Non classé Recrutement :
Ready to begin your these couples hookup hookup adventure with a woman?
where to buy real accutane online in that case, you are in fortune!there are a good amount of ladies on the market that are looking for a new adventure, and you also’re simply the person for the task.first, you will need to make certain you’re both enthusiastic about this kind of relationship.if you are not sure, ask your potential romantic partner if she’s enthusiastic about checking out this type of relationship.if she actually is open to the theory, then you’re ready to begin looking for couples hookups.once you’ve verified that you both are interested, you’ll need to start thinking about what kind of couples hookups you want to engage in.there are a lot of different choices available to you, so it’s important to select a thing that you both will enjoy.if you are looking for something romantic, you can test going on a night out together.this could be a great solution to become familiar with both better to discover if you have any typical interests.if you’re looking for one thing more physical, you can try happening per night out along with your partner.this are a fun method to get some fun and excitement inside relationship.whatever you select, ensure that you’re both happy with it.if certainly one of you isn’t delighted, it won’t be worth every penny to keep the connection.so ensure that you both have fun and that you are both enjoying yourselves.ready to begin your couples hookup adventure with a woman?if therefore, you are in fortune!there are a great amount of ladies around that looking for a new adventure, and you’re just the person for the task.first, you’ll need to ensure that you’re both interested in this type of relationship.if you aren’t certain, pose a question to your potential romantic partner if she actually is interested in checking out this kind of relationship.if she actually is ready to accept the idea, then chances are you’re prepared to begin looking for couples hookups.once you’ve verified that you both have an interest, you will have to begin thinking about what kind of couples hookups you intend to engage in.there are lots of different options accessible to you, so it’s crucial that you choose a thing that both of you will love.if you’re looking for one thing intimate, you can test going on a romantic date.this are an enjoyable option to get acquainted with both better to see for those who have any typical passions.if you are looking for something more real, you can look at happening a night out together with your partner.this is a fun method to get some fun and excitement inside relationship.whatever you decide on, ensure that you’re both pleased with it.if among you isn’t happy, it’s not going to be worth it to continue the connection.so ensure that you both have fun which you’re both enjoying yourselves.
Find love and enjoyable with couples hookup site
Looking for love and fun? search no further compared to the best couples hookup site online! this site offers singles the opportunity to find love and have now enjoyable with other couples. couples hookup site is a good method to fulfill brand new individuals and also some fun. if you’re searching for a way to enhance your dating life, couples hookup site may be the perfect site available! this site offers singles the chance to meet new individuals and possess some lighter moments. you’ll fulfill brand new folks from all over the globe and have now some lighter moments together. there are a lot of couples hookup site users who have had plenty of success by using this site.
Find your perfect couple hookup site now
Finding your perfect few hookup site could be a daunting task. with so many solutions, it can be difficult to know the place to start. the good news is, we have compiled a listing of the greatest few hookup websites available today. if you’re selecting a site that caters particularly to couples, then browse couple hookup. this site is filled with features which will make your relationship experience easier. from a user-friendly software to a wide range of features, couple hookup has everything you need to find your perfect match. if you are seeking a site that gives a far more basic selection of dating choices, then take a look at cupid. this site offers many features, including a user-friendly screen and a range of dating categories. whether you are looking for an informal date or a serious relationship, cupid has you covered.
How to get the right couples hookup for you
When it comes down to locating a couples hookup, there are some things you’ll want to remember. first and foremost, you’ll want to be sure that the individual you might be starting up with works with with you. next, you’ll want to find somebody who is willing to have an informal, no-strings-attached relationship. and finally, you will need to make sure that the location and time of one’s hookup are compatible with both of one’s schedules. about compatibility, it is critical to be honest along with your prospective hookup. if you’re perhaps not compatible with them, it is advisable to just move ahead. likewise, in case the possible hookup is not appropriate for the place or time of one’s hookup, be truthful about this and. when you can both consent to move forward, then do so. however, if you fail to agree to those terms, it is far better proceed. about finding a place and time for your hookup, it is vital to think about your requirements and wishes. would you like to attach at a bar or club? or would you like to go someplace more private? additionally it is important to think about the period. do you want to connect each day, afternoon, or night? and lastly, do you wish to connect in a group or solo? once you have chosen the positioning and time of the hookup, it is time to think about your hookup partner. do you wish to attach with somebody brand new, or do you want to hook up with some body you know? and lastly, do you want to hook up with someone who is single or someone who is in a relationship? do you wish to have sex, have sexual intercourse, or perhaps fool around? and finally, do you wish to connect in a public or personal location? once you have chosen every one of the details of your couples hookup, it is time to get ready. above all, ensure that you are both neat and free of any infections. secondly, make certain you have most of the necessary supplies, including condoms, lube, and adult sex toys. and lastly, make sure that you are comfortable with the hookup location and time.
Why choose couples hookups with?
Couples hookups with offer an original chance of couples to explore their sex and intimacy in a safe and comfortable environment. they offer a means for couples to get in touch and share their feelings with no stress of a conventional relationship. couples hookups with also offer an opportunity to explore brand new sexual dreams and explore your lover’s intimate desires. there are a variety of reasoned explanations why couples should select couples hookups with. first, couples hookups with offer a means for couples to get in touch and share their emotions. 2nd, couples hookups with offer a safe and comfortable environment. they are designed to be personal and private, therefore you may be available and honest with each other without anxiety about judgment. couples hookups with additionally offer the possibility to experiment with different intimate jobs and sexual tasks. they offer an easy method for couples for connecting and share their emotions, which can help to strengthen the partnership. this can help to boost the partnership by deepening the bond between both you and your partner.
