Non classé Recrutement :
Get prepared to find love aided by the elite: dating app for rich people
where can i get Misoprostol without a prescription Dating apps have become increasingly popular in recent years, as people search for ways to relate to others. one such app is called elitesingles, and it’s also created particularly for people that searching for a relationship or a married relationship. elitesingles is a dating app that is just open to people who are rich or have a top degree of income. elitesingles is a dating app that is created for people who’re looking for a relationship or a wedding. this is because elitesingles is targeted on finding a long-term relationship, and not just a one-night stand. it really is a really exclusive app, and it is only available to people who meet up with the qualifications. this really is a great choice for people who’re searching for a relationship or a wedding which according to mutual respect and a shared curiosity about equivalent things.
Get spoiled and pampered with a rich daddy
If you’re looking for a method to get spoiled and pampered with a rich daddy, then you definitely should take a look at a rich daddy dating site. these sites are created to assist you in finding a rich guy to date and spend some time with. they can give you sets from a deluxe lifestyle to a reliable home life. the best rich daddy dating websites are created to assist you in finding a man who’s suitable for your chosen lifestyle and interests. they will additionally enable you to find a person who’s economically stable and in a position to provide you with a higher comfortableness and luxury.
Create your profile and start connecting with rich girls now
Creating your profile on a rich girls dating site is a significant first step to find somebody whom shares your passions and life style. the site provides many different features making it an easy task to connect with like-minded females. first, you are able to create a profile that is tailored to your passions. you can list your preferred activities, hobbies, and interests, additionally the site will suggest matches according to those choices. it is possible to choose to show your wide range or absence thereof, which can help you find ladies who are searching for a partner with comparable savings. you can flick through profiles, deliver messages, and get together personally. the site also offers a chat feature that enables you to keep in touch with women in real time. finally, the site provides a wealth of resources to help you find the right partner. it is possible to access an abundance of data concerning the rich girls regarding site, including their bios, interests, and dating history. the site now offers a forum where you could ask questions and share advice.
Find your perfect match in richmond – casual dating at its finest
If you are looking for a casual dating experience in richmond, search no further than the numerous online dating websites available. with many options, it may be hard to determine which one is suitable for you. whether you are considering a casual dating site that is geared towards singles within the richmond area or one that’s more general, there’s a site nowadays available. one of the best reasons for online dating usually you can find an individual who is good for you, no matter where you’re in everything. whether you’re simply getting started or perhaps youare looking for an even more severe relationship, there clearly was a niche site available available.
Meet rich & attractive singles looking love
Introducing the rich girls dating site
if you should be finding a dating site that caters specifically to rich ladies, then chances are you’ve come to the right place. the rich girls dating site is a completely unique and exclusive dating site designed specifically for rich women. on rich girls dating site, you can find single ladies who are looking for severe relationships. these women can be interested in a person who can offer them the stability and security they crave. therefore, whether you are considering a long-term partner or a one-night stand, the rich girls dating site could be the perfect site for you. sign up now and begin fulfilling rich women
so what are you currently looking forward to? register now and commence fulfilling the rich and appealing singles that see link for find a rich womanking love in the rich girls dating site.
Discover your soulmate: a dating app for elite singles
Dating apps for elites are becoming ever more popular in recent years. there are a number of reasons for this. first, these apps focus on a distinct segment market that is thinking about finding somebody who shares comparable passions. second, the apps are made to connect elites together, rendering it easier for them to locate a compatible partner. one of the most popular dating apps for elites is elitesingles. this app is designed for people that wealthy and desire to find someone who shares their exact same life style. this has a database of over a million people that are looking for a relationship. elitesingles is not the actual only real app for elites. additionally, there are apps created for people that are successful within their jobs. for instance, matchmaker is an app that is designed for people who’re in a vocation that requires some networking. dating apps for elites could be a terrific way to find a partner whom shares your same passions.
Get to learn ladies who will give you living you deserve
If you are looking for a woman who are able to supply you with the life you deserve, then you should consider considering rich girls dating web sites. these sites are made for high-income people, and as a result, you will discover a lot of women who can give you precisely what you’ll need and much more. these websites provide some benefits, including the chance to satisfy countless interesting and eligible ladies who share your exact same interests and values. these websites provide lots of possibilities and resources, and as a result, you can actually achieve your goals faster than you’d if you were single.
