Non classé Recrutement :
Find sex with bbw women tonight
Poços de Caldas Bbw women tend to be over looked in terms of finding somebody for sex. it is because a lot of men see them to be fat or perhaps not appealing enough. but there are plenty of bbw women available who are looking for a very good time. if you are enthusiastic about dating one of these women, you need to be prepared to put in some effort. first, you will need to be confident and more comfortable with your self. which means you shouldn’t be bashful about showing your body to a bbw girl. if you are more comfortable with your body, she’s going to become more probably be confident with you. this means you ought to be in a position to provide a great therapeutic massage and perform other sexual tasks correctly. if you are perhaps not confident in your abilities, you won’t be able to attract a bbw girl. which means that home webpage for bbw looking for sex you shouldn’t be too costly or too inexpensive. a bbw girl will never be thinking about a guy who is not prepared to invest in a relationship.
How to locate bbw women looking for sex within area
If you’re looking for only a little excitement in your lifetime, then you should definitely think about looking for bbw women looking for sex. not only will they be some of the most sensual and erotic women nowadays, nonetheless they’re additionally some of the most fun. here are some tips on how to find bbw women looking for sex locally:
1. begin by doing a bit of research. not only will this help you find the best areas to check in, but it also provide some concept of what to anticipate. you will find some informative data on the net, as well as communicate with a few of friends and family whom may understand of good quality places. 2. confer with your neighbors. not just will they be prone to understand a lot in regards to the neighborhood, nevertheless they are often able to aim you in the right direction. all things considered, they are most likely familiar with the sort of folks who are looking for bbw women looking for sex. 3. head out and meet people. this might appear slightly daunting, but it’s really perhaps not that hard. there are a great number of great places to locate bbw women looking for sex, and there isn’t any reasons why you cannot have a lot of fun doing so.
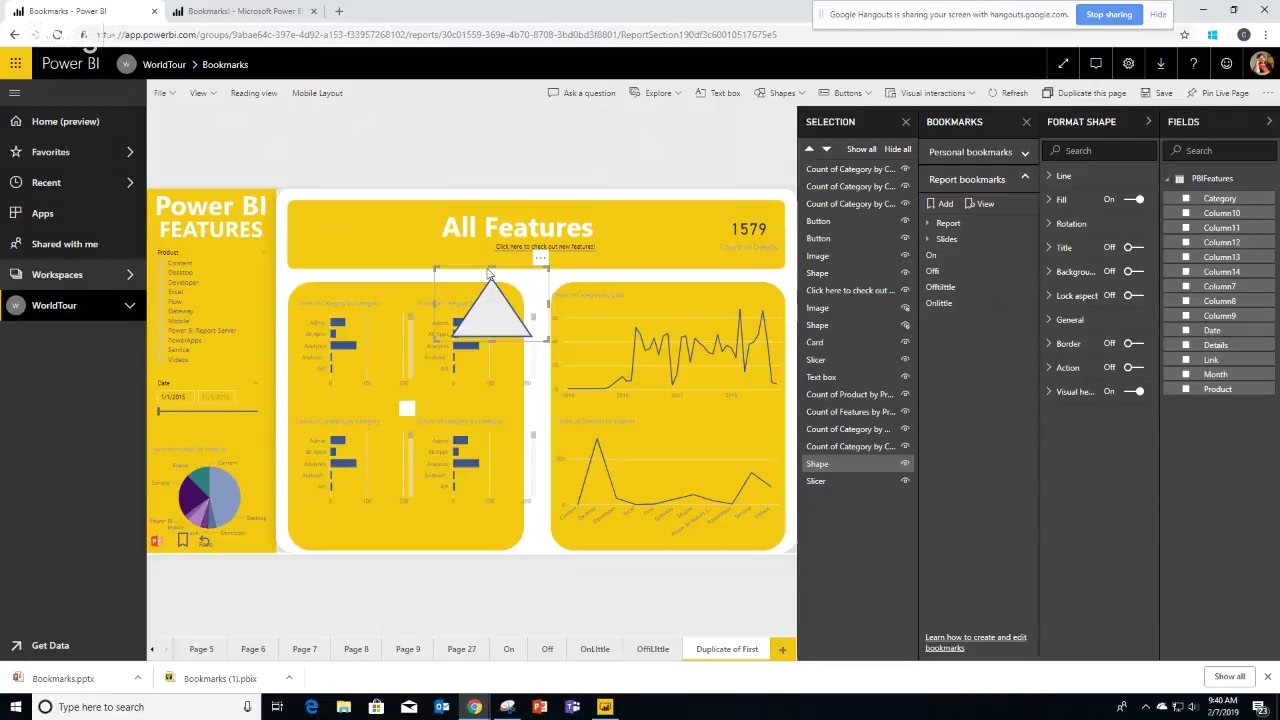
Get started with bbw dating now in order to find your perfect match
If you’re looking for a date which distinctive from standard, you then should think about dating a bbw.not only are these women attractive and sexy, but they also provide a great deal to provide in the way of relationships.if you find attractive dating a bbw, you then should start by doing some research.you will find an abundance of information on the online world about bbw dating, and you need not be bashful about asking concerns.you must also attend bbw dating occasions to meet up with a number of the women in person.if you are looking for a critical relationship, then you should think about dating a bbw.these women are faithful and dedicated, and they are certain to allow you to be delighted.
What makes bbw women special?
there are many items that make bbw women unique.for one, they are generally curvier than average.this means they have more body fat than other women, which could make them more content in their own skin.additionally, bbw women usually have plenty of power and generally are frequently really active.this will make them great lovers in bed, because they are unlikely to have tired quickly.finally, bbw women usually have many love to provide, and generally are often extremely affectionate.this can make them great lovers in a relationship, as they are likely to be faithful.
Why bbw women result in the best sex partners
There are several factors why bbw women make the most readily useful sex partners. first, they’ve a lot of experience with sex. second, they are usually more open-minded than other women. 3rd, they are usually much more comfortable with their bodies. 5th, they are often more physically fit than other women. finally, they are generally more learning and compassionate than many other women. most of these characteristics make bbw women the right partners for anybody looking for the most effective sex feasible. if you are looking for a partner that is skilled and open-minded, bbw women would be the perfect choice. if you are looking for a partner that is more comfortable with their human anatomy and it is capable be flexible and imaginative, bbw women are the perfect option.
Take the first step to satisfaction – join now in order to find bbw women for sex
With so many solutions for dating, it can be difficult to know where you should start.but if you are looking for a more satisfying experience, you should consider joining a dating website created specifically for bbw women.these web sites offer a more diverse range of members, therefore’re prone to find someone who’s thinking about having a sexual relationship with you.if you are hesitant to register for a dating website since you’re worried about being judged, do not be.these web sites are created to help you find love, never to judge you.and whether or not someone does judge you, that is ok.you can invariably ignore them unless you desire to speak to them.but cannot wait any longer.sign up now and begin finding bbw women for sex.you won’t be sorry.