Non classé Recrutement :
Discover the advantages of adult hookup dating sites
buy provigil from india There are benefits to utilizing an adult hookup dating site.first and foremost, these websites provide a safe and anonymous environment for people to connect up.this is a very important function, because it permits individuals to explore their intimate desires without concern with judgment.additionally, these sites offer a variety of different hookup options.this enables individuals find the correct match for them, according to their interests and desires.finally, adult hookup dating websites offer a feeling of community.this is an important function, since it enables individuals connect with other people who share comparable passions.all of the benefits make adult hookup dating internet sites a very important resource for people shopping for a casual relationship.
Find the right site for your needs
Finding the proper site for your requirements can be difficult, however with just a little research, you’ll find a website that’s ideal for you. there are a number of different adult hookup dating sites available, and each you’ve got its very own unique features. some of the most popular adult hookup dating sites consist of hornet, grindr, and adam4adam. each one of these sites features its own unique features that will ensure it is the right option for you. hornet is a website that is designed specifically for singles who’re wanting an informal relationship. it really is a website that is popular among singles that shopping for an enjoyable and easy way to fulfill brand new individuals. grindr is a niche site that is popular among singles who are finding a more severe relationship. it is a site that’s built to assist singles find long-term relationships. adam4adam is a website that’s designed for gay and bisexual singles. all of these sites have features which make them unique. it is critical to choose the site that’s right available, and also the easiest way to do that is to research each one. each site features its own set of features, which is important to discover the people which can be suitable for you. if you should be interested in a site which specifically designed for singles who are finding a casual relationship, hornet could be the perfect option. if you should be interested in a website which more severe and it is made for homosexual and bisexual singles, adam4adam may be the perfect option. each website has its own pair of features making it unique.
Meet regional singles and enjoy a steamy hookup
Dating in the 21st century may be a daunting task. you must find a match that’s appropriate for you, along with doing it quickly. that is where on line dating will come in. there are numerous of various dating internet sites available, and every you have its own set of features and benefits. if you are interested in a hookup, then chances are you should definitely check out an adult hookup dating site. adult hookup dating web sites are perfect for those who are finding an instant and dirty event. they are also ideal for folks who are shopping for a long-term relationship. there are a number of different features available on adult hookup dating websites. you’ll find people from all around the globe, and you may find folks who are searching for a casual relationship or an even more serious relationship. you can find people that are searching for a one-night stand or people who are looking a relationship. adult hookup dating websites are a great way to find a hookup.
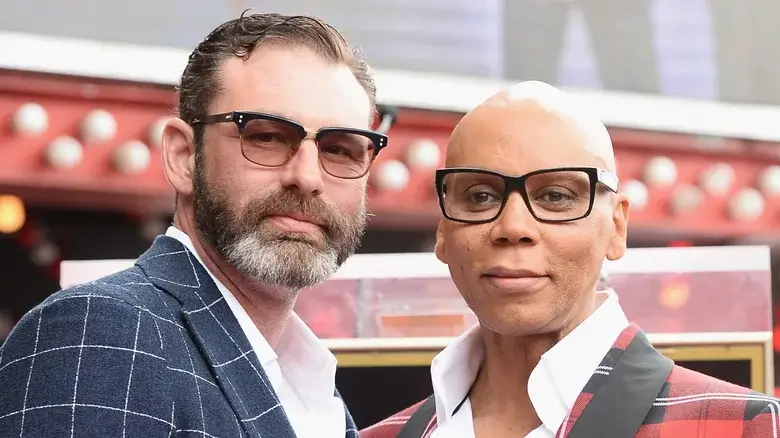
Get ready for a night of enjoyable and excitement
Are you searching for a night of enjoyable and excitement? if so, you need to browse adult hookup dating sites. these sites are perfect for people who wish to have lots of fun. they’re also great for individuals who want to find a brand new partner. there are a lot of great sites on the market. you can find sites which can be focused on several types of tasks. you can find sites that are dedicated to dating or sites which are dedicated to intercourse. additionally some great sites around which can be centered on fun.
Discover the most effective adult hookup dating sites
When it comes down to locating a romantic date, there are a variety of options available. if you’re looking for something casual, you can examine down on line dating sites. if you are in search of one thing more serious, you can look at dating services or groups. but if you’re finding one thing some various, you might like to try adult hookup dating sites. adult hookup dating sites are a great way to find an individual who you could have an informal relationship with. these sites are designed for those who are searching for a short-term relationship. you’ll find people who are thinking about the same things as you, and you can have a great time without worrying all about dedication. there are a number of adult hookup dating sites on the market, and it can be difficult to decide which is the greatest for you personally. however, should you want to find the best website, you need to consider some factors. first, you need to determine what kind of individual you are considering. would you like to find a casual date or an even more severe relationship? next, you need to think about the top features of the site. will be the users verified? could be the site safe? may be the website user friendly? would be the memberships affordable? if you should be shopping for a website which user friendly and has now lots of features, adult hookup dating sites might be your best option for you personally.
find your perfect match with this comprehensive reviews
Looking for a method to have a blast and meet brand new people? look no further than adult hookup dating sites! these sites offer a safe and anonymous environment for people discover partners for casual intercourse or a longer-term relationship. there are a variety of various adult hookup dating sites available, so it’s crucial that you pick the one that most useful suits your preferences. some sites are specifically designed for folks trying to find a one-time hookup, although some are intended for those who are shopping for an even more serious relationship. whatever you’re looking for, be sure to research each site before signing up. each one features its own unique features and benefits that can make it a great choice for you. here are some of the most extremely popular adult hookup dating sites:
1. adult friendfinder is among the oldest and a lot of popular adult hookup dating sites. it gives a wide variety of features, including the search engines that will help you discover specific types of lovers, a note board where you are able to interact with other users, and a dating area where you could browse profiles and send messages. 2. tinder is a mobile app that’s popular because of its quick and easy search functionality. searching for users near you or worldwide, and the app enables you to send and receive communications including make connections along with other users. 3. grindr is a mobile app that is specifically made for individuals selecting sexual partners. 4.
Find your perfect match on official adult hookup dating site
If you are looking for a method to have a blast while making some brand new buddies, then you definitely should truly check out the official adult hookup dating site. it’s a terrific way to satisfy new individuals and now have some fun. plus, it is liberated to make use of, so thereisn’ reason never to try it out. the site is not hard to utilize. you’ll join a totally free account, and you could start searching the pages of this people who are utilizing the site. there are a lot of different people in the site, which means you’re sure to find a person who you’ll like. plus, the site is made to enable you to find somebody who works with you.