Non classé Recrutement :
thinking about join an older lesbian dating site?
http://thelittersitter.com/? There are many reasons why you ought to join an older lesbian dating site.for beginners, these websites tend to be more selective in their account, meaning that you likely will find a more compatible partner.additionally, these websites often have a wider range of interests, which means you’re almost certainly going to find someone with who you share common passions.finally, these websites usually have a more mature and experienced membership, which can make them a good spot to learn about dating and relationships.
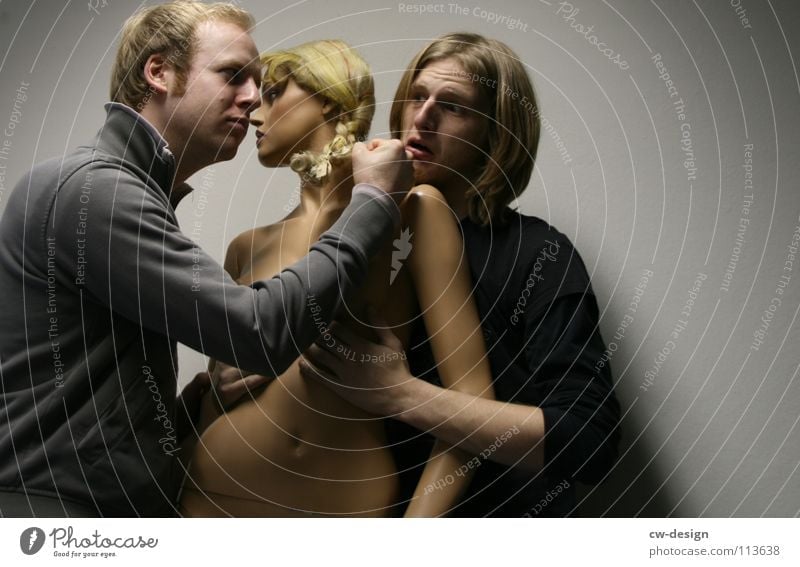
Enjoy a safe and safe dating experience
There are numerous great dating internet sites currently available for singles of all of the ages. but if you are searching for a dating site that’s created specifically for older singles, then chances are you should read the best dating site for older women. this site provides a safe and protected dating experience for singles avove the age of 50, which is full of features that will make your dating experience enjoyable and effective. one of the best reasons for having this site is that it’s created especially for older singles. which means you will not need to compete with younger singles for the interest associated with site’s people. furthermore, the site provides a wide range of features which will make your dating experience unique and unique. for instance, the site offers a wealth of dating opportunities, including on line dating, get redirected here loveexperienced.org forums, and discussion boards. this means there is the perfect match for you no real matter what your interests are. in addition, the site is quite user-friendly. which means you won’t have to spend hours trying to puzzle out utilizing the site’s features. in fact, the site is really so simple to use that even beginners can begin dating immediately. general, the best dating site for older women is a good choice for singles searching for a safe and protected dating experience. it includes quite a lot of features that may make your dating experience unique and special, and it is created particularly for older singles.
Find an ideal older lesbian dating site for you
If you’re looking for an older lesbian dating site that provides singles over 50, then chances are you’re in luck! there are numerous of good choices nowadays, and it will be difficult to decide what type is suitable for you. among the best reasons for having older lesbian dating sites is that they tend to be more selective. which means you likely will find someone who is an excellent match for you considering your interests and personality. another neat thing about older lesbian dating internet sites is that they frequently have a more mature and advanced environment. this means you might find people that are open-minded and that seeking a critical relationship. finally, older lesbian dating websites tend to be more energetic than more youthful lesbian dating websites. which means that you’re more prone to find anyone to date straight away. so, if you’re trying to find a great older lesbian dating site, you then should definitely discover one of many choices available to you.
Sign up now and commence fulfilling older women today
Are you searching for a dating site that suits older women? in that case, you have arrived at the best destination! here, you will find an array of dating options which will suit your needs. above all, we suggest our top dating site for older women, senior dating site. this site is ideal for those who are looking for a serious relationship. it is not only outstanding site for those over 50, but it also has a large user base that is diverse in age, race, and faith. another great choice for older women is cupid news’s senior dating site. this site is created specifically for seniors, and contains plenty of features making it a great choice. for example, it has a wealth of member pages offering pictures, bios, and passions. plus, this has a very active forum which filled with helpful seniors. if you’re searching for a dating site that caters to those over 50, we suggest our top two alternatives. but if you’re not quite prepared to commit to a long-term relationship, there are other dating internet sites which are perfect for you. for instance, our site for more youthful singles features a wide range of dating choices which are perfect for those who find themselves searching for an informal relationship. plus, it has a big user base that’s diverse in age, competition, and religion. so, never wait any more – register now and begin fulfilling older women today!
Find your perfect match: our comprehensive database of older women
Finding love could be difficult, nonetheless it doesn’t have become impossible. if you are looking for a dating site that suits older women, you then’ve arrived at the right spot. our database of older women is comprehensive and user-friendly, so finding your perfect match is straightforward. plus, our site is filled with interesting content and features that will keep you entertained. just what exactly have you been waiting for? start searching our database today!
What is an older lesbian dating site?
What is an older lesbian dating site explanation? an older lesbian dating site explanation is an online site or application created specifically for older lesbians. these dating sites provides a safe and supportive environment for older lesbians for connecting with other lesbian singles. older lesbian dating internet sites can also provide older lesbians with valuable resources and information regarding dating and relationships. there are many explanations why older lesbians may want to make use of an older lesbian dating site. older lesbian dating sites also can provide older lesbians with many different opportunities to satisfy new friends and potential partners. what are the great things about making use of an older lesbian dating site? some great benefits of using an older lesbian dating site are the following:
older lesbian dating web sites can offer a safe and supportive environment for older lesbians to connect along with other lesbian singles. there are a few disadvantages to utilizing an older lesbian dating site. first, older lesbian dating web sites can be difficult to get. 2nd, older lesbian dating web sites can be more costly than traditional dating web sites. tenth, older lesbian dating web sites is difficult to maintain a long-term relationship with. the downsides of utilizing an older lesbian dating site include the following:
first, older lesbian dating web sites may be difficult to get.
References:
https://static.130.82.4.46.clients.your-server.de/doc/krasota/momentalnyy-zagar.html