Non classé Recrutement :
Enjoy lesbian dating and flirting with our members
buy isotretinoin usa Looking for a fun and flirty method to get to know other lesbian ladies?look no longer than our online dating service!our users are of the most passionate and romantic ladies around, and they are always up for a very good time.whether you are considering a casual date or something more serious, our site has one thing for you.our website is filled with beautiful ladies who are searching for the same you’re: a link with that special someone.so why don’t you test it out for?you never understand, you might just find the next girlfriend here!so what exactly are you waiting for?sign up today and commence flirting with the ladies!
Get to understand the women in search of love on lesbian
Everyone! if you are seeking some fun that you experienced, you ought to definitely consider dating a lesbian. they’re some of the most passionate and romantic females around, and they are constantly up for a good time. if you are enthusiastic about dating a lesbian, there are some things you need to know. to start with, you have to be comfortable with your sexuality. if you should be unsure what sort of person a lesbian is, never worry. they’re usually pretty open about who they really are, and they’re not really going to judge you. next, you have to be comfortable dealing with sex. lesbians are experts at intercourse, and they are likely to wish to know every thing about this. if you’re uncomfortable dealing with intercourse, you do not be an excellent match for a lesbian. final, but not minimum, you’ll need to be comfortable being yourself. lesbians are interested in females, and that means you’ll need become comfortable with that. so, if you should be enthusiastic about dating a lesbian, make sure to take these exact things into consideration. they are certain to make your relationship experience more enjoyable.
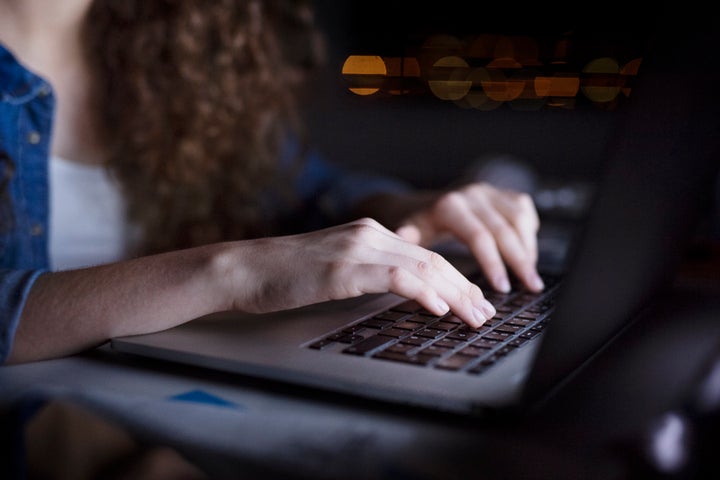
Start a conversation while making connections that last
If you are looking for a new option to relate to other lesbians, then chances are you should check out lesbian hookup chat. this on line forum is a good solution to begin a conversation and also make connections that final. lesbian hookup chat is a superb option to fulfill brand new individuals and have fun. additionally it is a powerful way to find friends and relationships.
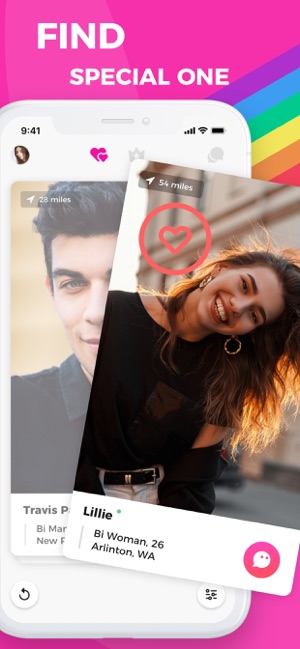
Find the right lesbian hookup with our site
Looking for a lesbian hookup? look no further than our website! we’ve the greatest selection of lesbian hookups in your community, which means you’ll make sure you discover the perfect one for you. plus, our website is updated every day with the latest lesbian hookups in your community, so you’ll always have the best solutions. so what have you been looking forward to? begin searching our site today and find the perfect lesbian hookup available!
Benefits of lesbian hookup chat
There are many benefits to engaging in lesbian hookup chat. not just does it offer a safe and comfortable room for lesbians for connecting, however it can also help to create relationships and increase interaction. very essential great things about lesbian hookup chat is it can benefit to construct trust. whenever lesbians can communicate openly and truthfully, they are prone to build trust and trust is paramount to a wholesome relationship. additionally, lesbian hookup chat will help increase communication and understanding between partners. whenever partners have the ability to explore their emotions and issues, they are able to build a stronger relationship. finally, lesbian hookup chat can help to build camaraderie and help between lesbian partners. whenever lovers are able to support and encourage each other, they have been almost certainly going to flourish within their relationships.
References:
https://www.angelfire.com/crazy/nova2x/amateur-lesbian-sex.htm