for over weight people; it is proper who is interested in dating an individual who is perhaps not the average-sized person.there are advantages to dating a bbw, such as the fact that they are often more understanding and compassionate than many other types of individuals.bbw dating is not merely for obese people; it is proper whom is enthusiastic about dating someone who is maybe not the average-sized person.there are many benefits to dating a bbw, like the undeniable fact that they are generally more learning and compassionate than other forms of individuals.bbw dating is not merely for over weight people; it is for anybody who is interested in dating someone who is not the average-sized individual.
what exactly is a bbw and exactly why should you date one?
Dating a bbw is a great method to explore your intimate dreams and find a partner who shares comparable interests. not merely are bbws curvier and sexier than the majority of women, but they also provide a unique viewpoint on life that you could not find in other females. listed here are five reasons you ought to date a bbw:
1. they truly are unique
there’s no one else quite like a bbw. they truly are curvier than most women, and their body shapes are unique. which means that you might never find a bbw who appears the identical while you do. this really is a great chance to explore your sexual dreams and discover something new and exciting. 2. they are passionate
bbws are usually really passionate and psychological. they are perhaps not afraid showing their feelings, and this can be a great solution to interact with a bbw. they’re additionally often extremely expressive during sex, meaning you can experience all of their pleasure. 3. they’re fun
bbws are usually really fun and outbound. they may be very open-minded, and they’re maybe not afraid to test new things. this means you’ll be able to explore your intimate dreams and find one thing brand new and exciting every time you date a bbw. 4. they are faithful
bbws are often really dedicated and committed to their relationships. they’re usually extremely faithful to their lovers, and they’re often very supportive. which means that you can interact with a bbw on a deeper degree than almost every other women. 5. they truly are usuallyhorny
bbws are usually extremely horny. which means you can actually experience all their pleasure, and they’re going to be able to experience yours besides.
How to generally meet and attract bbws for a fulfilling relationship
Dating a bbw could be a rewarding experience if you treat it the proper way. below are a few ideas to help you get started:
1. know your boundaries. when dating a bbw, it is vital to be clear about what you’re and generally are not comfortable with. if you’re not comfortable with being actually intimate, be upfront about this and don’t waste time wanting to date someone who isn’t appropriate for you. 2. be respectful. when dating a bbw, it’s important to be respectful of their size and weight. don’t make fun of these size or weight, and don’t act like you might be better than them due to it. 3. show patience. dating a bbw is challenging at first, but it’s worth it in the end. show patience together and give them time for you conform to dating a bbw. 4. you shouldn’t be afraid to inquire of for help. if you are struggling to date a bbw, avoid being afraid to inquire of for help from friends or family members. they may be capable provide you with some advice or assist you in finding a dating mentor. 5. be yourself. the easiest method to date a bbw is to be your self. if you are bashful or introverted, dating a bbw might be difficult. cannot try to be some one you are not.
just what is bbw dating and just why is it therefore popular?
