Non classé Recrutement :
Take the jump in order to find horny online dating success
buy Lyrica from india If you are considering a method to spice up your sex life, then chances are you must look into looking at horny online dating. this type of dating is perfect for individuals who are looking for a more discreet way to have sex. you can find those who are interested in equivalent things as you, and you also don’t have to concern yourself with embarrassing yourself in front of some one that you do not understand. one of the better reasons for horny online dating is it really is anonymous. which means you can be your self, therefore do not have to worry about some one judging you. you can even fulfill people from all over the world, that is great if you are searching for a brand new adventure. if you’re ready to make the leap and take to horny online dating, there are some items that you must know. first, make sure that you are more comfortable with the thought of being naked before some one. if you can respond to these questions, then you’re willing to begin your journey into horny online dating.
Enjoy discreet and secure horny adult dating with this matchmaking service
If you are looking for a way to have a great time and move on to know new people, then horny adult dating is the strategy to use. with your solution, you’ll find singles that in the same way thinking about getting down and dirty while. plus, our matchmaking system is made to assist you in finding the right match, to help you enjoy discreet and safe dating. why not give horny adult dating a try today? you will not be sorry!
what to anticipate from horny online dating?
What to expect from horny online dating
if you are searching for a little excitement in your life, horny online dating may be the ideal solution. this kind of dating site is made for people that are looking for a sexual relationship. you’ll find all sorts of individuals on horny online dating web sites, from singles whom would like to have a blast, to couples who’re seeking a little extra spice in their lives. when you’re on a horny online dating website, you ought to be prepared to encounter a lot of people that are selecting the same thing you are. meaning that you will be capable of finding somebody who works with with you and who you might have a very good time with. needless to say, you should also be ready for the chance that you won’t find anyone who you wish to date. this is exactly why it is vital to be open-minded also to not let your expectations have excessive. if you’re not comfortable because of the notion of dating an individual who is looking for a sexual relationship, then chances are you should probably steer clear of horny online dating websites. general, horny online dating is a superb strategy for finding someone who works with you and whom you can have a great time with. just be prepared the possibility you wont find anyone that you need to date straight away, and start to become prepared to experiment only a little bit.
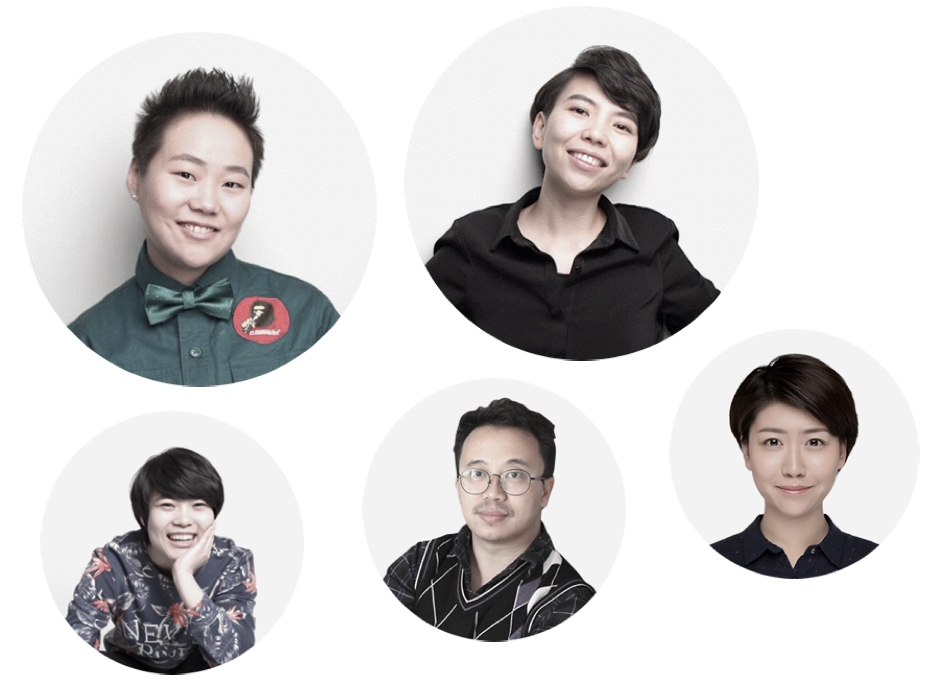
Discover horny online dating – the easiest way to locate love
Online dating has become a favorite strategy for finding somebody, and horny online dating is the perfect strategy for finding an individual who works with with you. horny online dating is straightforward and easy to utilize, and it is a terrific way to fulfill new individuals. horny online dating is a good strategy for finding an individual who works with you. horny online dating is easy and easy
What are horny women dating sites?
Horny women dating sites are internet sites designed to help lonely women find partners.sites like these offer a variety of features, including the capacity to look for singles by location, age, and passions.they additionally often offer forums and discussion boards in which users can keep in touch with both.some of the most extremely popular horny women dating sites consist of datehookup, eharmony, and match.com.these sites offer many different features, like the capability to look for singles by location, age, and interests.they also often provide boards and discussion boards where users can talk to both.horny women dating sites are a great way to fulfill brand new individuals.they can also be a powerful way to find a partner.sites like these offer a variety of features, including the capacity to search for singles by location, age, and interests.they also usually provide forums and discussion boards in which users can keep in touch with each other.
Get started now in order to find your perfect horny adult dating match
Adult dating is an excellent option to meet horny new individuals and explore your sex. with all the right person, it could be a remarkably fun and satisfying experience. however, it could be difficult to find the right match. that is where horny adult dating is available in. with horny adult dating, you can find a person who works with your intimate desires and interests. this can be a terrific way to explore your sexuality and discover new partners. there are numerous of facts to consider when searching for a horny adult dating match. first, you should consider your interests and desires. this can assist you in finding somebody who works with you. 2nd, you ought to try to find a person who can be compatible with your chosen lifestyle. this means that they need to have similar passions and then enjoy the exact same items that you do. which means that they must be good match available regarding character and life style. with horny adult dating, there is the perfect partner for you personally.
