Non classé Recrutement :
Get connected in order to find your match for sex tonight
http://rodneymills.com/2q6ji/preston-athletics-track.html Dating apps for sex are a terrific way to relate genuinely to people who might be thinking about having sex. with so many options available, it could be hard to decide which software is right for you. listed below are five of the greatest dating apps for sex. 1. hornet
hornet the most popular dating apps for sex. it has an array of features, including a messaging system and a number of filters that make it easy to find those who are enthusiastic about sex. 2. tinder
tinder the most popular dating apps for sex because it is easy to use. there is folks who are thinking about sex by swiping left or appropriate. 3. grindr
grindr is a popular dating application for gay and bisexual individuals. it’s especially popular for folks who are searching for sex. 4. okcupid
okcupid is one of the earliest dating apps and is popular for people that are looking for a relationship. it has an array of features, including a messaging system and a number of filters. 5. hornet plus
hornet plus is a premium version of hornet. this has many features which make it better than the standard hornet.
Find the best sex dating sites of 2016
Best sex dating sites of 2016 selecting a powerful way to enhance your sex life? discover a number of the best sex dating sites of 2016! there is a large number of great options available to you about finding a sex partner, that dating sites are certain to have something for everyone. sexdating whether you’re looking for a casual encounter or something much more serious, these sites could have you covered. 1. adultfriendfinder adultfriendfinder is one of the most popular sex dating sites in the world, and for good reason. it’s an array of features, including the search engines that will find matches predicated on location, interests, and also particular tasks. 2. match.com match.com is another popular site for finding sex partners. 3. craigslist craigslist is a great spot to find sex partners if you are wanting something casual. it offers an array of groups, including » casual encounters, » » sex personals, » and » personals. » 4. eharmony eharmony is a great site if you are shopping for a critical relationship. 5. nsfw nsfw is a great website if you are selecting something a little more risqué. it’s an array of categories, including » sex and dating, » » kinky sex, » and » fetish and bdsm. »
Connect with like-minded women regarding best dating site
If you’re looking for a dating site that caters especially to bisexual women, then chances are you’re in luck. there are numerous of good choices on the market, and each one offers a unique unique group of advantages. among the best dating sites for bisexual women is bisexual.com. this site is designed particularly for bisexual singles, also it provides many different features that make it a great choice for those searching for a dating site that caters specifically to bisexual women. very important top features of bisexual.com is its myspace and facebook. this system was created to assist bisexual women relate genuinely to the other person and share information and advice. in addition provides a great many other features, particularly a forum and a chat room. this site provides many features which make it an ideal choice for those looking for a dating site that caters especially to bisexual women. this algorithm is made to assist bisexual women find matches which are an excellent fit for them.
How to choose the right sex adult dating site for you
When it comes down to dating, there are plenty ofoptions available. whether you are considering a casual fling or something more serious, there’s a niche site out there available. but what type is the better for you personally? to find the right website, you need to decide everything’re looking for. do you wish to date people in your town, or have you been open to dating individuals from all around the globe? would you like to chat with people or do you wish to receive and send messages? once you have your requirements set, you will need to consider the features of the different sites. some sites provide more features than the others, plus some offer features for different types of users. once you have a good idea of everything you’re looking for, it’s time to consider the various sites. check out tips to help you choose the best one for you:
1. do your research
before you decide to even begin looking at sites, you ought to do a little research. this will allow you to figure out which sites would be the perfect for you. consider the reviews and ratings of this different sites, and find out about the features and services they feature. 2. think about the size associated with community
perhaps one of the most key elements to consider may be the size associated with the community. some sites have an inferior community, while others have actually a more substantial community. this is really important because it will regulate how active your website is and how most likely you might be to locate someone you find attractive. 3. it’s important to find a niche site that is affordable and will be offering the features you will need. 4. 5. once you have most of these factors in your mind, it is time to start looking at various sites. some sites are more
Start exploring now and find your perfect partner
Dating apps are a powerful way to fulfill new individuals and explore various passions. they can be a terrific way to find some body with who you might have sex. there are a number of various dating apps available, and each features its own features and benefits. if you’re looking for a method to explore your sexual interests and find new lovers, contemplate using a dating application. here are five of the finest dating apps for sex:
1. tinder: tinder is a dating app which popular for its quick and easy search function. you can search by location, age, and passions. you’ll be able to flick through the pages of people who have actually matched with you. 2. hornet: hornet is a dating app which dedicated to finding long-lasting relationships. you are able to browse through profiles and send communications to people you’re interested in. 3. grindr: grindr is a dating app that is centered on finding intimate lovers. 4. 5. coffee satisfies bagel: coffee suits bagel is a dating software which dedicated to finding intimate partners.
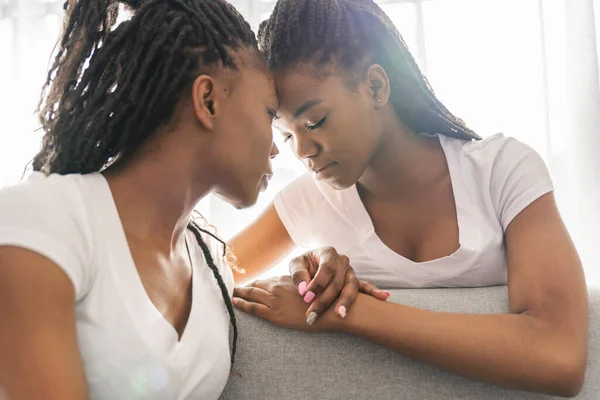
Get prepared to enhance your love life with the best dating sites of 2016
If you’re looking for a way to add spice to your love life, you should look at utilizing one of many best sex dating sites of 2016. these sites offer a variety of features that may make your dating experience more enjoyable. among the best features of these sites may be the selection of users. you can actually find someone who shares your interests and desires. plus, the sites are made to be user-friendly, which means you’ll don’t have any trouble finding a romantic date. another great function of the sites is that they offer many different dating options. you’ll find a person who is simply right for you utilizing the site’s search function. there is someone who shares your passions and desires, as well as features that can make your dating experience more fun.