Bbw dating is an increasing trend that is gaining interest all over the world.people are attracted to bbw dating simply because they locate them become appealing and interesting.bbw dating is not merely if you are obese, however for anybody who is enthusiastic about dating an individual who is distinctive from the norm.bbw dating is a great way to find somebody who is not like the rest of the individuals on the market.there are many reasons why individuals are drawn to bbw dating.first of all of the, bbw dating is a powerful way to find someone whom is not like the rest of the people nowadays.bbw dating is a powerful way to find someone who is not like all the other people out there.bbw dating is a terrific way to find a partner who is nothing like all of those other individuals nowadays.bbw dating is a terrific way to find someone who is not like all the other individuals around.bbw dating is a terrific way to find someone who is in contrast to all the other people nowadays.bbw dating is a great way to find someone whom is in contrast to all the other people out there.bbw dating is a great way to find someone whom is nothing like all of those other people online.bbw dating is a great way to find someone whom is in contrast to the rest of the individuals available to you.bbw dating is a terrific way to find somebody who is nothing like the rest of the individuals available.bbw dating is a great way to find someone who is not like the rest of the individuals available to you.bbw dating is a terrific way to find a partner who is not like the rest of the people online.bbw dating is a terrific way to find a partner who is not like all the other individuals online.bbw dating is a terrific way to find somebody who is not like all the other people on the market.bbw dating is a great way to find someone whom is nothing like all the other people online.bbw dating is a great way to find a partner whom is not like all of those other people around.bbw dating is a terrific way to find a partner whom is not like all the other individuals available to you.bbw dating is a terrific way to find someone who is nothing like the rest of the people nowadays.bbw dating is a great way to find a partner whom is nothing like the rest of the people available.bbw dating is a powerful way to find somebody whom is not like the rest of the individuals out there.bbw dating is a powerful way to find someone whom is nothing like all the other people online.bbw dating is a terrific way to find somebody whom is not like all the other people online.bbw dating is a terrific way to find somebody whom is nothing like all the other individuals out there.bbw dating is a great way to find somebody whom is in contrast to all the other individuals online.bbw dating is a powerful way to find somebody who is in contrast to all the other people available.bbw dating is a great way to find someone who is in contrast to the rest of the people online.bbw dating is a powerful way to find a partner who is not like all of those other individuals nowadays.bbw dating is a powerful way to find someone who is nothing like all of those other people available.bbw dating is a powerful way to find somebody whom is in contrast to the rest of the individuals around.bbw dating is a great way to find a partner whom is in contrast to the rest of the individuals available.bbw dating is a terrific way to find somebody whom is in contrast to the rest of the individuals available.bbw dating is a great way to find a partner who is in contrast to all of those other people available.bbw dating is a great way to find someone whom is not like the rest of the people on the market.bbw dating is a great way to find a partner whom is not like the rest of the people on the market.bbw dating is a powerful way to find somebody whom is in contrast to all of those other individuals on the market.bbw dating is a terrific way to find somebody who is in contrast to all the other individuals out there.bbw dating is a great way to find a partner who is in contrast to all the other people around.bbw dating is a powerful way to find somebody whom is in contrast to the rest of the individuals available to you.bbw dating is a great way to find a partner who is in contrast to all of those other individuals nowadays.bbw dating is a great way to find a partner who is nothing like the rest of the people available.bbw dating is a terrific way to find somebody whom is in contrast to the rest of the people around.bbw dating is a powerful way to find a partner who is not like the rest of the individuals on the market.bbw dating is a terrific way to find somebody whom is not like the rest of the individuals around.bbw dating is a terrific way to find someone who is not like all of those other people on the market.bbw dating is a
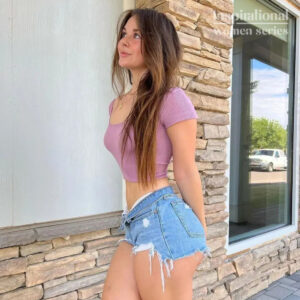
Find love with a plus-size partner: start dating a bbw now
Dating a plus-size partner are a great way to find love. plus-size dating websites are a great way to connect to people who share your passions. plus-size dating may be a great strategy for finding a partner who’s appropriate for you. plus-size dating can be a great way to find a partner who’s interested in you for who you really are, not only your size.
Ready to start out bbw dating? let us get started
If you’re considering starting bbw dating, you’re in the right place.here, we will demonstrate all you need to know to begin with.first, you will need to find a bbw dating site that is right for you personally.there are plenty of great choices available to you, so it’s important to find the one that’s right for you.some of the best bbw dating websites include bbw dating website, bbw personals, and bbw love.all of those web sites offer an excellent selection of bbw dating pages and are also ideal for those interested in a significant bbw dating experience.next, it’s important to create a profile that’s ideal for you.make certain to add most of the important info, including your age, height, weight, and interests.once you’ve developed your profile, it is time to start meeting bbw dating lovers.the simplest way to do this is to participate several bbw dating groups and start chatting with other users.finally, do not forget to keep your dating experience safe.always usage protection when dating bbw ladies, and be respectful of their privacy.if you follow these pointers, you’re going to be ready to start bbw dating in no time!