Non classé Recrutement :
Unleash your desires with gay bdsm chat today
buy Lurasidone 40mg Looking for a way to get out of your everyday routine and explore something new?look no further than gay bdsm chat!this kind of chat is perfect for those who are trying to find a more intimate experience.with gay bdsm chat, you are able to explore your desires and dreams along with other like-minded individuals.this is a safe and private environment, ideal for those who want to explore their kinks and fetishes in a safe and discreet environment.if you are considering a way to speak to your concealed desires, gay bdsm chat may be the perfect solution.this chat is perfect for those people who are wanting an even more intimate experience.with gay bdsm chat, you’ll explore your desires and dreams with other like-minded individuals.this is a safe and private environment, ideal for those that want to explore their kinks and fetishes in a safe and discreet setting.gay bdsm chat is an excellent way to speak to your hidden desires.this chat is ideal for those who find themselves shopping for an even more intimate experience.with gay bdsm chat, you’ll explore your desires and dreams along with other like-minded individuals.this is a safe and private environment, ideal for people who wish to explore their kinks and fetishes in a safe and discreet setting.so exactly what are you awaiting?unleash your desires with gay bdsm chat today!
Unlock the potential of bdsm chat gay around today
When it comes down to bdsm chat gay, there isn’t any better option to explore your kink than with a small grouping of like-minded individuals.with our platform, you’ll explore your desires in a safe and consensual environment, without judgement or fear of reprisal.our chat space is designed for abdlchatrooms.com/bdsm-chat/ those who find themselves in search of a romantic experience, and all of us of specialists can be acquired 24/7 to help you explore your dreams and desires.whether you are considering a bdsm chat or just desire to chat about everything, our chat space is the perfect place for you.so exactly what are you awaiting?unlock the potential of bdsm chat gay around today!
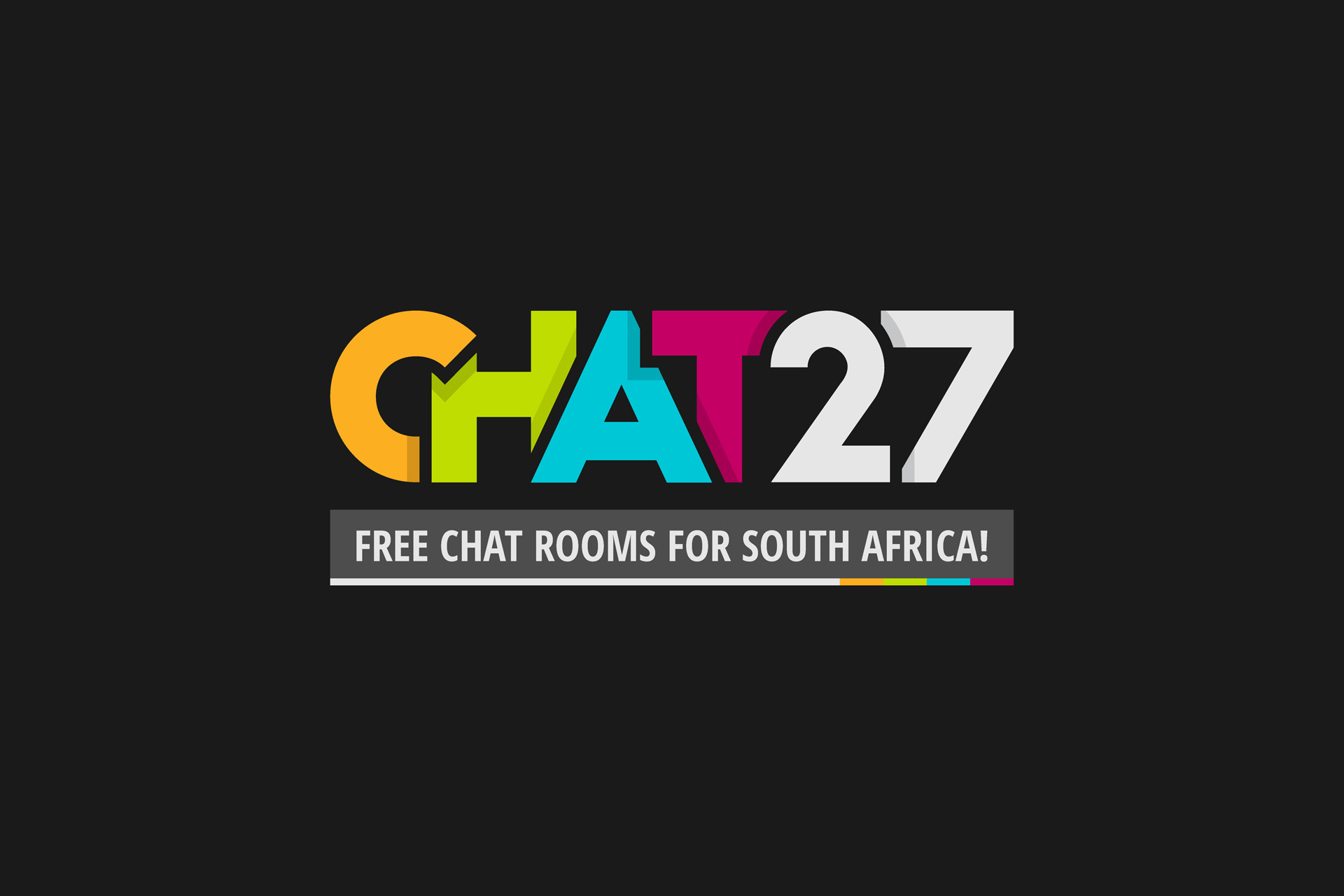
Find the right partner for your gay bdsm chat adventure
Finding the right partner for the gay bdsm chat adventure is a daunting task. with so many people on the web, it can be hard to find somebody who works with together with your passions and desires. the good news is, there are a few steps you can take to help make the procedure easier. first, be sure you are seeking an individual who works with your lifestyle. this means that they ought to share your exact same passions in bdsm chat along with other activities. if you should be shopping for anyone to role-play with, it is necessary that they are comfortable doing so and. 2nd, ensure you are interacting along with your potential partner. that is type in finding a compatible partner, since you need to be able to discuss your desires and dreams. if you’re incapable of repeat this, it may possibly be difficult to acquire a relationship that is satisfying for you both. finally, be sure you are fulfilling your potential partner in a safe and comfortable environment. which means that you ought to fulfill in a spot where there’s absolutely no threat of being caught. because of this, you will be certain that your tasks are safe and private.
Find the right partner for your bdsm chat desires
Finding the right partner for your bdsm chat desires can be a daunting task. with many individuals online, it can be hard to find someone who is compatible together with your kinks and desires. however, with some research, you’ll find the perfect partner for the bdsm chat needs. when searching for someone for bdsm chat, it is critical to consider your compatibility. can you both enjoy being spanked? are you both comfortable being naked facing each other? have you been both comfortable roleplaying various figures? once you have determined your compatibility, it is vital to find a chat room that is right for you personally. some chat spaces are more open-minded than others, and might be more accepting of different kinks. if you are searching for a more vanilla chat experience, you might try to find a chat room that’s more conservative. once you’ve discovered a chat room that is correct for you personally, it is vital to be respectful of the partner. don’t spam your lover, and start to become aware of your language. if you are experiencing uncomfortable with a conversation, do not wait to get rid of it.
