Non classé Recrutement :
Meet suitable singles and begin a meaningful relationship today
http://midequalitygroup.co.uk/events/2024-12-31/ Single chat rooms website is a superb way to satisfy appropriate singles and commence a meaningful relationship. with many people online, it is easy to find somebody who shares your passions and who you can relate genuinely to on a deeper level. the website is straightforward to make use of and navigate, as well as the chat rooms are a great way to make new buddies in order to find a relationship. the chat rooms are a great way to meet those who share your interests and also to find somebody who it is possible to relate genuinely to on a deeper level. the chat rooms are a powerful way to make new friends and also to find an individual who you can date.
Welcome to spokanesingles chatrooms
Welcome towards wonderful world of spokane singles chatrooms! these chat rooms are a https://www.singlechatroom.net/ terrific way to satisfy new individuals while making some brand new buddies. they truly are additionally a great way to check out new dating opportunities in your community. there are a variety of good spokane singles chat rooms available, while’re sure to find the one that’s ideal for you. whether you are looking for a place to speak about the latest movie or perhaps wish to make some new buddies, these boards would be the perfect destination to do so. so just why maybe not let them have a go today? you will not be disappointed.
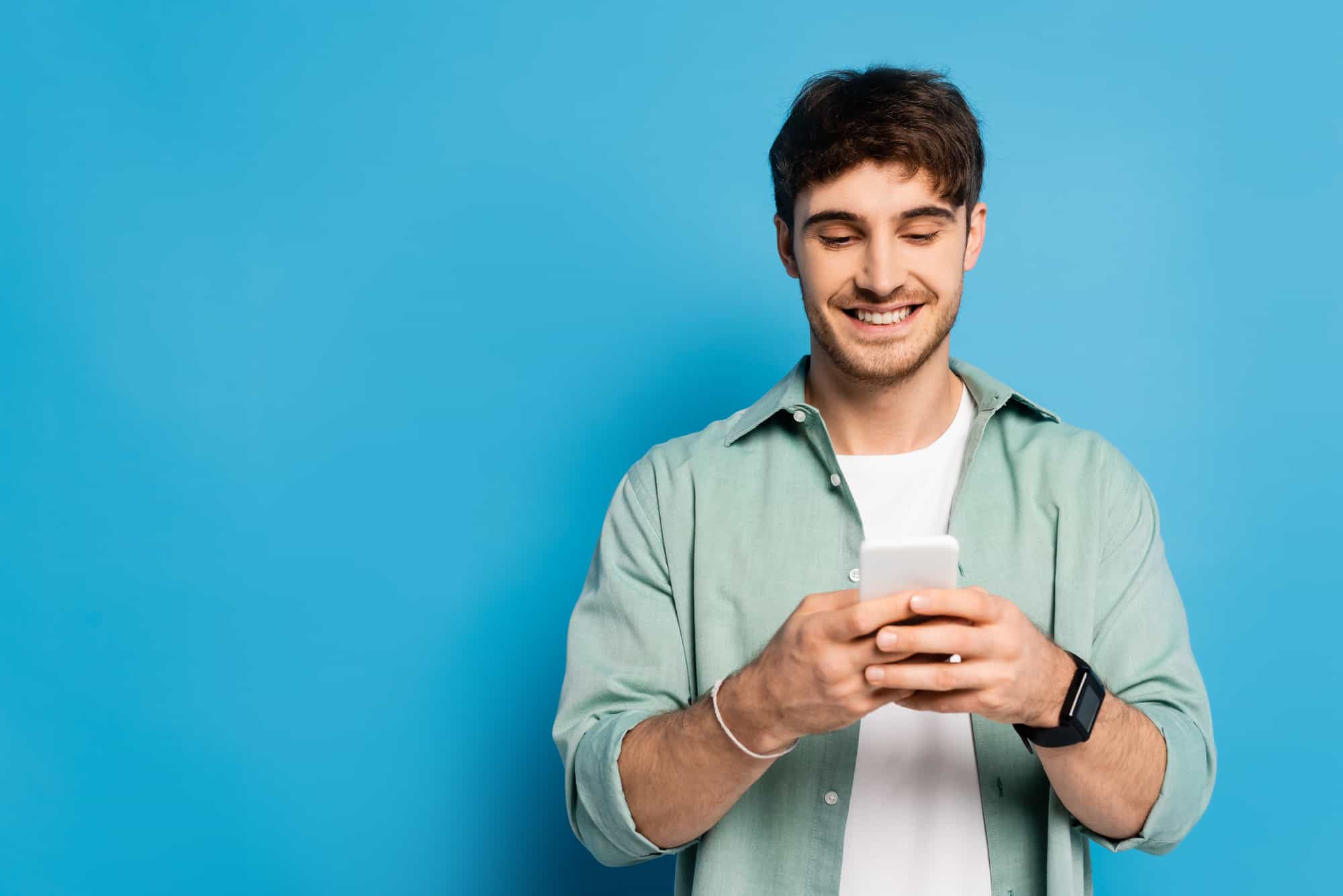
Find your perfect match in grand rapids’ singles chatrooms
Grand rapidssingles chatrooms are a great way to meet new individuals in order to find your perfect match. with many individuals to chat with, you are sure to find a person who shares your interests. plus, the chatrooms are a great way to get to know individuals better. whether you’re looking for someone to talk to casually or for a more severe relationship, the chatrooms are an excellent place to start. to obtain probably the most out of the chatrooms, always make use of the lsi key words. these keywords are specifically strongly related the chatrooms and can help you find the individuals you’re looking for. plus, through these keywords, you’ll be able to attract more attention through the other users. if you’re trying to find special someone, the chatrooms will be the perfect place to begin. therefore cannot wait any further, join the chatrooms today and commence finding your perfect match!
Find the best singles chat rooms for you
