Non classé Recrutement :
Enjoy a safe & protected environment inside our gay bdsm chat line chat rooms
Genzano di Roma Enjoy a safe and safe environment inside our gay bdsm chat rooms. our chat rooms are made if you are interested in someplace to explore their kinks and fetishes in a safe and secure environment. whether you are considering someplace to role-play or just desire to speak with somebody about your fetish, our chat rooms would be the perfect place to do that. our chat rooms are moderated so that you can always feel secure and safe, and our staff can be obtained 24/7 to obtain many out of your experience. why perhaps not give our gay bdsm chat rooms an attempt today?
Welcome towards best gay bdsm chat rooms around
Whether you are looking for a spot to have some slutty fun together with your partner or perhaps desire to speak about kink along with other like-minded people, these chat rooms are ideal for you.in these rooms, you can chat with other gay men about a number of dirty subjects, from bondage and discipline to role-playing and spanking.whether you’re a newbie or a skilled player, these chat rooms are sure to have one thing for you.so then provide them with an attempt today?you defintely won’t be disappointed!
Start your dating journey now in order to find your ideal bdsm partner
If you are considering a dating adventure that’s different, then chances are you should browse gay bdsm chat. this network is filled up with people that are finding brand new and exciting methods to explore their sex. if you are interested in finding a partner who shares your interests, then gay bdsm chat is the place to be. there are lots of people who are interested in a partner that is willing to explore their sexuality in brand new and exciting ways. if you’re selecting somebody who is ready to accept brand new experiences, then gay bdsm chat is the spot to be.
Welcome to the most useful gay bdsm chat room
Our chat space is an excellent spot for people who are seeking an enjoyable and safe spot to chat with other gay bdsm enthusiasts.in our chat room, you’ll be able discover people who are interested in all sorts of gay bdsm tasks, from roleplaying to real-life intercourse.whether you might be a beginner or an experienced gay bdsm enthusiast, our chat space will certainly have a chatty member you will enjoy talking to.so have you thought to join united states today and start communicating with a number of the coolest gay bdsm chatters around?
Welcome to the ultimate bdsm chatroom
Here you will find a wide variety of people interested in exploring the kinkier part of life.whether you’re a newcomer trying to learn more about this taboo topic or a seasoned player wanting brand new lovers, the chatroom is the perfect spot to find everything youare looking for.whether you’re looking to role-play a specific scenario or simply chat with like-minded individuals, the chatroom has all you need.and if you are trying to find something a bit more personal, our personal spaces are perfect for you.so why not supply the chatroom a try today?you won’t be disappointed!
Find your perfect match today
Looking for ways to add spice to your sex-life? browse gay bdsm chat! this sort of chat is ideal for those who are seeking something new and exciting inside their bedroom. with gay bdsm chat, it is possible to explore your kinks and fetishes in a safe and personal environment. you can even find someone to share those dreams with! why maybe not try it out today?
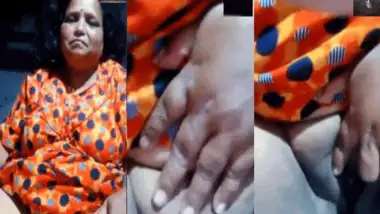
References:
https://www.themix.org.uk/sex-and-relationships/single-life-and-dating/where-to-pull-3191.html