Non classé Recrutement :
Find your perfect match: tips for fulfilling older women online
Aichach Finding an ideal match may be difficult, but it is maybe not impossible because of the right tips. below are a few to help you meet older women online:
1. begin by searching online internet dating sites. this is certainly a terrific way to find prospective matches whom share your passions. you may want to look for certain types of older women, such as retired women or women with young ones. 2. join dating teams. this is a powerful way to meet other singles and also make new friends. you can also find teams that concentrate on particular age ranges or interests. 3. usage social media. that is a powerful way to stay in touch with potential matches and find out whatever they’re around. you are able to make use of social media marketing to locate matches who reside towards you. 4. attend events. 5. usage online dating services. this really is a terrific way to find matches that are already living towards you. you may also utilize online online dating services discover matches who’re enthusiastic about similar things.
Take control of the love life – meet single women within area
Are you finding a method to manage your love life? in that case, you’ll want to begin looking for single women online. there are a lot of great singles on the market, and with the right approach, you will find the one that’s suitable for you. the initial step is to look for an excellent dating internet site. there are a lot of great people nowadays, and you can find one which fits your needs. when you find a niche site, you will need to start using it. what this means is joining up, producing a profile, and filling in the information. make sure to consist of your interests, your aims, and your lifestyle. this can assist you in finding matches that are an excellent complement you. once you’ve a good profile, the next thing is to begin in search of matches. this is where the online dating website comes in handy. you can search by interests, location, and more. you have to be casual and friendly. you can question them questions about on their own. this will help you get to know them better. after you have a great relationship with a match, the next phase is to just take things further. what this means is dating. you can date face-to-face or online. in either case, you need to be prepared for things to go wrong. that’s why it’s important to have a good plan set up. you need to know what you want, and you also need to be prepared to simply take things slow if things never work out. general, online dating is a superb way to manage your love life. it’s easy to utilize, and it can support you in finding a match that’s a great complement you. just be sure to have a plan in place, and start to become ready for things to make a mistake.

Ready to locate your perfect match?
Single women will always in search of brand new possibilities to meet brand new individuals and date. online dating is a great method to meet brand new singles in order to find your perfect match. there are lots of online internet dating sites available, and each features its own unique features and advantages. probably one of the most popular online dating sites is match.com. match.com is a totally free online dating website that provides many different features and benefits, including the ability to look for singles local dating servicesly, view photos, and deliver messages. match.com comes with a feature called « matches available. » this feature allows you to see every one of the matches that other users are making in the past, along with the matches they have been thinking about. this is certainly a powerful way to find potential matches that you could not need otherwise discovered. another great online dating website is eharmony. eharmony is a paid online dating site, nonetheless it provides a number of features which make it well worth the fee. eharmony provides a matching algorithm that’s designed to find you a partner who’s similar to you. in addition has an attribute called « eharmony fits. » there are also a number of free online online dating sites available. these websites provide a restricted amount of features, but they are still a powerful way to meet new individuals and date. whatever online dating website you select, ensure that you take time to explore every one of its features and advantages. there is a constant know, you will probably find your perfect match on one of those websites.
