Non classé Recrutement :
The ultimate guide
http://preferredmode.com/tag/afropunk/ How to attach with a milf hookup site – the ultimate guide
if you are looking to add a small spice to your daily life, and possibly also find your real love, then chances are you should search into starting up with a milf. milfs are hot, horny, and ready for such a thing, and they understand how to have a good time. here are some recommendations on how to attach with a milf:
1. be confident
if you like to attach with a milf, you’ll need to be confident and also a positive attitude. milfs love dudes who’re confident and understand what they need, and they’re going to be more likely to desire to connect with you in the event that you show that you are a good man. 2. be respectful
similar to virtually any woman, a milf wants to be respected. make sure that you are always respectful towards her and her belongings. don’t be a jerk and behave like you are better than the lady. 3. if you should be open-minded and want to have a good time, she will likely be operational to it aswell. do not be afraid to take to new things and be adventurous. 4. you shouldn’t be a guy who is always attempting to force things. if you prefer to attach with a milf, be respectful of her some time let her know very well what you’re looking for. 5. don’t be a guy that is constantly attempting to touch the woman inappropriately or make intimate reviews. be respectful and respectful of the woman human anatomy. 6. respect her privacy and she will respect yours. 7. be respectful of her feelings and she will be respectful of yours. 8.
How to obtain the perfect naughty milf hookup
If you are considering a naughty milf hookup, you are in luck. there are lots of sexy ladies available to you that up for a great time. just be sure to find someone who works with your lifestyle and interests. check out suggestions to help you find the perfect naughty milf hookup:
1. look online
first, check online dating services. there are a lot of naughty milf hookups out there, therefore’re sure to find a person who is a perfect match for you. 2. join a dating website for naughty milfs
another strategy for finding the perfect naughty milf hookup would be to join a dating internet site designed for naughty milfs. these websites are specifically designed for people who are looking for a naughty hookup. 3. attend a naughty milf celebration
finally, if you cannot find what youare looking for on line or at a dating internet site, take to attending a naughty milf party. these parties in many cases are high in sexy women who are searching for a very good time.
Hot and horny milfs prepared to play
There’s nothing like a naughty milf getting your blood pumping! whether you’re looking for a quickie or a longer-term relationship, these horny ladies are often up for a good time. if you’re prepared to connect with a milf, listed below are five suggestions to help you get started. 1. be upfront
if you should be trying to find a naughty milf hookup, it is important to be upfront about what you’re looking for. allow the lady know very well what you find attractive and everything’re willing to do. this can help her become more selective and make certain that she actually is getting associated with an individual who works with. 2. expect you’ll get all the way
simply because a milf is naughty doesn’t mean she’s a pushover. ensure you’re prepared to get completely. this implies being prepared to do whatever it takes to obtain what you need. if you are not willing to go the excess mile, then you might want to reconsider setting up with a naughty milf. 3. make sure you’re respectful and do not treat the girl like a sex object. this can show the lady that you are serious about wanting to attach and certainly will help build a stronger relationship. 4. likely be operational to new experiences
just because a milf is naughty doesn’t mean she’s always finding equivalent things. be open to brand new experiences and decide to try something new. this can help to spice up your relationship while making it more exciting. 5. have patience and allow things develop at a normal pace. this will help ensure that your relationship is built on trust and respect.
The ultimate guide to setting up with a milf
If you’re like most guys, you’ve been searching for ways to attach with a milf. and, if you are similar to dudes, you are not really certain how to begin it. well, worry no more! in this article, i’m going to give you the ultimate guide to hooking up with a milf. to begin with, you will need to recognize that milfs vary off their females. they’re usually more capable, and they are in search of someone who will give them whatever they require. so, if you’re selecting a one-night stand, a milf probably is not the best option. as an alternative, you may need to find a woman who’s thinking about a long-term relationship. once you’ve identified a milf you want to attach with, the next step is to determine how to get her attention. there are a few things that you certainly can do to get her attention. first, you can try to charm the girl. it is possible to speak about your passions, and you will show the girl you are a good listener. 2nd, you can test to show her that you are a good provider. you are able to offer to simply take the lady out to dinner, or perhaps you can find her a gift. you can make sure you’re attentive to her needs, and you can ensure that you’re always ready to please her. once you’ve got the lady attention, it’s time to start hooking up with her. first, you will need to ensure that you’re comfortable with the idea of hooking up with a milf. if you should be unpleasant with the concept, you’ll likely never be able to attach with the lady. 2nd, you may need to ensure that you’re prepared the sexual encounter. you will need to be prepared to get completely, and you should need to be prepared to offer the woman perfect experience. and, finally, you will need to ensure that you’re ready to deal with any prospective fallout. if things do not go as prepared, you’ll likely have to deal with the consequences. therefore, if you should be searching to hook up with a milf, here is the guide that you might want. I really hope that you believe it is helpful.