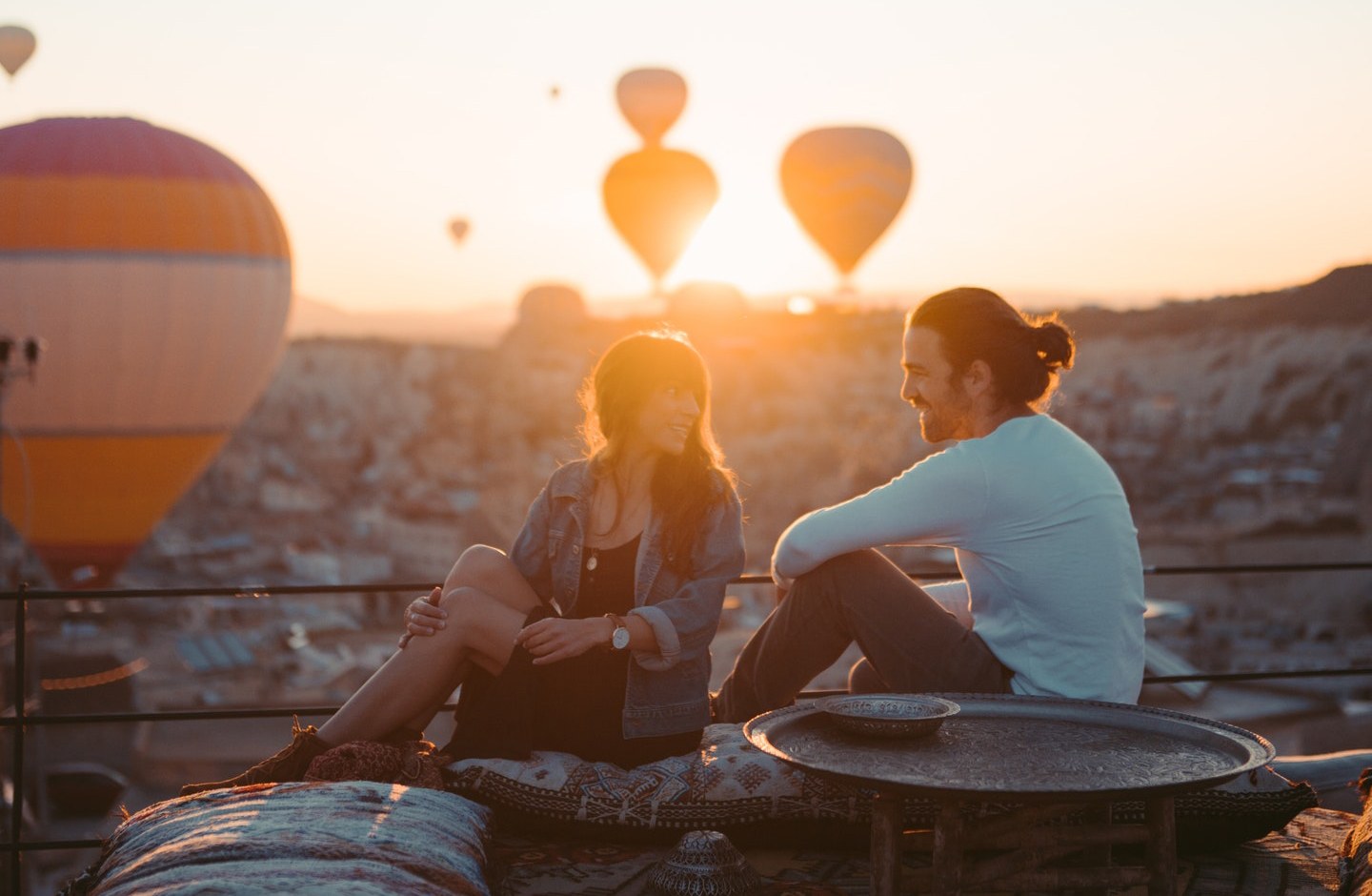