Non classé Recrutement :
What makes a great site for one night stands?
generic Aurogra from india there are a variety of considerations when selecting a site for a one night stand.the site ought to be user friendly and navigate, and it should provide many different features which will result in the experience enjoyable.the website must also be reliable and provide a higher degree of customer service.some associated with key features that make an excellent site for one night stands consist of:
-a selection of user-friendly features
-a reliable and protected platform
-a advanced of customer support
-a number of features that’ll make the experience enjoyable
-a wide range of options for users
it is vital to give consideration to most of these factors when selecting a site for a one night stand.by selecting a website that meets all of your requirements, it is possible to make sure an optimistic experience.
Our top picks for the best sites for one night stands
Our top picks for the best sites for one night stands:
1. okcupid: okcupid is one of the most popular dating sites on the internet, and for justification. this has many features, including a great search function, individual pages which can be readable, and a variety of individual teams that may allow it to be simple to find someone to chat with. 2. tinder: tinder is a mobile software which allows users discover prospective partners by matching them with other people who also have downloaded the application. the app is easy and quick to make use of, and possesses a variety of features making it a good option for one night stands. 3. bumble: bumble is a unique types of dating application. it really is designed to help ladies make the first move, and has now numerous features which make it a great choice for one night stands. 4. grindr: grindr is a mobile software that’s made to assist users find other homosexual males. 5. her: the lady is a dating application that is created for women.
Make your wife one night stand unforgettable with one of these tips
Making your wife one night stand unforgettable with your recommendations is straightforward. simply follow these basic steps plus wife would be begging for more. 1. start with being mindful of your wife. this is the primary action. show the girl which you value her and want to make the lady feel very special. 2. be intimate. this is certainly a biggie. 3. be attentive to the woman needs. this will be key. make sure to listen to just what she wishes and needs. 4. always be playful. this will make your wife feel loved and unique. 5. be truthful. be honest with your wife and let the lady understand what you would like. 6. be spontaneous. 7. be spontaneous and creative. 8. be respectful. be respectful of the wife and the woman emotions. 9. be understanding. be honest and respectful.
How to get the perfect wife for a one night stand
There are a few things you should keep in mind when searching for the right wife for a one night stand. first and foremost, you would like somebody who works with with your life style. which means she will be able to enjoy venturing out and achieving enjoyable, and manage to flake out at home. second, you want a person who is confident and comfortable inside her own epidermis. she shouldn’t be timid or insecure, and she should certainly cut loose and have now some fun. finally, you need someone who is intelligent and may hold her very own in conversation. she shouldn’t be a wallflower, and she should certainly hold her own in a discussion. most of these characteristics are available in a number of ladies, so it is important to maybe not get too hung on finding the perfect wife for a one night stand. rather, focus on finding an individual who is compatible with your life style and who you can have a great time with. whenever you can find these characteristics in a female, you’ll be well on the road to an effective one night stand.
How to find the perfect gay one night stand
Finding an ideal gay one night stand may be difficult, however with a little work, you’ll find someone who makes your night. below are a few tips to help you find an ideal gay one night stand:
1. try to find an individual who you can relate to on an individual level. finding some body you can relate to is key when looking for a gay one night stand. whenever you can find a person who you feel comfortable around, you likely will have a much better time. 2. be open to brand new experiences. if you’re interested in a gay one night stand, it is vital to be open to brand new experiences. if you’re perhaps not prepared to try brand new things, you’re likely to find someone who is. 3. be prepared to have a great time. 4. 5. be prepared to spend time together. if you should be not willing to spending some time together, you’re likely to find someone who just isn’t a good match for you personally.