Finding the right singles chat room can be a daunting task. but never worry, we’re right here to greatly help. in this specific article, we will describe the best ways to get the perfect chat room for you. above all, make sure to research the various chat rooms available. not totally all chat rooms are created equal. some are intended for a specific market, although some are far more basic. once you have narrowed down your options, it is time to search for the right chat space for you personally. here are a few ideas to help you find the best singles chat rooms for you:
1. look for a chat room that is tailored towards passions. some chat rooms are specifically made for singles. these chat rooms tend to be more specific in terms of subjects and interests. this can be a terrific way to interact with like-minded individuals. 2. try to find chat rooms that are strongly related your local area. if you should be trying to find a chat space that is certain towards location, always try to find a chat space that is strongly related your area. it will help you relate genuinely to individuals who live in your area. 3. search for chat rooms which have a sizable user base. ch chat rooms with a large user base may be active. this means there are more odds of finding an individual who shares your interests. 4. numerous chat rooms are aimed at a mature individual base. which means the chat space is prone to be respectful and expert. 5. numerous chat rooms have a good community vibe. 6. the chat program is very important because it determines how effortless it really is to talk to other users. this may ensure it is simpler for you to locate and connect to other users. 7. look for chat rooms that have an excellent chat space environment. the chat space environment is important since it determines how enjoyable and engaging the chat space is. 8. chat rooms have actually a code of etiquette that must be followed. 9. 10. 11.
Join spokanesingles chatrooms and find your perfect match today
Spokanesingles chatrooms are a powerful way to satisfy brand new individuals in order to find your perfect match. with so many individuals on the web at any time, it’s easy to find anyone to talk to. plus, the chatrooms are a terrific way to get acquainted with individuals better. if you are seeking a method to satisfy brand new people and possess some lighter moments, then join a spokanesingles chatroom today!
Chat with spokane singles within our chatrooms
Spokane singles are seeking a spot to chat and connect with others. our chatrooms would be the perfect spot to do just that. it is possible to speak to singles from all over the spokane area, and you also’re certain to find someone who interests you. whether you are looking for a romantic date, a friend, or something like that more, our chatrooms are the perfect spot to start.
