Non classé Recrutement :
Meet lesbian singles within area
bluely Looking for ways to fulfill other lesbian singles in your area? look no further versus local lesbian hookup chat rooms chat forum! this network is a good solution to interact with other lesbian singles to see about local events and meetups. you may inquire and acquire advice off their people. plus, if you should be selecting someplace in order to make some new friends, the local lesbian hookup chat forum is the perfect place to start.
Benefits of lesbian hookup chat
There are many benefits to engaging in lesbian hookup chat. not only does it offer a safe and comfortable room for lesbians to connect, but it can also help to construct relationships and increase interaction. one of the most important advantages of lesbian hookup chat is it can benefit to build trust. when lesbians are able to communicate freely and actually, they’re almost certainly going to build trust and trust is key to a healthier relationship. in addition, lesbian hookup chat can help increase interaction and understanding between partners. when partners can talk about their feelings and issues, they can build a stronger relationship. finally, lesbian hookup chat can help build camaraderie and help between lesbian partners. whenever partners have the ability to support and encourage both, they are more prone to thrive within their relationships.
Enjoy a secure and safe on the web environment
Online relationship became ever more popular lately, and for valid reason. it provides a safe and secure on the web environment where singles can relate genuinely to others who share similar interests. but like most on the web platform, there are risks connected with making use of online dating. one of the most typical dangers is meeting some body yourlove on line and having that relationship falter. one good way to reduce steadily the risk of meeting someone yourlove on the web and then having that relationship fall apart is by using local lesbian hookup chat. in this manner, you will be certain that yourlove is actually in the same city as you are. furthermore, local lesbian hookup chat provides a safe and safe environment in which singles can talk about any problems that they might have. this way, you can be sure yourlove is truly interested in you and not just in search of a one-night stand. finally, local lesbian hookup chat is a great method to fulfill brand new individuals. general, utilizing local lesbian hookup chat is an excellent way to reduce the risk of meeting somebody yourlove on line then having that relationship fall apart.
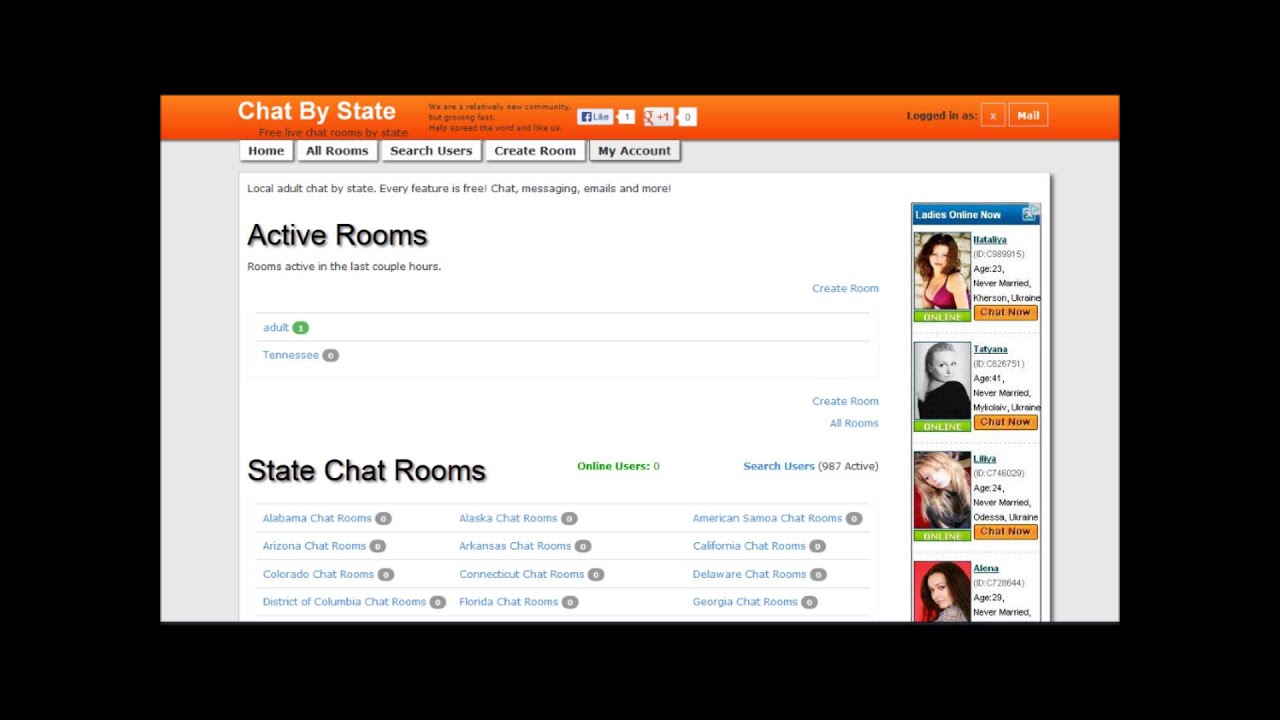
What makes a great online hookup chat?
there are some key items that alllow for a fantastic online hookup chat.first and most important, it needs to be user friendly.users should be able to sign in and begin chatting without any difficulty.second, the chat ought to be interactive and engaging.it should really be a spot where users can certainly connect and possess fun.finally, the chat must be protected and private.users can feel safe and secure whenever chatting online, without anxiety about being exposed or ashamed.all of the facets are very important to make outstanding online hookup chat.if a chat is straightforward to use, interactive, and safe, it’s going to be more likely that users will want to use it.it may also be much more likely that they’ll find success within their online dating experiences.
