Non classé Recrutement :
Find your perfect sugar momma hookup today
http://snyderartdesign.com/author/admin/page/22/ Looking for a sugar momma hookup? you have come to the best place! finding a sugar momma hookup is lots of fun, and it can be a great way to get acquainted with some one brand new. plus, sugar mommas in many cases are really large, and so they provides some valuable advice and support. if you should be looking for a sugar momma hookup, you should start by utilising the online dating solutions. these services are high in sugar mommas that are looking brand new relationships. you could try contacting sugar mamas straight. many of them are content to satisfy brand new people, as well as could be ready to give you a sugar momma hookup. whatever route you decide on, be sure to be prepared. sugar mommas tend to be demanding, plus they may not be enthusiastic about dating someone who is not ready for a significant relationship. ensure that you’re prepared to commit, and that you’re willing to give the sugar momma hookup the possibility.
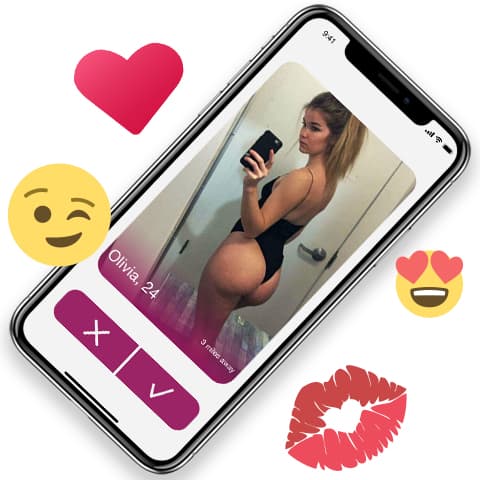
How to get the right sugar momma hookup for you
Finding the best sugar momma hookup available may be a daunting task. there are a great number of sugar mamas out there, and it can be hard to know what type is right for you. here are some tips on how to find the appropriate sugar momma hookup available: 1. start by doing some research. before you decide to even begin looking for a sugar momma hookup, you should do a little research. in this way, you will know what things to try to find. it is possible to go online or in publications for tips. 2. be honest with yourself. when register for dating site for milfs and sugar moms right here you are shopping for a sugar momma hookup, you ought to be truthful with your self. which means you should not lie to your self or even to the sugar momma. if you’re not sure if you’re ready for a sugar momma hookup, you then cannot proceed along with it. 3. be practical. which means you ought not expect every thing to be perfect. sometimes things cannot work-out, which is okay. aren’t getting upset if things do not get as in the pipeline. 4. have patience. which means you should not rush things. do not make any choices unless you have had the opportunity to think about it. 5. be respectful. its also wise to be respectful of the woman time. usually do not come over to the woman home too often or expect the lady to spend all the woman time with you.
How to get the right sugar momma for you
Finding a sugar momma hookup can be a disheartening task, but with some research, there is an ideal match for you. here are a few tips to help you find the best sugar momma available:
1. search for a sugar momma that is compatible with your chosen lifestyle. make sure that your sugar momma is somebody who you are able to enjoy hanging out with. she must be an individual who you can have fun with and whom you will enjoy hanging out with. if you’re looking a long-term relationship, make sure your sugar momma is someone who is compatible with your life style. 2. try to find a sugar momma who is financially stable. make sure your sugar momma is economically stable. she needs to have a stable task and enough cash to aid herself. if she’s perhaps not financially stable, she may not be in a position to provide you with the stability and security that you will be interested in in a sugar momma hookup. 3. she should certainly manage your emotional requirements and also offer you emotional help. 4. she needs a great body and be able to offer the real attraction that you’re searching for in a sugar momma hookup. 5. she should be able to hold a conversation and then think on her behalf foot. 6. she will be able to have fun and be able to cause you to laugh. if she’s not enjoyable, may very well not be able to have a good time along with her. 7. she shouldn’t be publicly referred to as your sugar momma. 8. she should certainly be faithful for your requirements along with your relationship. if she is not loyal, she may not be in a position to be faithful to you along with your relationship. 9. she should certainly be truthful with you and let you know what is on her brain. 10. search for a sugar momma who is confident with being a sugar momma. she is confident with giving you psychological and financial support.
Tips to really make the much of your sugar momma hookup
Sugar momma hookups are a powerful way to fulfill new individuals and possess some lighter moments. but there are many things that you must know to really make the much of your hookup. below are a few tips to take full advantage of your sugar momma hookup. 1. make certain you are more comfortable with anyone that you are starting up with. if you’re uncomfortable together, then it’s not likely to be good experience. 2. 3. don’t let yourself be afraid to ask anyone that you are setting up with questions. unless you understand one thing, then question them. 4. avoid being afraid become yourself. if you are uncomfortable aided by the individual that you’re hooking up with, then you shouldn’t be afraid to share with them. 5.
Get prepared for a sugar momma hookup
When you are looking for a sugar momma hookup, it is in addition crucial to be equipped for what to anticipate. here are five what to remember:
1. be upfront in what you’re looking for. if you’re in search of a one-time hookup, be upfront about this right away. if you should be longing for one thing much more serious, be truthful about this, too. honesty is key when it comes to sugar momma hookups, because if you should be perhaps not upfront by what you’re looking for, you might find yourself disappointed. 2. cannot expect a committed relationship. sugar momma hookups won’t be the same as traditional relationships. they’re more about convenience and intimate gratification. if you are seeking a committed relationship, you’re likely to be disappointed. 3. never expect to be the just one included. sugar momma hookups in many cases are about satisfying anyone’s sexual requirements. expect you’ll be involved in a sexual relationship with increased than one individual. 4. anticipate to be open and truthful. if you are taking part in a sugar momma hookup, anticipate to be open and honest with one another. this implies being truthful about your motives and expectations. if you are uncomfortable being open and truthful, you likely will become frustrated and disappointed. 5. anticipate to have some fun. sugar momma hookups are often about having a great time. what this means is being open to brand new experiences being ready to have some fun within the bedroom.