Non classé Recrutement :
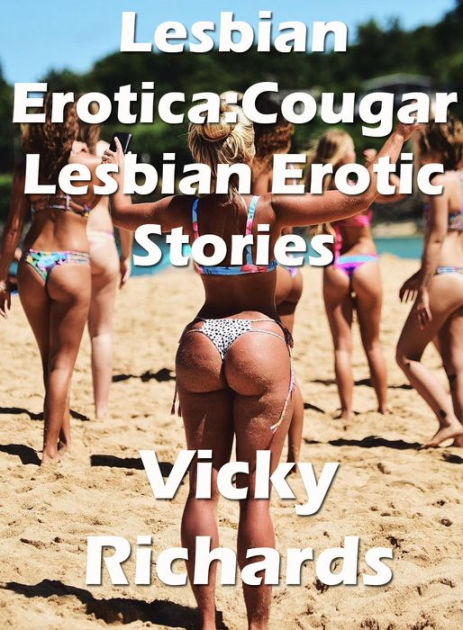
Enjoy a secure and safe lesbian asian dating experience
http://californiawithkids.com/tag/rancho-cordova/ When it comes to locating somebody, there are many possibilities for your requirements. if you are seeking a traditional relationship, you can test dating some one from your own ethnic or cultural background. alternatively, you could test dating somebody from another country or area. if you are shopping for one thing a little more unique, you could test dating a lesbian. lesbian dating can be a fun and exciting experience, however it may also be slightly high-risk. there are a few activities to do to make sure that your dating experience is safe and secure. first, make certain you are employing an established dating site. sites like okcupid and match.com offer many options, and that means you’re sure to find a site that is perfect for you. second, ensure that you are employing a secure connection when you are on the web. this can help protect important computer data from being stolen or hacked. finally, ensure that you are always alert to your surroundings. should you feel as you are being followed or that somebody is attempting to steal your phone, please contact the authorities. general, lesbian dating could be a fun and exciting experience. just be sure you are alert to the risks involved and that you might be utilizing safe and secure ways to protect yourself.
Get ready for love: strategies for asian lesbian dating success
Are you looking a lesbian dating site that caters particularly to asian females? if that’s the case, you’re in luck! there are a number of great asian lesbian dating sites on the market that will help relate genuinely to other women who share your passions. below are a few strategies for success on asian lesbian dating sites: 1. be proactive. start with trying to find sites that match your passions and browsing through the profiles of people. this may give you an improved notion of what types of individuals are available and what kind of conversations you could be capable have. 2. pay attention to town. once you’ve found a niche site that you’re enthusiastic about, be sure to take a look at user discussion forums and /asian-lesbian-dating/ boards to have a sense of the environment and what individuals are searching for. 3. expect you’ll make good first impression. whenever you join a dating site, you are placing yourself available. make certain you’re willing to be viewed and heard. 4. be respectful. keep in mind that you are coping with other people’s emotions and feelings, therefore be respectful of their privacy and emotions. don’t be afraid to inquire of concerns if you don’t understand something or you’re not sure how to proceed. 5. most probably to new opportunities. if one thing seems right, do it now. if something doesn’t feel right, most probably to permitting go. there isn’t any have to force any such thing if it is not right for you. be proactive, be respectful, and start to become open to new opportunities – and you will certainly be on the road to locating the love of your life!
Unlock the joys of lesbian asian women dating
Asian women are recognized for being separate and strong-willed. this can ensure it is difficult for them discover partners who share their values. lesbian asian women dating can be a great way of these women to find partners whom share their passions and values. there are a number of advantageous assets to dating lesbian asian women. first, these women are likely to be more understanding and supportive than guys in terms of dilemmas like sexuality and sex identification. second, lesbian asian women will tend to be more open-minded than guys in terms of dating and relationships. this means these are typically more likely to be accepting of several types of relationships. if you should be thinking about dating lesbian asian women, you will need to be aware of the difficulties that you may face. first, these women might more selective than guys in terms of dating. this means you need to anticipate to devote many effort should you want to date one of these brilliant women. second, lesbian asian women might almost certainly going to be critical of one’s dating skills than males are. this means you need to anticipate to simply take criticism and learn from your errors. if you’re prepared to put in your time and effort, dating lesbian asian women could be a rewarding experience. this means that you might be prone to find someone who shares your interests and values.
Meet suitable lovers with your top-rated asian lesbian dating sites
Asian lesbian dating sites are a powerful way to find compatible lovers. these sites provide a wide range of features, including the power to search by location, age, and interests. numerous sites also provide boards, in which users can communicate with each other. among the best features of asian lesbian dating sites could be the diversity of this users. they consist of people from all over the world, that makes it easy to find a person who shares your passions. they provide an array of features, and the users are diverse and interesting.