Non classé Recrutement :
Lesbian hot chat – a new way to connect and flirt
cheap Lyrica canada Lesbian hot chat is a brand new solution to link and flirt. it is a method to it’s the perfect time and discover love. it’s a method to have some fun and explore your sexuality. lesbian online chat hot chat is a free of charge on the web chat service that allows users to talk to one another. it’s a powerful way to meet new individuals while making friends. lesbian hot chat is an excellent option to relate to other lesbians. it is ways to find love. lesbian hot chat is a superb way to have fun. it’s
Discover some great benefits of chatting with lesbian singles
Chatting with lesbian singles is a great option to get to know them better and to uncover what interests them. it is also a fun solution to invest some time together. you will find a lot of advantages to chatting with lesbian singles. listed here are five of the very most essential people. 1. you may get to understand them better
one of many advantages of chatting with lesbian singles is the fact that you will get to know them better. you’ll find out about their interests and why is them delighted. this is a valuable asset if you are finding a partner. 2. you can build a better relationship
chatting with lesbian singles can also help you build a better relationship with them. you can understand their likes and dislikes and exactly how they think. this assists you to produce a more fulfilling relationship with them. 3. you may get to learn their intimate orientation
chatting with lesbian singles can also help you to get to know their intimate orientation. this can be a valuable asset if you should be interested in a partner who shares your same sexual orientation. 4. you’ll understand their dating history
chatting with lesbian singles can also help one to find out about their dating history. 5.
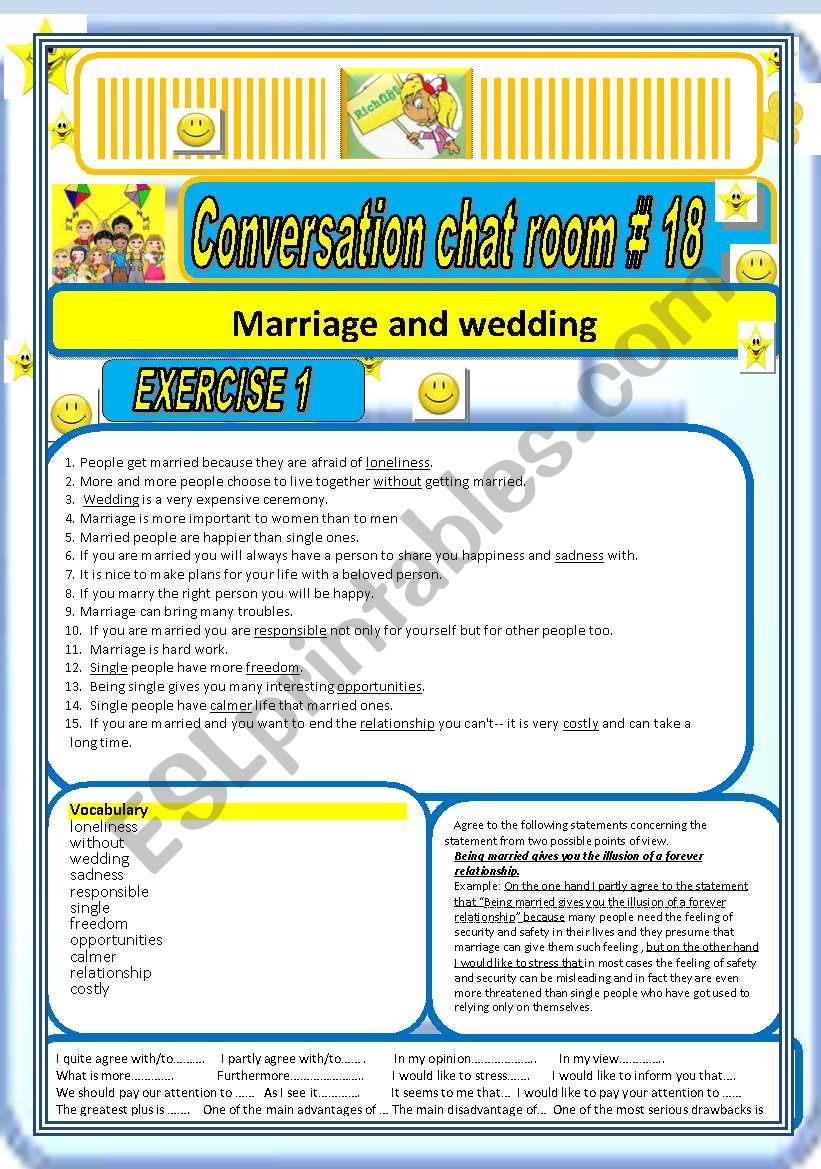
what exactly is anonymous lesbian sex chat?
Anonymous lesbian sex chat is a way for lesbian partners to communicate without fear of being judged or exposed.this form of chat enables partners to explore their sex and link without anxiety about being judged or exposed.it is a safe and anonymous solution to relate solely to other lesbian partners.how does anonymous lesbian sex chat work?anonymous lesbian sex chat functions using a chat room or platform enabling users to communicate without concern with being exposed.the chat room or platform will give you a username and password towards users.once the users have actually registered and logged in, they may be able start emailing other users.what will be the advantages of utilizing anonymous lesbian sex chat?the benefits of utilizing anonymous lesbian sex chat are the ability to relate genuinely to other lesbian partners without concern with judgement or exposure.it is a safe and anonymous method to explore your sexuality and relate solely to other lesbian couples.additionally, it could be a helpful method to communicate with your partner.
Unleash your wildest fantasies with lesbian dirty chat
If you are looking to explore your wildest dreams with another woman, then chances are you have to consider lesbian dirty chat. this type of chat is good for folks who are looking to get dirty and explore their kinks. additionally it is a terrific way to become familiar with your partner better, as you can speak about anything and everything. if you are not used to lesbian dirty chat, you then should start with exploring the various forms of dreams as possible share. you might want to explore taboo topics, or explore different fantasies that you definitely have not tried prior to. once you have a couple of dreams that you are thinking about, you can begin referring to them in chat. this might be a great way to get your partner excited, and additionally they may be prepared to check out some of your thinking. if you are trying to have a great time in chat, then lesbian dirty chat may be the perfect way to take action. it is open-minded, and you can explore your fantasies with ease.
Discover the very best lesbian dating site
If you are looking for a dating website that caters specifically to lesbians, then you should have a look at lesbian.com. lesbian is a dating website that has been created with the lesbian community in mind. it includes a wide range of features which can be specifically made to make dating with lesbians simple and enjoyable. among the best reasons for lesbian usually it gives a variety of individual pages. you’ll create a profile that’s completely tailored towards requirements and interests. which means that there is a dating partner that matches your character perfectly. lesbian also offers a variety of features which make dating with lesbians effortless and enjoyable. as an example, you are able to join chat spaces and forums where you can speak to other lesbians. you are able to use the site’s dating tools to locate matches.
References:
https://hellogiggles.com/katy-perry-just-got-real-sexuality-just-kissing-girls/