Take the next step and begin your online hookup chat journey now
If you are looking to start your online hookup chat journey, there are a few things you must do first. first, you will need to find a chat room that is correct available. there is a room that’s perfect for you by using the search club regarding the right side associated with the page. once you find a space that you are thinking about, you will need to register for an account. to register for a free account, you need to provide your name, email address, and a password. once you verify your email address, you could begin chatting in the chat room. first, you should know how to begin a conversation. to start a conversation, you have to be in a position to explore such a thing. you could begin a conversation by asking a question in regards to the other person’s life. it is possible to begin a conversation by discussing the elements. if you’re experiencing adventurous, you may begin a conversation by discussing sex.
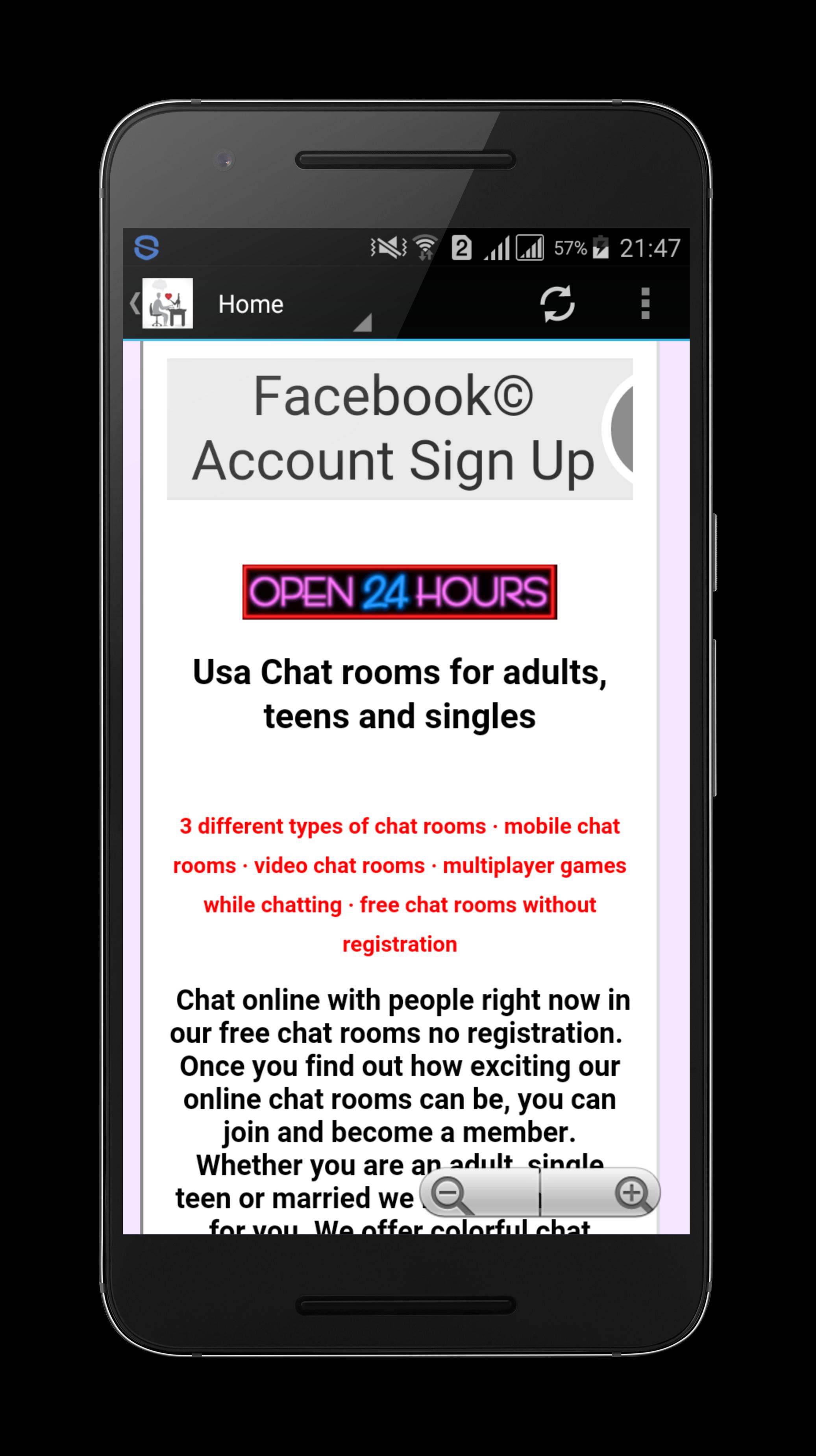
References:
https://www.baltimoresun.com/chi-online-dating-age-wars-tinder-eharmony-20150406-story.html