Non classé Recrutement :
Get prepared to enjoy a wild couples looking for other couples hookup
http://rodneymills.com/hnjaz/matthew-astorga-car-accident.html Couples hookup can be a wild and exciting experience, if you are prepared for it. below are a few suggestions to prepare yourself:
1. ensure that your relationship is healthier. if you’re uncertain whether your relationship is healthy, ask your partner. if you are unsure whether your spouse is thinking about couples hookup, inquire further. 2. discuss your objectives. exactly what are you seeking from couples hookup? looking for a one-time occasion, or are you looking for something more? are you looking for a sexual encounter, or looking for a relationship? looking for something serious or are you searching for a casual fling? after you have answered these questions, you could begin setting boundaries. 3. ensure that your environment is conducive to couples hookup. cannot bring your complete toolbox of relationships luggage to couples hookup. if you should be looking something severe, don’t bring your full psychological toolbox to couples hookup. set some ground guidelines in the beginning in the act, and stay glued to them. 4. most probably to brand new experiences. if you’re wanting something serious, most probably to attempting brand new things. you shouldn’t be afraid to experiment. 5. be equipped for such a thing. if you should be interested in a significant relationship, be ready for a critical relationship. if you’re finding an informal fling, be equipped for a casual fling. 6. have fun. if you’re not having enjoyable, couples hookup is not going to be enjoyable for you personally or your partner. have fun, and enjoy the knowledge. 7. be safe. if you’re not safe, never do couples hookup. if you’re maybe not safe, don’t get anywhere. be safe, and also enjoyable. 8. be respectful. if you are maybe not respectful, don’t do anything.
Ready to begin your couples hookup adventure with a woman?
if that’s the case, you are in fortune!there are a great amount of women available that looking for a brand new adventure, and you’re simply the person for the job.first, you’ll need to make sure that you’re both thinking about this sort of relationship.if you’re not sure, ask your potential romantic partner if she actually is enthusiastic about exploring this sort of relationship.if she actually is available to the theory, you then’re willing to begin looking for couples hookups.once you have verified that the two of you want, you will need to begin considering what kind of couples hookups you need to engage in.there are some different alternatives accessible to you, so it is important to choose a thing that the two of you will love.if you’re looking for one thing intimate, you can look at happening a date.this are a fun option to get to know one another better to discover when you have any typical interests.if you are looking for one thing more physical, you can test going on a night out together with your partner.this is an enjoyable option to acquire some fun and excitement in your relationship.whatever you decide on, ensure that you’re both happy with it.if among you isn’t happy, it will not be worthwhile to carry on the partnership.so make certain you both have a good time which you’re both enjoying yourselves.ready to start out your couples hookup adventure with a woman?if therefore, you are in luck!there are lots of women on the market who’re looking for a brand new adventure, while’re just the person for the work.first, you will have to make certain you’re both enthusiastic about this type of relationship.if you are not sure, pose a question to your potential romantic partner if she’s thinking about exploring this type of relationship.if she’s available to the theory, then chances are you’re ready to start looking for couples hookups.once you have verified that the two of you have an interest, you will have to start considering what kind of couples hookups you intend to engage in.there are many different choices open to you, so it’s vital that you select something which the two of you will relish.if you are looking for something intimate, you can test happening a night out together.this can be a fun way to get acquainted with both better to see for those who have any typical passions.if you are looking for something more real, you can test happening per night out together with your partner.this could be an enjoyable option to get some good enjoyable and excitement inside relationship.whatever you decide on, make certain you’re both satisfied with it.if among you isn’t happy, it’s not going to be worth every penny to carry on the relationship.so ensure that you both have fun and that you’re both enjoying yourselves.
what’s a couples hookup?
A couples hookup is a sexual encounter between two people who’re maybe not hitched or in a committed relationship.it can be a casual encounter, or a more serious relationship move.couples hookups can be enjoyable and exciting, or they can be high-risk and dangerous.why do people participate in couples hookups?people engage in couples hookups for many different reasons.some individuals take pleasure in the thrill regarding the not known.others are looking for a fresh and exciting sexual experience.still other people are looking for a method to spice up their current relationship.what are the risks and benefits of couples hookups?the dangers and advantages of couples hookups depend on the person situation.some risks connected with couples hookups include the danger of stds.other dangers range from the threat of emotional and physical damage.what would be the most readily useful how to avoid couples hookups?the most useful methods to avoid couples hookups include being careful and making use of protection.additionally, be sure to talk to your lover about your intentions and objectives.
Make your few hookup benefit you
Make your couple hookup be right for you by integrating the proper keywords into the conversation. couples hookups with are a great and exciting experience, but it is crucial that you ensure they are a good fit for both parties. here are some suggestions to make your few hookup meet your needs:
1. mention that which you’re looking for. just before even begin, it is important to have a conversation by what you are both interested in. this may assist avoid any potential disputes or frustration down the line. 2. respect both’s boundaries. what this means is being truthful in what you’re comfortable with and avoiding anything that could possibly be uncomfortable or embarrassing. 3. most probably to trying brand new things. among the benefits of couples hookups is you can experiment with new things. this is often a great way to enhance your relationship. 4. avoid being afraid to fairly share intercourse. sex is a big part of any relationship, and it’s really vital that you likely be operational in what you need and do not wish. this may help avoid any awkwardness or shocks later on. 5. be communicative. one of many key advantages of couples hookups is you are able to communicate better. what this means is being open and truthful with one another. if you can find any problems or concerns, make sure to discuss them.