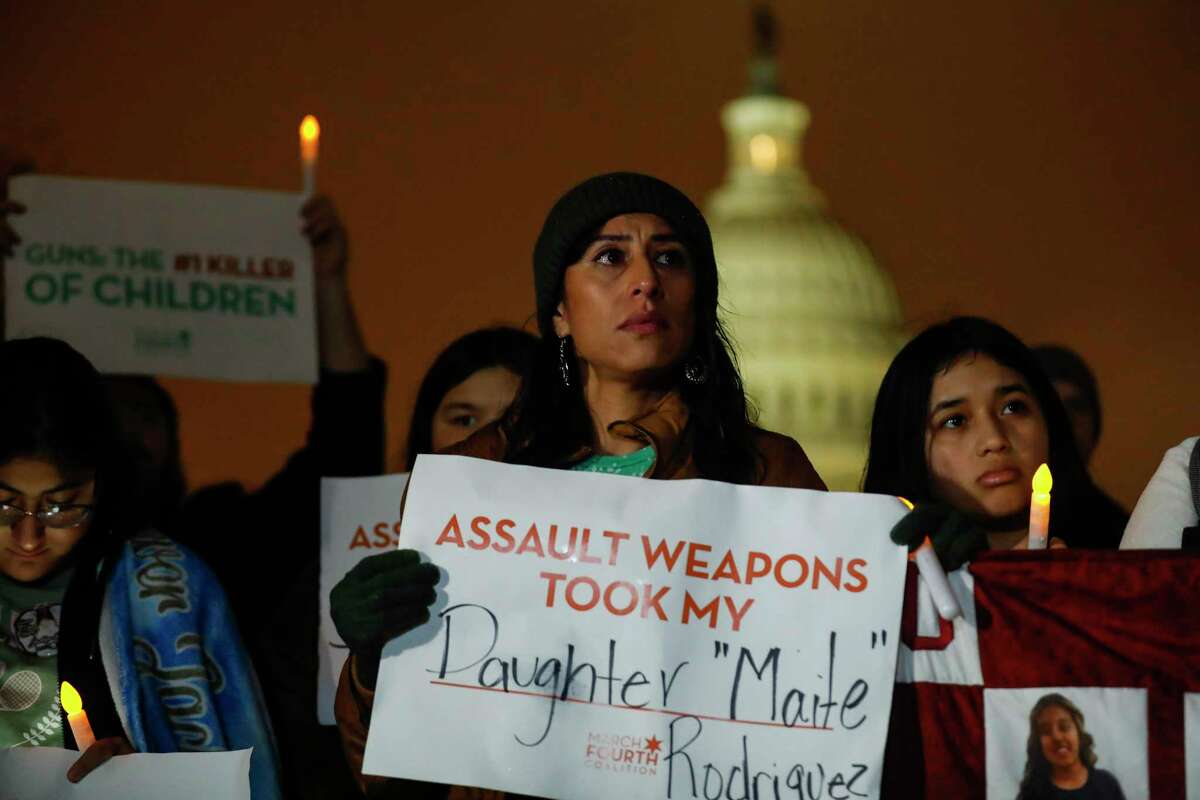
Ready to locate love? here’s getting started
If you are considering love, therefore’re unsure how to start, you’re not alone.in fact, many people feel lost regarding dating.if you are considering love, while’re uncertain the place to start, you’re not alone.in reality, many people feel lost with regards to dating.that’s why we are here to help.we’ve got some suggestions about how to start off, and now we wish to share it with you.the first rung on the ladder is to have a look at your aims.what would you like out of a relationship?do you would like a person who it is possible to share everything with?do you want someone who you’ll have fun with?or are you wanting someone who you are able to relate with on a deeper degree?once do you know what you are considering, you could start to look for indications.do you prefer hanging out alone?is hanging out together with your buddies your first concern?are you drawn to those who are independent?once do you know what you’re looking for, you could start to find indications.do you love hanging out alone?is spending some time together with your buddies your first priority?are you drawn to those who are separate?if you are looking for love, and you also’re not sure how to start, you aren’t alone.in reality, many individuals feel lost when it comes to dating.that’s why we are right here to help.we’ve got some suggestions about how to start off, and now we wish to share it with you.
Sign up now and enjoy a secure and personal dating experience
When you join a dating account with a niche site like ours, you’re getting to be able to enjoy an exclusive and secure dating experience.our website is made for folks who are looking a critical relationship, and now we wish to make sure that you have the best possible possibility of choosing the individual that you’re looking for.we simply take the security of our members extremely really, and we ensure that our data is protected utilizing the latest security measures.we have a group of experts who’re available 24/7 to assist you with any questions that you will find.so why don’t you sign up today and start enjoying the dating experience that we have to give you?
Find love with single women who share your interests
Single women whom share your passions can be a great way to find love. through online dating services, you’ll connect to singles who share your passions, which will make dating and relationships a great deal more enjoyable. you can also find singles whom share your values and opinions, which will make dating far more comfortable.