Non classé Recrutement :
Join our women male seeking male woman website today and commence connecting
http://garrygolden.com/images If you are considering a dating website that caters particularly to women, then you definitely should definitely consider our women seeking woman website today! our website is packed with features that’ll make connecting with other women a piece of cake. and of course, our user-friendly program allows you to obtain the right woman for you. so what have you been waiting for? join our website today and start linking utilizing the women you have been looking for!
Create your profile & begin linking with like-minded women
Creating your profile on a women seeking woman website is a superb option to relate solely to other like-minded women. the website will request your title, age, location, and interests. you are able to decide to share only a little about yourself, such as your work, hobbies, or favorite things. you may create a profile for someone or a friend. once you have developed your profile, you could start linking with other users. you can chat with them, share photos, as well as meet in person. there is a large number of great advantageous assets to joining a women seeking woman website. first, you’ll find somebody or a pal who shares your passions. second, you are able to find out about brand new topics and cultures. third, there is support when it’s needed. finally, you could make brand new friends and connections. if you’re searching for a place to get in touch with other women, you need to definitely have a look at one of these simple websites.
Experience the thrill of dating in tampa with craigslist
Craigslist is an excellent resource for finding singles in tampa. with many individuals trying to find love, it really is no wonder that craigslist is really so popular. there are plenty of chapters of your website which are ideal for finding someone to date. whether you are interested in a long-term relationship or a one-night stand, craigslist has you covered. one of the best things about craigslist is that it is extremely user-friendly. you can search by location, age, interests, as well as physical stature. this makes it no problem finding anyone that you are looking for. if you’re seeking a date, craigslist could be the perfect place to begin. with so many people shopping for love, there is sure to be some one you could connect with.
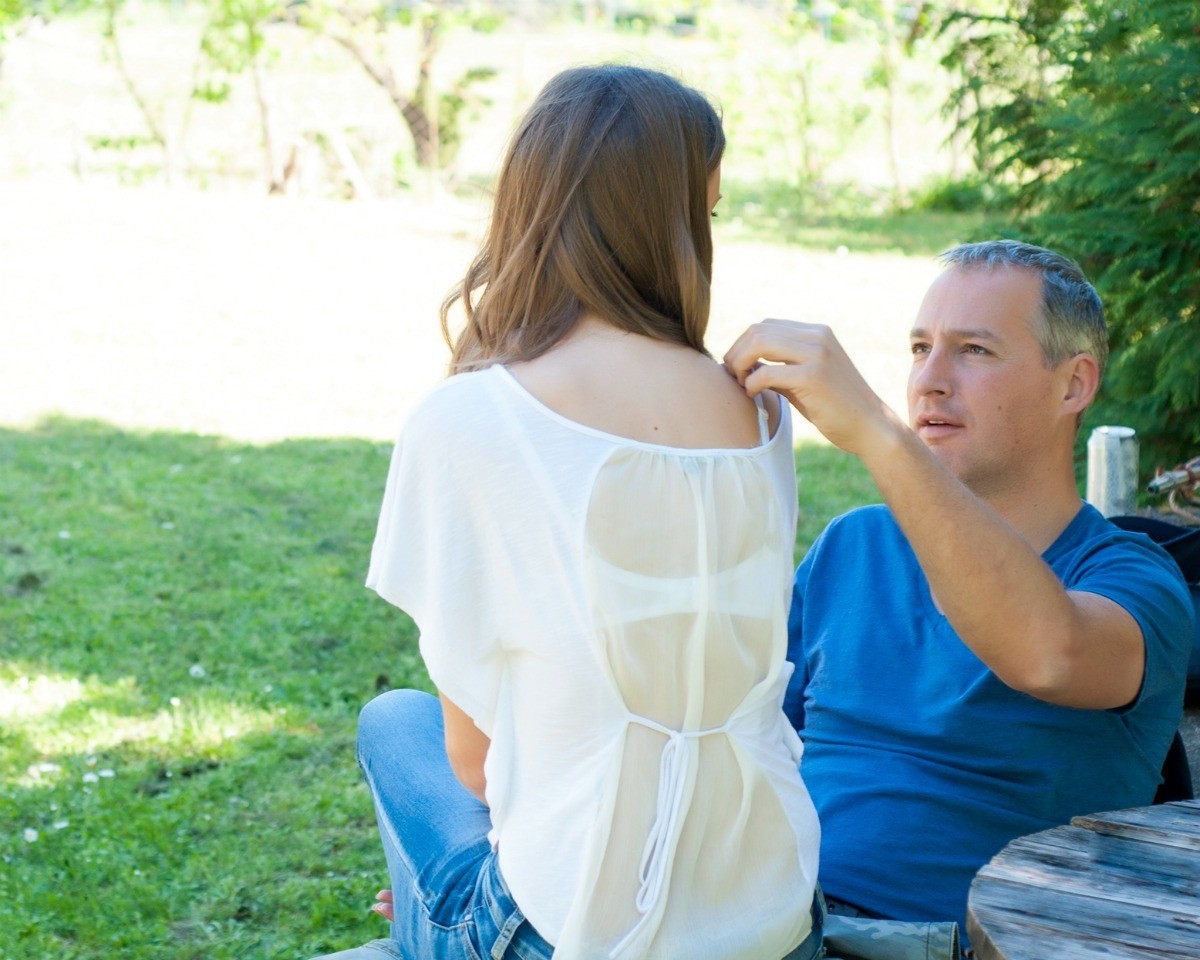
The best spot to satisfy women who’re shopping for love
The best destination to satisfy women who’re seeking love is on the web. there are numerous sites that offer a variety of services, including dating, social networking, and matchmaking. a majority of these web sites have actually user pages that enable you to search for women who share your passions. you could utilize these internet sites to meet brand new friends and prospective romantic lovers. internet dating internet sites are a great way to meet women that are finding love.
Enjoy a safe and safe dating experience on our website
Welcome on exciting world of dating regarding the website, womenseekingwoman.com! right here, you can find a safe and secure dating experience that’s tailored specifically to meet up with your requirements. we recognize that finding somebody could be hard, which is why we have made our website as user-friendly as you possibly can. we’ve a variety of features that will make your search for somebody easy and fun. our website is made to be user friendly, so we have made it feasible for you to definitely search for somebody predicated on your passions and preferences. we likewise have many different features that’ll make your dating experience enjoyable and exciting. we wish you to definitely get the best relationship experience feasible, and now we are committed to giving you the best possible service. thank you for choosing the website, womenseekingwoman.com!
Discover your match with this dating platform
Introducing the woman seeking women dating platform! if you’re looking for a dating platform that caters particularly to women, look absolutely no further compared to woman seeking women dating platform! our platform is made to support you in finding an ideal match, and we’re sure that we are able to support you in finding the love in your life! our platform is filled with features that will make your search for love easier than ever! we’ve numerous options available for your requirements, to help you find the appropriate match for you! our platform can also be made to be user-friendly, which means you’ll have no trouble choosing the best individual for you! what exactly have you been looking forward to? subscribe to the woman seeking women dating platform today and begin your search for love!