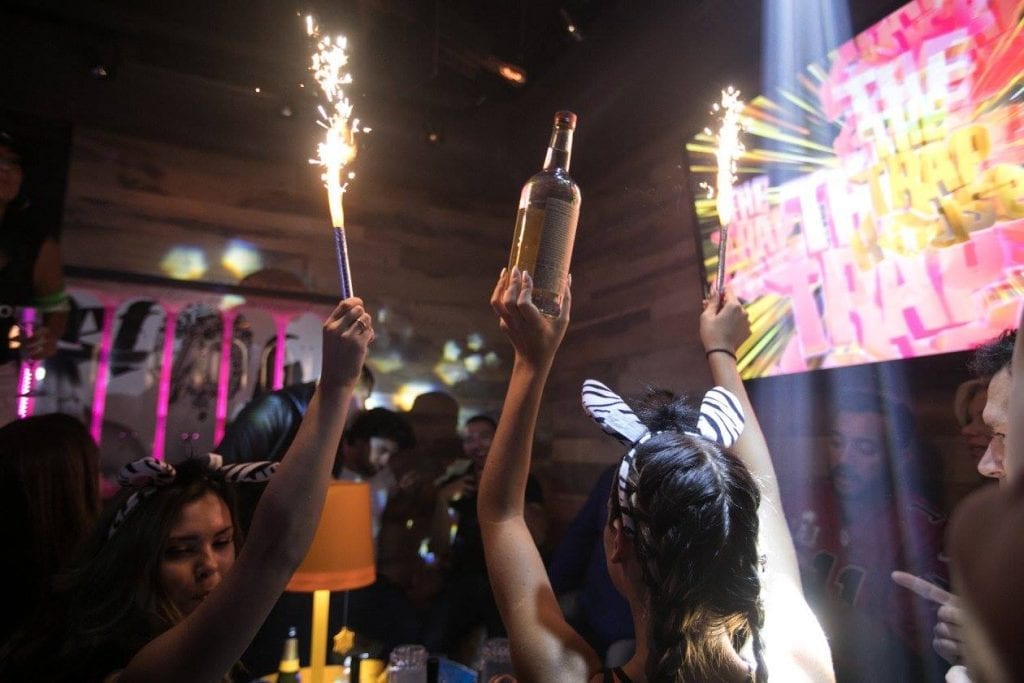
Find an ideal match for the couple
When it comes down to locating someone, it could be difficult to know how to start. if you should be shopping for anyone to share your lifetime with, or just anyone to have a blast with, you will want to make sure youare looking the right individual. and, if you should be looking someone to connect with, it’s also important to make sure you’re finding the right individual. there is a large number of considerations when searching for someone, and, if you’re interested in anyone to connect with, it is additionally vital to start thinking about things like chemistry, compatibility, and intimate passions. but, if you are shopping for a long-term relationship, additionally wish to think about things like compatibility and interaction. if you are in search of a partner that is compatible, it is additionally vital to always’re both shopping for the same things in a relationship. for example, if you’re wanting anyone to share everything with, as well as your partner wants anyone to hook up with, your relationship likely will not be effective. similarly, if you are in search of an individual who is communicative, you will want to ensure your partner is communicative and. in case the partner just isn’t communicative, thereforeare looking for an individual who is, your relationship is probably perhaps not likely to work. so, if you are selecting you to definitely attach with, make sure you’re looking for a person who works, communicative, and seeking for similar things in a relationship as you are. and, if you should be finding a long-term relationship, make sure you’re looking for an individual who is compatible and communicative also.

Experience the excitement of a woman looking for couples hookups today
If you are looking for one thing new and exciting in your life, then chances are you should definitely give consideration to checking out the thrill of a woman looking for couples hookups.this variety of dating can be very worthwhile, and it can offer you a lot of enjoyment and excitement.if you find attractive attempting this away, then you should truly start thinking about doing so today.there are lots of benefits to dating a woman looking for couples hookups.for one, you can experience some new and exciting things.this style of dating can be very stimulating, and it can help you to explore your sexuality in a brand new means.additionally, you can actually meet brand new people while making brand new connections.this may be a tremendously valuable experience, and it can enable you to expand your community and build new relationships.if you have in mind checking out this type of dating, then you should definitely achieve this today.it is a lot of fun, and it will provide you with a lot of brand new and exciting experiences.
Find your perfect couples hookup with our easy-to-use platform
Looking for ways to spice up your relationship? couples hookups with our easy-to-use platform could be exactly what you’ll need! with our platform, you can find the perfect match available and your partner very quickly anyway. plus, our easy-to-use search features make discovering the right couple hookup easy. so what are you looking forward to? start searching our database today!