Non classé Recrutement :
Find your match: get associated with rich women now
buy disulfiram uk Dating rich women are a great way to find a partner who has all you are seeking. not merely are they rich, nevertheless they likewise have many expertise in the world. which means that they are probably be familiar with lots of subjects, which could make for outstanding conversation partner. also, they might have connections that will help you receive what you would like. if you are seeking a partner who can provide economic stability, dating a rich woman could be the best option for you.
just what does it take to date rich women?
Dating rich women are a terrific way to find someone with a strong financial foundation. however, it could be difficult to date somebody who is rich. there are some items that you must do currently a rich woman. first, you have to be confident and now have an excellent sense of self-esteem. rich women are often attracted to guys that are confident and now have good feeling of self-worth. 2nd, you have to be in a position to speak about cash. rich women tend to be enthusiastic about studying their partner’s financial predicament. finally, you have to be in a position to handle your finances responsibly. rich women tend to be very savvy along with their money. if you cannot handle finances responsibly, you won’t manage to date a rich woman.
How for probably the most away from rich women dating sites
If you’re females looking for sex for ways to improve your dating life, then you definitely should consider making use of rich women dating sites. these sites were created for people who are seeking a significant relationship, and so they offer many different advantages that will help you discover the right match. one of the better things about rich women dating sites usually they provide a wide range of choices. you can find sites being centered on a specific style of girl, or perhaps you can browse in order to find a site that fits your interests. another advantageous asset of making use of rich women dating sites is that they often times have actually a big pool of possible partners. this means that you will find lots of singles that are enthusiastic about dating some body as if you. finally, rich women dating sites provide many different benefits which will help you find the proper relationship. these sites often have features that help you find matches, plus they frequently have forums that offer advice and help.
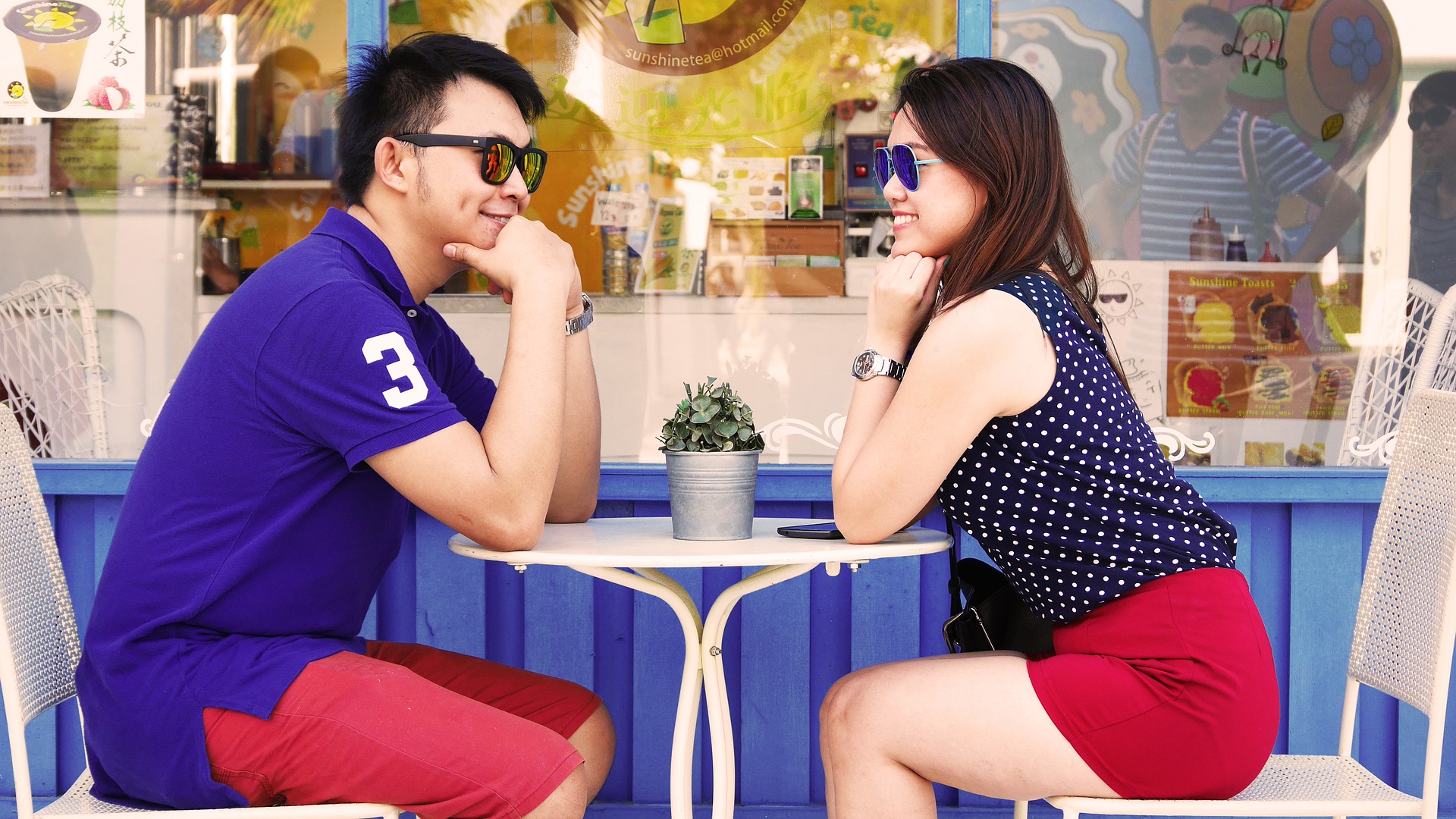
Get access to high-quality dating resources that allow you to link quickly
Dating sites for rich women can be a terrific way to meet new individuals in order to find a potential partner. however, before you decide to register for a dating website, you should know associated with different alternatives available additionally the features being vital that you you. several of the most popular dating sites for rich women consist of elitesingles, eharmony, and match.com. each of these sites has its own features and advantages, therefore it is crucial that you compare them just before decide. elitesingles is a dating website that is specifically designed for individuals who are wealthy and want to find someone. it’s several features which are important to rich individuals, such as reduced account that gives more features and a faster search procedure. eharmony is another popular dating website for rich women. it’s many features which can be crucial that you them, like a database in excess of 50 million users and a matching system that is predicated on compatibility. match.com is a dating website which well-liked by folks of all many years and incomes. this has numerous features which can be crucial that you rich individuals, such as for example a user-friendly program and a number of dating options. whichever dating website you choose, be sure to research the various choices and features before you subscribe. in this way, you can find the site which ideal for your needs.
Discover the benefits of dating a rich woman
Dating a rich woman can be a very gratifying experience. not just will they be rich, however they also provide many knowledge and experience to share with you. they may be really right down to earth and easy getting and. plus, they may be very ample along with their time and resources. if you are trying to find a relationship which is saturated in luxury and convenience, dating a rich girl is the path to take. here are a few associated with benefits of dating a rich woman:
1. they’re prone to be interested in you. one of the biggest advantages of dating a rich woman is that they are more likely to be interested in you. they are apt to be more selective in who they date, and they are likely to be more interested in a person who is rich and effective. which means that you are prone to get a romantic date with a rich woman. 2. rich women are more ready to spend time with you. they’re not as busy as other folks, and they’re often interested in having a good time. 3. they are more prone to be ready to give you a hand. they’re much less selfish as others, and they’re usually more ready to help out other people. 4. rich women usually are more willing to offer you a financial boost. they may be more nice along with their cash, and they are frequently more willing to assist you economically. 5. they’re usually more willing to provide you with advice which help you away along with your career.