Non classé Recrutement :
Benefits of a threesome hookup
http://midequalitygroup.co.uk/events/2021-06-23/ There are benefits to having a threesome hookup. first of all, it can be a lot of fun. if you are both interested in each other, it could be a terrific way to explore your sexual boundaries and have now some brand new and exciting experiences. it can also be a method to add spice to your sex-life. if you are solitary and seeking for a few brand new excitement in your lifetime, a threesome hookup could be the perfect solution. another good thing about a threesome hookup is it may be a method to conquer a breakup. if you’re solitary as well as your ex continues to be on your mind, a threesome hookup could be a powerful way to overcome him. it could be ways to explore your sex while having some brand new and exciting experiences with another person. finally, a threesome hookup could be a method to enhance your self-confidence. if you are feeling down about your dating life or your sex life, a threesome hookup could be a powerful way to boost your self-confidence.
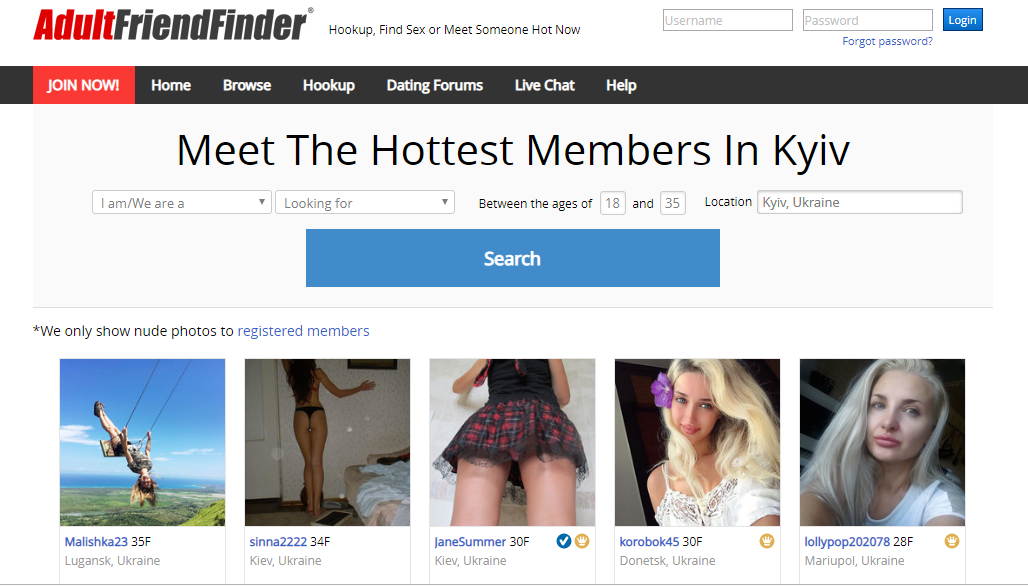
How to obtain the perfect threesome hookup
Finding a threesome could https://hookupndate.com/threesome-hookups.html be a lot of fun, but it can also be some tricky. here are some tips on how to find the perfect threesome hookup. 1. try to find sets of like-minded individuals. the easiest method to find a threesome is to try to find sets of people that are thinking about exactly the same things. which means that you need to be open-minded and willing to take to new things. 2. talk to your buddies. one of the better how to find a threesome is to confer with your buddies. when they understand of any groups or individuals who are wanting a threesome, they may be able to point you in right way. 3. join online dating services. another good way to locate a threesome would be to join online dating services. in this way, you can search for those who have been in the same area as you are. 4. try to find groups on social media marketing. one final strategy for finding a threesome is search for groups on social media marketing. this really is a terrific way to find people that are interested in the same things while.
Ready to begin your threesome hookup adventure?