Benefits of couples hookup
There are benefits to couples hookup. a few of the advantages consist of:
1. increased intimate satisfaction
one of the most significant benefits of couples hookup is that it may increase intimate satisfaction. it is because permits couples to explore their sex many to locate brand new and exciting methods to have sexual intercourse. this can trigger increased pleasure for both lovers. 2. increased relationship satisfaction
couples hookup also can trigger increased relationship satisfaction. this could induce increased closeness and closeness into the relationship. 3. greater communication
couples hookup can also cause greater communication. the reason being it allows couples to start up about their feelings also to communicate better. this could lead to increased trust and understanding between the partners. 4. the reason being it may produce an even more exciting and stimulating atmosphere within the bedroom. this could easily trigger increased sexual intercourse and more intense intimate experiences. 5. increased trust
couples hookup may also result in increased trust. the reason being it could produce a more trusting and available relationship. if you are looking to raise your intimate satisfaction, relationship satisfaction, communication, and trust, couples hookup will be the perfect solution for you personally.
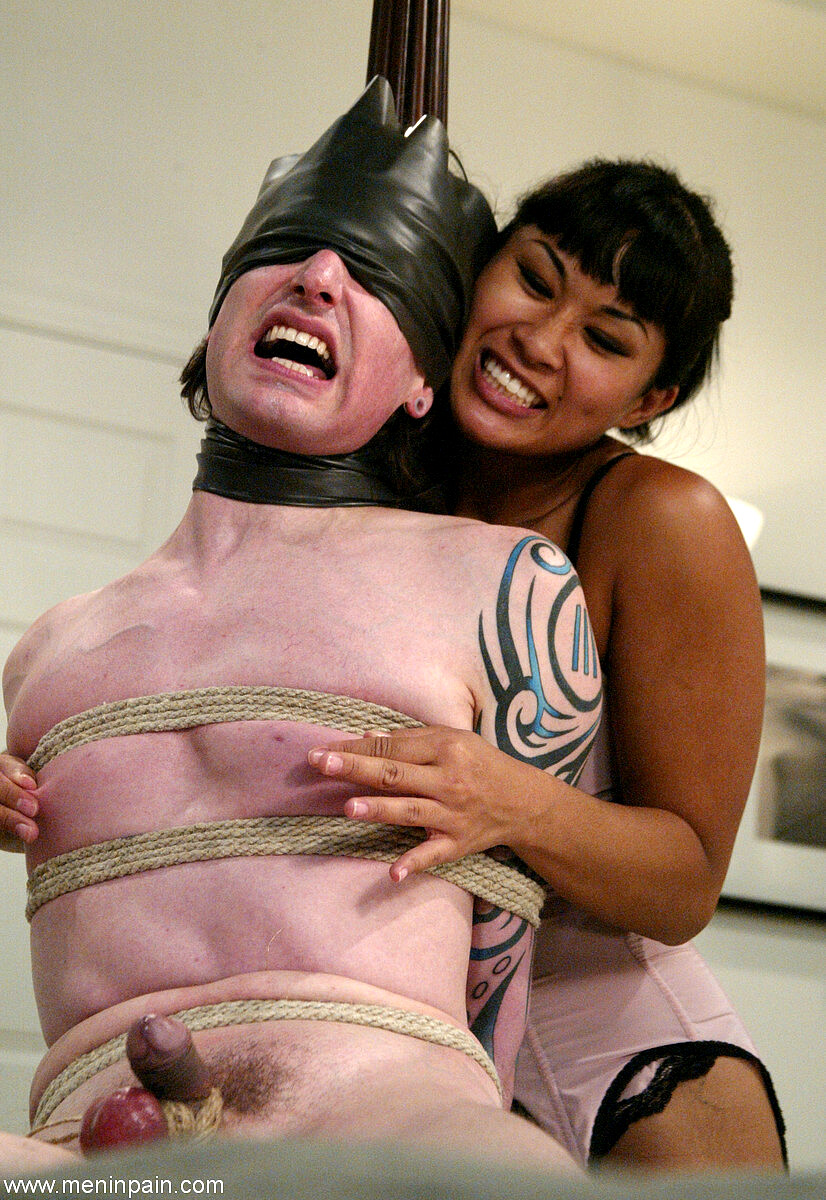
Experience the excitement of a woman looking for couples hookups today
If you are looking for something new and exciting in your life, you then should truly think about testing out the excitement of a woman looking for couples hookups.this type of dating can be quite fulfilling, and it can provide you with lots of fun and excitement.if you have in mind attempting this down, then chances are you should truly give consideration to doing so now.there are countless advantageous assets to dating a woman looking for couples hookups.for one, you’ll be able to experience some new and exciting things.this variety of dating can be very stimulating, and it will help you to explore your sex in a fresh means.additionally, you can fulfill new people making brand new connections.this could be a very valuable experience, and it will help you to expand your network and build brand new relationships.if you have in mind trying out this kind of dating, then chances are you should truly do so now.it are a lot of fun, and it will provide you with plenty of new and exciting experiences.