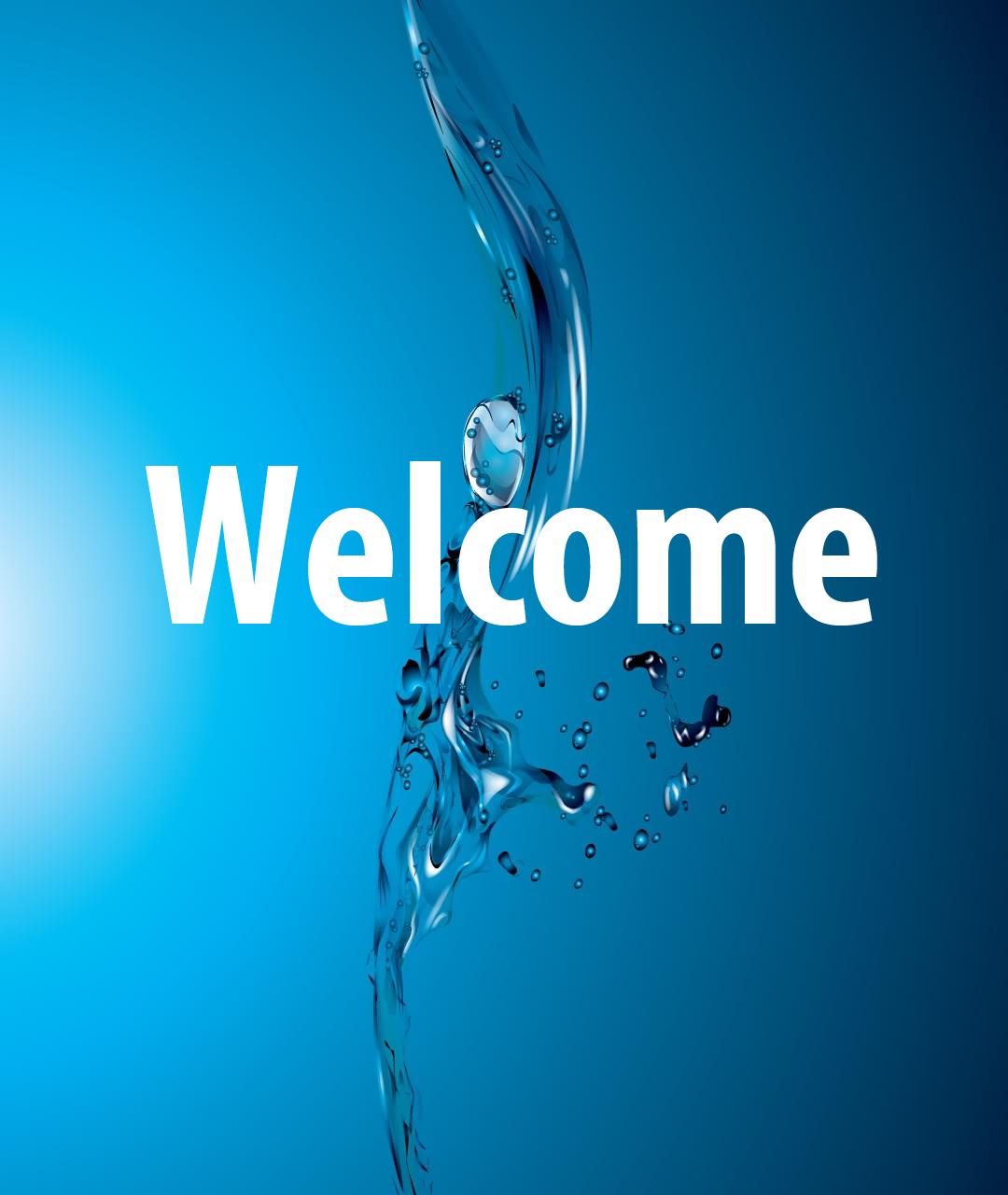
Find your perfect match in our single chat rooms
Single chat rooms website is the perfect destination to find your perfect match. with our substantial database of singles, you are sure to find someone who shares your passions and who you can connect to on a personal level. whether you’re looking for an informal discussion or something like that more severe, our chat rooms will be the perfect destination to find that which youare looking for. why wait? register today and begin chatting with the people you want to satisfy!
References:
https://meduza.io/en/feature/2021/07/19/the-brain-behind-russia-s-eye-of-god