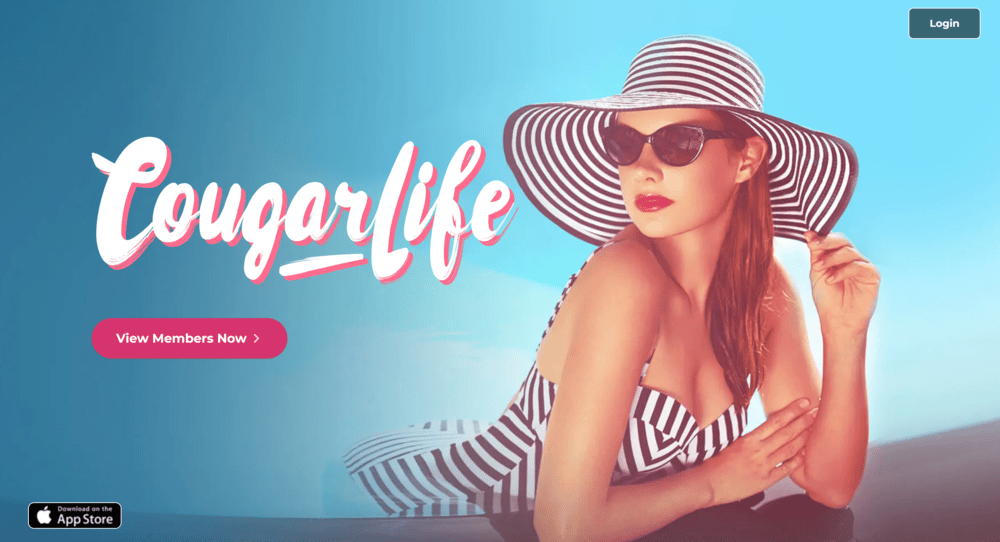
Get prepared to find your perfect milf hookup
If you’re looking for a milf hookup, you are in luck. there are numerous milfs around finding a great time, and also you’re certain to find one that’s perfect for you. here are some suggestions to help you get started:
1. be open-minded
you shouldn’t be afraid become your self when you’re shopping for a milf hookup. if you’re a good man, a milf will undoubtedly be interested in you. you should be your self and you will be fine. 2. be respectful
like with any other form of hookup, be respectful of one’s milf hookup. you shouldn’t be too rough or too demanding. let the lady set the pace and luxuriate in the knowledge. 3. be ready
always’re ready for a milf hookup. bring a great mindset and start to become prepared to have fun. you will both have a very good time.
Find your perfect match with this advanced level search features
Milf hookup is a term which frequently always describe a sexual encounter between a person and a lady that both over the age of 40. while this kind of encounter can be enjoyable for both events, it’s also dangerous if not done properly. below are a few suggestions to assist guarantee a successful milf hookup:
1. ensure you are both confident with the concept. let me give you, ensure both events are on exactly the same page. if one party isn’t more comfortable with the notion of a milf hookup, it’s likely your encounter will never be successful. 2. be respectful and understanding. it’s important to be respectful and knowledge of each other’s desires. cannot pressure them into any such thing they are not comfortable with. 3. be safe and accountable. remember, security and duty are fundamental factors in terms of any intimate encounter. make sure you are both aware of your environments and just take precautions to ensure both of one’s security. 4. have some fun. the goal of a milf hookup ought to be to have some fun. if you should be devoid of enjoyable, the likelihood is that the encounter will never be successful. try to relax and revel in the ability.
Find your perfect match with hookupmilfs
Looking for a casual hookup without strings attached? search no further than hookupmilfs. these milfs are down for anything and can satisfy your every need, whether you’re in the mood for a quickie or a full-blown event. if you’re seeking a no-strings-attached intimate encounter, then hookupmilfs is the site for you personally. these milfs are open-minded and can do just about anything to get your attention. whether you’re looking for a one-time hookup or a longer-term relationship, these milfs are sure to please. just what exactly are you awaiting? register today and commence browsing the pages of hookupmilfs. you will not be sorry!
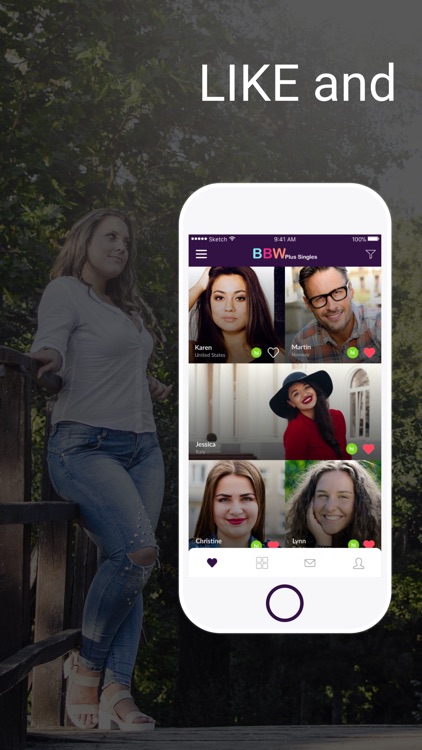
References:
https://www.muscleandfitness.com/women/dating-advice/5-secrets-to-dating-older-women/