Non classé Recrutement :
Enjoy fun and engaging conversations with people from your generation
Chatrooms for seniors are a terrific way to keep busy and connected. they provide a space for seniors to socialize and engage in interesting conversations. chatrooms for seniors are a terrific way to relate to other seniors and possess enjoyable. they are a great way to stay linked to relatives and buddies.
Have enjoyable & take it easy with chatrooms for seniors
Chatrooms are a terrific way to have a great time and revel in life. also a great way to relate genuinely to other seniors. there are plenty of chatrooms available, and they all have actually different features. some chatrooms are for individuals who are looking for a dating site, while some are for those who are looking for someplace to speak about their time. whatever your needs are, there was a chatroom for you. one of the best features of chatrooms is that they have been private. this means you can keep in touch with individuals without fear of being overheard. this really is great if you should be timid, or if you are fulfilling somebody for initially. this is because seniors are often more open than younger people. also prone to have the same interests, which makes them a great resource for finding friends. if you are searching for a chatroom that is especially for seniors, be sure to take a look at options. you will find chatrooms for people of all many years, so there will certainly be one that is perfect for you.
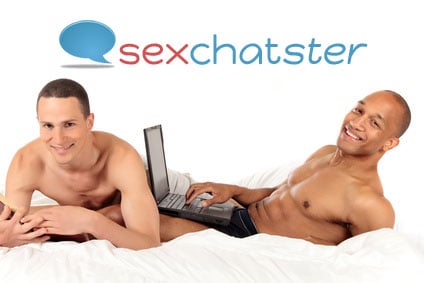
Spice your life & enjoy chatrooms for seniors
Chatrooms are a great way to stay connected with family and friends. they are able to be a powerful way to have a great time. if you’re looking for a new solution to keep busy, consider joining a chatroom for seniors. these chatrooms are perfect for those who are searching for ways to socialize and also have fun. they’re also a terrific way to connect with other seniors.
Meet new individuals and share experiences in senior chatroom
The senior chatroom is a great spot to satisfy brand new people and share experiences. it’s a fantastic destination to relate genuinely to other individuals who come in the same stage of life while, and also to share advice and tips on how to live a wholesome and satisfying life. if you are wanting a place to connect along with other seniors, the senior chatroom is the perfect spot for you personally.
Enjoy fun and exciting conversations with people your age
Looking for enjoyable and exciting conversations with people your age? look absolutely no further compared to the senior chatroom! here, it is possible to relate solely to other seniors and also have some great conversations. plus, the chatroom is an excellent option to fulfill brand new individuals and also make new friends. the senior chatroom is a good spot to discuss whatever you want. you’ll talk about your preferred topics, or just shoot the breeze. you will find always lots of people in chatroom, which means you’ll never ever be alone. whether you’re looking for you to definitely speak to, or just you to definitely speak to, the senior chatroom may be the perfect spot available. therefore come on in and now have some lighter moments!
Experience the joys of online dating – join now for free
Chatrooms for seniors are a powerful way to satisfy new individuals and also fun. also, they are a powerful way to talk to friends and family who live far. there are lots of chatrooms for seniors available, and every you’ve got its very own unique features. some chatrooms are particularly for seniors, while some are open to anybody avove the age of 18. no real matter what sort of chatroom you select, make sure you take advantage of the features it offers. many chatrooms provide chat rooms for seniors, chat rooms for singles, and forums for partners. there are also boards for children, chat rooms for gamers, and chat rooms for people who have disabilities. additionally, there are chat rooms for folks of all many years. if you’re searching for a chat room for seniors, be sure to check out the forums for seniors available. these boards were created specifically for seniors, and so they provide a variety of features which will make your experience enjoyable.