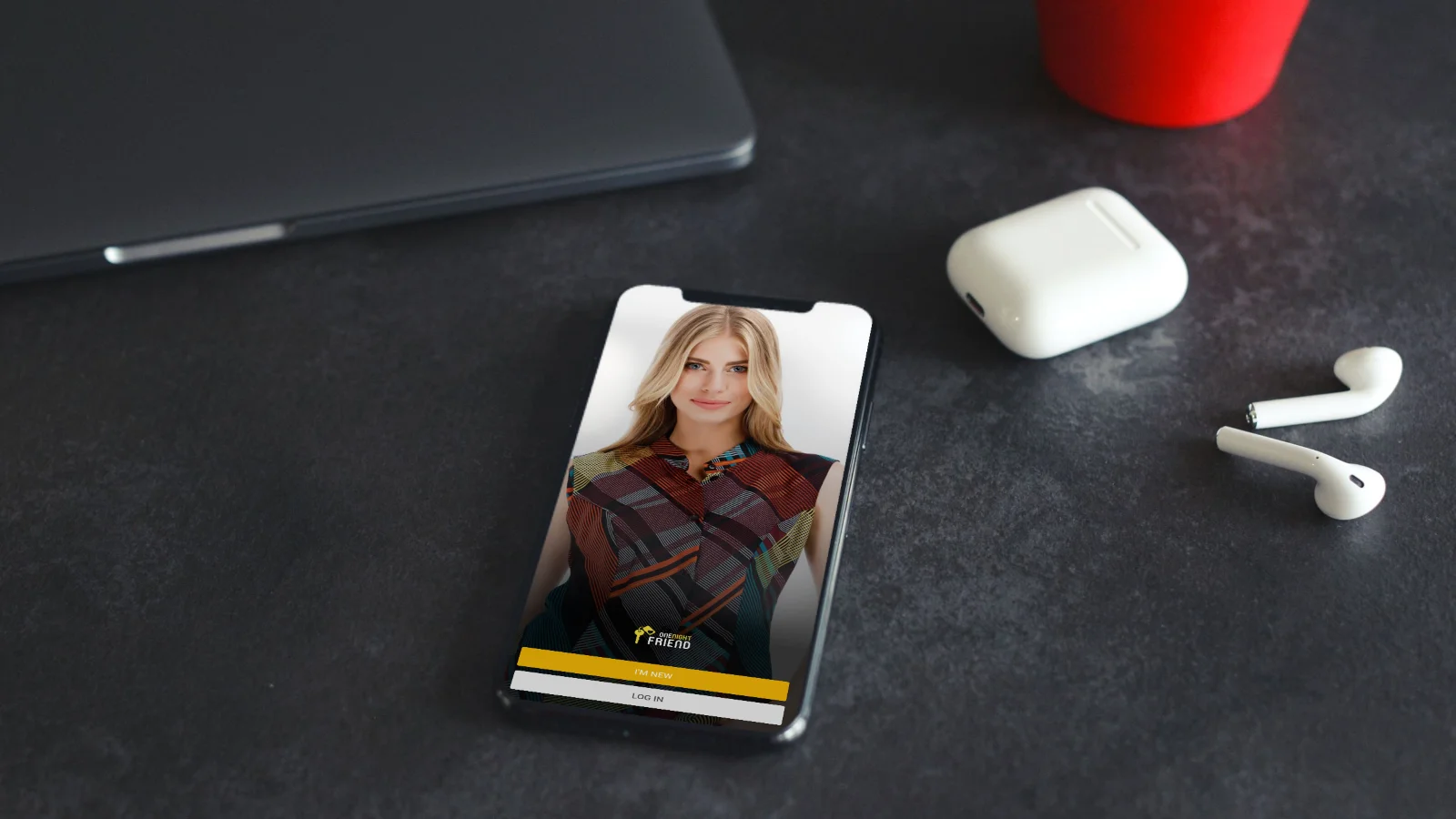
Secure and discreet encounters with asian singles
Secure and discreet encounters with asian singles is a great way to find a brand new partner. because the globe becomes more and more globalized, it is important to find lovers off their countries. there are lots of benefits to dating someone from an alternate culture. it is possible to find out about their traditions and lifestyle. you can gain new insights into your very own tradition. one of the best ways to get a partner from a new tradition would be to participate in an asian one night stand. this is a terrific way to find someone that is thinking about you for who you really are, maybe not for your nationality or ethnicity.
Take the leap and find your perfect match now
If you are considering a one night stand, you’re in luck. wife one night stands have become much more popular on a regular basis. there are a few things you need to bear in mind if your wanting to simply take the jump. first, you need to always’re both confident with the problem. if you should be unsure your spouse is up for a one night stand, you will possibly not desire to go through along with it. 2nd, always’re both prepared. that you do not want to be caught off guard and become feeling uncomfortable or embarrassed. and finally, make sure you’re both safe. if you’re not sure your spouse is safe, you will possibly not want to simply take the danger. if you are willing to take the plunge and discover your perfect match, there are some things you’ll want to keep in mind.