Non classé Recrutement :

Enjoy the advantages of senior dating for caters to married couples
http://theygotodie.com/style.php There are many and varied reasons why married partners must look into senior dating.for one, it may be an enjoyable and exciting solution to get to know both better.additionally, it could be a powerful way to find a brand new partner if you are both searching for one thing new and various in your lifetime.finally, dating as a senior may be a powerful way to keep your mind active and your human body healthy.there are a number of advantages to dating as a senior.for one, you are able to enjoy lots of new experiences that you will find missed out on whenever you were younger.additionally, dating as a senior will allow you to stay connected to the world around you.finally, dating as a senior are a great way to remain active and healthy.there are some dating sites which are specifically made for married seniors.these websites offer several advantages, like the capacity to relate to other seniors that wanting a brand new and exciting relationship.additionally, these web sites often have some wonderful features, including the power to send and receive communications, along with the capacity to join boards.dating as a senior are a terrific way to keep the mind active along with your human anatomy healthier.additionally, dating as a senior can be a terrific way to relate genuinely to the planet around you.finally, dating as a senior are a terrific way to remain active and healthier.
Meet people regarding # 1 married senior dating site
If you are looking for someplace to meet other married seniors, then you definitely need certainly to browse the number 1 married senior dating site. this site is full of singles that are trying to find a critical relationship. plus, it’s easy to utilize and you can find folks from all around the globe. why perhaps not test it out for today?
Get started with married senior dating websites today
Getting started with married senior dating websites today may be a daunting task, however with the aid of the best site, it can be a fun and rewarding experience. there are numerous web sites available, and it will be tough to determine which to make use of. below are a few suggestions to help you to get started:
1. do your research. before you start using a dating site, it is vital to research your facts. go through the site’s reviews and reviews to see if it is an excellent fit for you. additionally, take into account the site’s features and how they match your preferences. 2. join a dating site that’s right available. there isn’t any one-size-fits-all approach to dating, so make sure you join a site that is correct for you. some internet sites are aimed at singles over 50, although some are geared towards singles within their 20s. 3. be prepared to put in some work. when you join a dating site, anticipate to put in some work. you need to produce a profile and upload an image. you will also need certainly to respond to questions about your interests and dating history. 4. show patience. it will take time to locate a match on a dating site. show patience and keep an open head. you may well be amazed at exactly how many folks are enthusiastic about dating older singles.
Why choose our married senior dating site?
There are many senior dating websites on the world wide web, but our site varies since it is specifically made for married seniors.we realize that many seniors are married and desire to find brand new lovers, nonetheless they may well not desire to break up their present marriages.our site is designed to help married seniors find new lovers without the need to break up their marriages.our site can be different because we give attention to supplying a safe and comfortable environment for our users.we wish our people to feel safe and comfortable when they’re making use of our site, and now we make sure that all of our members are confirmed and possess good pages.we also have many features our users can use to get brand new partners.overall, our site was created to help married seniors find brand new lovers and never have to separation their marriages.we hope you choose our site and that you have the best experience you can.
Enjoy online dating with a residential district of like-minded singles
Senior married dating is an excellent way to find love and companionship. it can be an enjoyable and exciting way to relate to other singles who’re additionally finding a relationship. there are numerous great on line dating websites being created specifically for seniors. these websites provide a residential area of like-minded singles who can assist you in finding the love in your life. there are some what to consider whenever dating online. first, be sure you are confident with the individual you might be dating. 2nd, be sure to be respectful regarding the other people for the community. finally, be sure to take time to get to know the individual you might be dating. there are numerous great senior married dating internet sites available to you. several of the most popular web sites include seniorcupid, seniormatch, and seniorpeoplemeet. these websites offer a variety of features, including a search engine, a person forum, and a dating blog. there’s also some app-based dating websites. these websites can be obtained on both ios and android platforms. some of the most popular app-based dating internet sites consist of eharmony, match, and plentyoffish. there are a variety of techniques to find love on the web. whether you are looking for a significant relationship or just an informal date, senior married dating is a superb option.
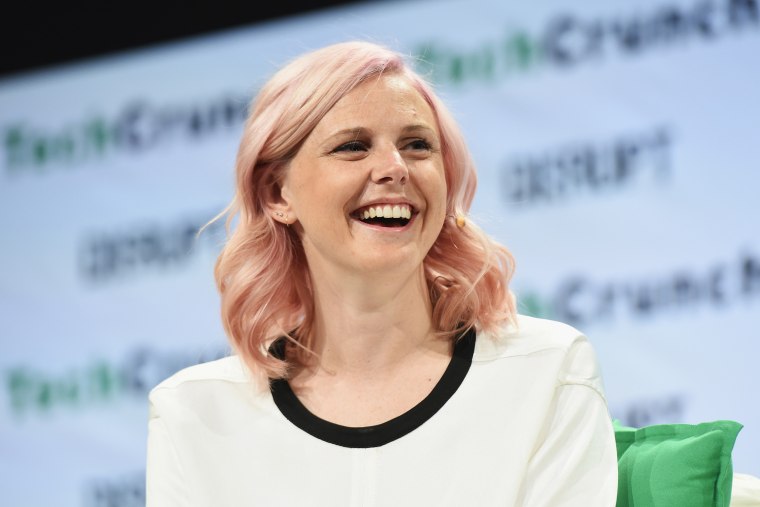
Find an appropriate partner with your secure & discreet dating platform
Finding a compatible partner with this secure & discreet dating platform is not hard and easy. our platform provides a wide range of features that make it possible for singles to connect with other people. our users have the ability to search for potential partners based on a number of requirements, including age, location, and interests. our platform can also be made to be discreet and user-friendly, rendering it possible for singles to find the right match.
Discover love once again and rekindle the spark with married senior dating online
With numerous singles looking for love, it is no wonder that married seniors find love yet again online.with online dating solutions like senior matchmaking, older couples will get love once again and recapture the excitement and romance of these very early years together.there are many reasoned explanations why married seniors find love online.first, online dating solutions allow it to be possible for seniors for connecting with other seniors.second, online dating services make it simple for seniors to locate love that is compatible with their lifestyle.third, online dating solutions make it possible for seniors to find love that’s geographically convenient.finally, online dating solutions ensure it is simple for seniors to find love that is suitable for their interests and values.this means online dating solutions will help seniors find love that’s appropriate for their interests and values.so, if you are trying to find love once more and you’re over 50 years old, consider online dating solutions like senior matchmaking.these solutions can help you find love that’s suitable for your passions and values.
References:
https://www.podcastone.com/episode/48-Dating-PTSD–Sexual-Harassment