Non classé Recrutement :
Enjoy the benefits of our revolutionary dating app
isotretinoin no perscription required If you are considering a dating app that caters particularly to your lesbian community, you need to discover latina lesbian dating app. this app is made to assist lesbian singles relate solely to each other and explore their dating choices. latina lesbian dating app is a free app that is available on both android and ios products. the app has a user-friendly software and is simple to use. one of many benefits of utilizing latina lesbian dating app is it is an extremely diverse app. this means it will be possible discover singles who share your ethnicity, culture, and life style. this means it will be possible to locate singles who are trying to find a relationship or an informal dating relationship. overall, latina lesbian dating app is a superb app which built to assist lesbian singles relate with each other.
Find true love – christian lesbian dating source
Finding real love – christian lesbian dating source if you should be looking for ways to find true love, you need to check out christian lesbian dating internet sites. these websites will allow you to interact with other lesbian christians that wanting a significant relationship. there are a lot of great christian lesbian dating sites on the market. you will find web sites which are specialized in linking christians from all walks of life, including those people who are single, those people who are in a relationship, and the ones who’re married. if you are trying to find a site that’s created specifically for christian lesbians, you need to browse christian lesbian dating website. this website is specifically designed to get in touch christian lesbians along with other christian lesbians. https://lesbiandatingsite.net/lesbian-dating-sites-over-50.html there are additionally websites which are specifically designed for christian singles. these websites will allow you to find someone whom shares your spiritual philosophy. if you’re wanting a niche site that’s ready to accept all types of relationships, you need to consider internet sites that are focused on connecting christians with christians, christians with non-christians, and non-christians with christians.

Join the largest online community for lesbians
Looking for a place to connect with other lesbians? search no further than her lesbian dating site! this network is the biggest and a lot of comprehensive resource for lesbian singles on the planet. with over two million people, her lesbian dating site could be the perfect spot to find love. whether you are considering a long-term relationship or perhaps an informal talk, her lesbian dating site has all you need. plus, the community is incredibly friendly and inviting, and that means you’ll never feel alone. what exactly are you currently looking forward to? sign up today and commence searching the pages regarding the amazing women on her lesbian dating site!
Find love with her lesbian dating site
Looking for love? browse her lesbian dating site! her lesbian dating site is perfect for singles finding an even more intimate connection. with a person base of over 2 million people, you’re sure to find somebody who shares your interests and desires. plus, the site is filled with features making it simple to relate with other members. whether you’re looking for an informal date or something like that more severe, her lesbian dating site is the perfect starting point your search.
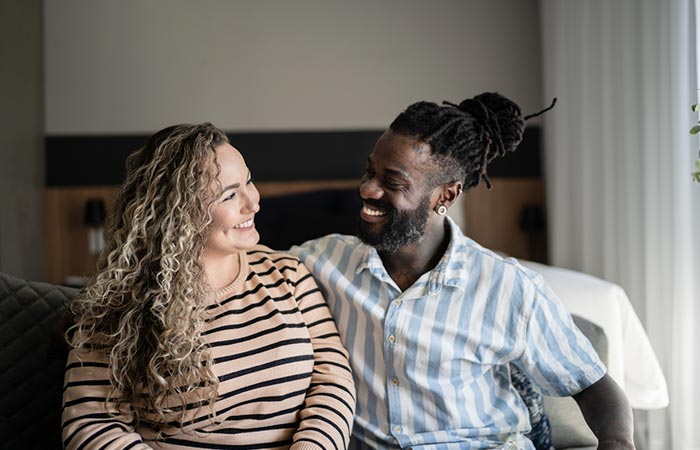
Discover the most effective platforms in order to connect with like-minded women
Finding christian lesbian dating sources
dating could be a difficult task, nonetheless it can be made much easier knowing where you should look. if you are trying to find a christian lesbian dating source, you are in fortune. there are numerous of platforms available that can help you relate genuinely to like-minded ladies. among the best platforms to use is christianmingle. this web site is designed designed for christian lesbians and bisexual women. it’s many features, like the ability to produce a profile, deliver communications, and meet other users. another great option is theladders. if you’re interested in a far more casual dating experience, discover grindr. this website is made for people that selecting casual sex. whatever your dating needs, there’s a platform available that can help you fulfill your match. therefore please explore the choices available and discover the best christian lesbian dating source available.
Meet amazing latina lesbians near you
Latina lesbians dating app is a great way to meet amazing latina lesbians in your area. with this particular app, you’ll relate solely to other latina lesbians and start dating. you can also find friends and meet brand new people. this app is ideal for people who are interested in a dating or relationship possibility. additionally it is great for people who are looking a brand new friend.