If you’re interested in checking out the planet of threesomes, there are many things you must know first.first, it is important to be open on idea.if you’re not comfortable with the notion of sharing your spouse with someone, you are not planning to have a good time.second, be sure you have a notable idea of what you’re looking for.are you interested in an informal hookup with somebody brand new, or are you searching for one thing more serious?third, expect you’ll involve some fun.threesomes are about enjoying yourself, assuming you are not ready to have a great time, you aren’t going to have fun.so, isn’t it time to start your threesome hookup adventure?here are a few tips to help you get started.1.make sure you have got recommended of what youare looking for.if you are looking for a casual hookup with someone new, you’re going to wish to look for somebody who is available to the idea.on others hand, if you are in search of something more serious, you’re going to want to look for someone who works with.if you’re not yes everything you’re looking for, it is best to communicate with someone who has experience with threesomes.2.be prepared to involve some fun.if you are looking for a serious threesome hookup, you will need to be prepared to involve some fun.if you are not prepared to have a blast, you are not planning to have a good time.make sure you are confident with the idea of having sex with two others, making yes you’re both confident with the thought of sex with you.3.make certain you are available to the idea of sharing your partner.if you’re not open to the notion of sharing your lover, you are not going to have a good time.on another hand, if you’re available to the thought of sharing your lover, be sure you’re willing to do so.make certain you have got a definite agreement about who is likely to do what, and make yes you’re both more comfortable with the arrangement.ready to start out your threesome hookup adventure?if you are available to the idea of exploring the entire world of threesomes, there are some things you need to know first.first, you need to most probably to your idea.if you are not more comfortable with the thought of sharing your partner with someone, you’re not planning to have a good time.second, be sure you have a good idea of everythingare looking for.are you interested in a casual hookup with some one brand new, or are you searching for something more serious?third, anticipate to involve some fun.threesomes are all about having a good time, and in case you’re not willing to have a great time, you aren’t gonna celebrate.so, are you ready to start out your threesome hookup adventure?here are a couple of suggestions to help you to get started.1.make yes you have advisable of what youare looking for.if you are considering a casual hookup with some body brand new, you’re going to wish to search for a person who is ready to accept the theory.on one other hand, if you are in search of something much more serious, you will wish to try to find a person who is compatible.if you aren’t sure everything youare looking for, you need to keep in touch with anyone who has experience with threesomes.2.be willing to have some fun.if you are looking for a significant threesome hookup, you will should be prepared to have some fun.if you are not ready to have some fun, you aren’t planning to celebrate.make sure you’re more comfortable with the idea of making love with two other folks, while making certain you are both more comfortable with the thought of having sex with you.3.make sure you’re available to the notion of sharing your partner.if you’re not open to the thought of sharing your partner, you’re not going to celebrate.on others hand, if you should be available to the thought of sharing your partner, make sure you’re ready to do so.make certain you have got a clear agreement about who’s gonna do what, and also make certain you are both confident with the arrangement.ready to start out your threesome hookup adventure?if you’re available to the idea of exploring the world of threesomes, there are a few things you must know first.first, it is critical to be open to the concept.if you are not comfortable with the notion of sharing your partner with another person, you’re not going to celebrate.second, make sure you have a notable idea of that which youare looking for.are you trying to find an informal hookup with some one brand new, or are you searching for one thing much more serious?third, anticipate to involve some fun.threesomes are about having fun, and in case you aren’t willing to have some fun, you aren’t likely to celebrate.so,
Find your perfect threesome hookup app
Finding an ideal threesome hookup app may be hard, but with the proper app, you could have some fun and explore your kinks with some one brand new. here are a few of the finest threesome hookup apps available today. 1. threesome.com
this app is perfect for those who are in search of a threesome with some one brand new. you can flick through profiles of people that are searching for a threesome, and then you could start a conversation with one of many members. you’ll be able to content users and set up a period for a meeting. 2. threesome.co.uk
this app resembles threesome.com, however it is specifically for people in the united kingdom. 3. 4. 5.
Get began now and find your perfect threesome hookup site
If you’re looking for a threesome hookup site, you’re in fortune! there are a lot of great choices available to you, and you may find one which’s ideal for you. first, it’s also important to consider what variety of threesome hookup site you want. there are traditional hookup web sites, where you meet individuals personally and hook up, after which you will find social media-based threesome hookup websites, and you’ll discover people through social media. whichever type of site you decide on, make sure to read the reviews first. this may assist you in finding a site that has been well-rated by other users. once you have discovered a site that you are enthusiastic about, the next step is to register. this may offer you use of the site’s features and invite you to definitely begin browsing for prospective partners. once you’ve registered, the next thing is to start out searching the profiles of individuals regarding site. this is how you’ll find your possible partners. once you’ve found a potential partner, the next phase is to meet up. this is done in person, or through other form of interaction. once you have met up, the next thing is to attach. this is done in many ways, and it is your decision plus partner. if you should be searching for a threesome hookup site that is safe and secure, then you’ll be wanting to look at some of the safety features that the site offers. overall, threesome hookup internet sites are a great way to find a partner and connect with someone brand new. if you are willing to get going, make sure to check out one of the many great options on the market.